When it comes to PowerShell scripting, consistent naming conventions make the task of building robust solutions much easier. The good news is that there are PowerShell approved verbs you can use when writing scripts and commands.
In this tutorial, you will learn which approved verbs to use in PowerShell through a series of examples.
Jump right in and level up your PowerShell scripting!
Prerequisites
This tutorial comprises hands-on demonstrations. If you wish to follow along, ensure you have a system running PowerShell 5.1 or later. This tutorial uses Windows 11 with PowerShell 7.
Retrieving a List of PowerShell Approved Verbs
In PowerShell, cmdlets are small, single-function commands that perform specific actions in the PowerShell environment.
Each cmdlet in PowerShell has a unique name that follows a verb-noun pair format where:
- The verb part of the name describes the action that the cmdlet performs. Some common verbs used in PowerShell are as follows:
Verb | Function |
---|---|
Get | Retrieves information. |
Set | Changes or updates settings. |
New | Creates something new. |
Remove | Deletes or removes something. |
- The noun part of the name identifies the entity or object on which the action is performed, the target to take action upon. The noun typically describes the category or type of object the cmdlet interacts with, such as command, process, service, or file.
To see all approved verbs you can use for your script:
Execute the following Get-Verb
cmdlet to get a complete list of PowerShell-approved verbs.
Get-Verb
The output below shows just a few verbs you can use for your script.
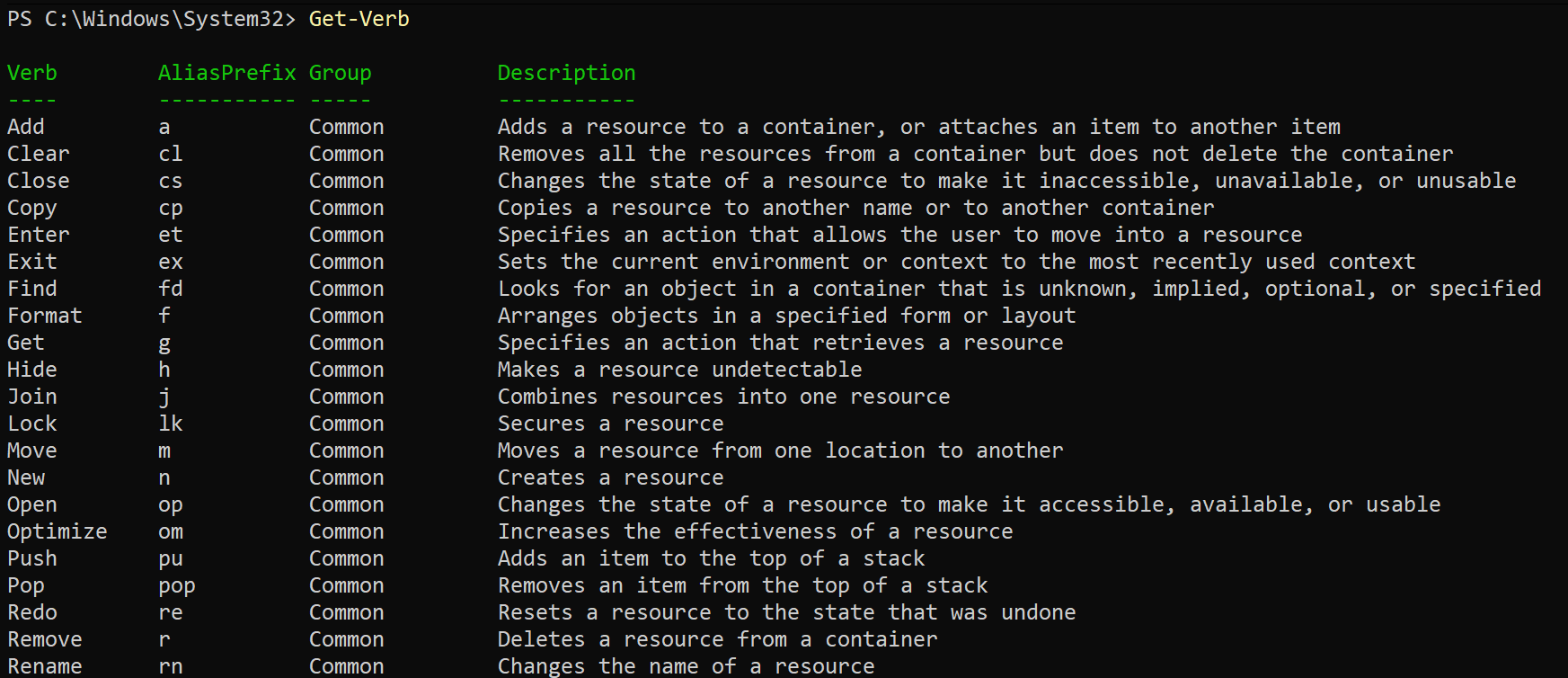
Now, run the same command as below, but this time, you will list all approved verbs that start with re
via a wildcard search. Doing so narrows down the list of verbs to those associated with reset, resize, redo, and so on.
Get-Verb re*
As shown below, narrowing down the list of verbs can be helpful when you have a specific action in mind and wish to find a verb that closely matches that action.
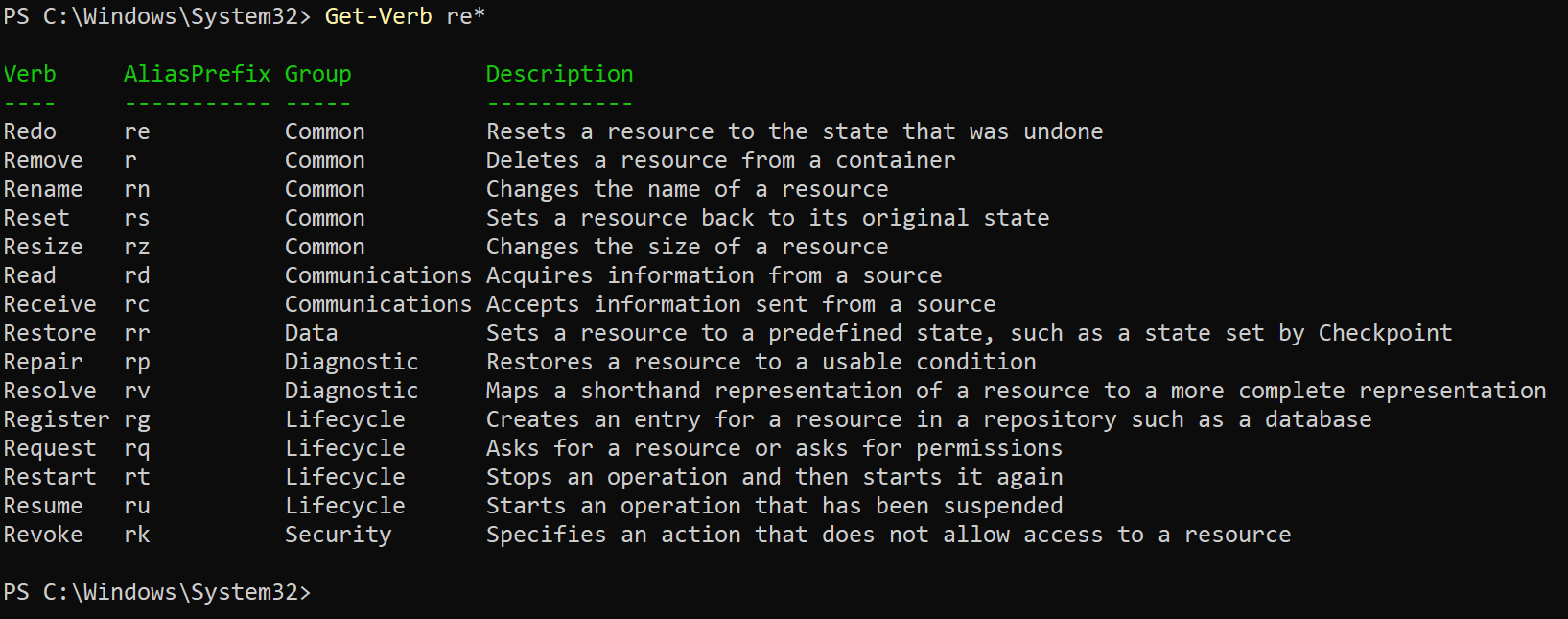
Creating New Resources via the New
Verb
You have just retrieved a list of approved verbs in PowerShell, but how do you use those verbs in your script or when running commands? You will prepend the New
verb to a noun representing the type of resource you wish to create (i.e., user, file, service).
But in this example, you will create a new resource, specifically a new user, as follows:
Execute the following New-LocalUser
command to create a new user called John Smith
with a password (Password123
) and set the account never to expire (-AccountNeverExpires
).
New-LocalUser -Name "John Smith" -AccountNeverExpires -Password (ConvertTo-SecureString -AsPlainText "Password123" -Force

Retrieving Resource Information via the Get
Verb
Besides creating new resources, PowerShell also has a verb that lets you retrieve information about any of your existing resources. In PowerShell, the Get
verb is commonly used to retrieve or fetch information about a particular resource, such as the OS, services, files, and other objects.
To retrieve resource information via the Get
verb:
Run the Get-LocalUser
command below to get information about a specific local user (John Smith
).
Get-LocalUser -Name "John Smith"
Below, the output shows the user account John Smith is Enabled (active).
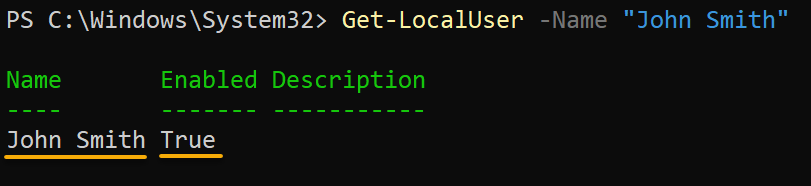
Get
verbModifying Existing Resources via the Set
Verb
Imagine you noticed something was off after seeing one of your resource’s information. How can you modify an existing resource? Worry not! A PowerShell-approved verb, the Set
verb, allows you to make changes to existing resources.
Instead of recreating resources, the Set
verb lets you adjust by modifying their properties or configurations.
To modify existing resources via the Set
verb:
Execute the below commands to set the value of $myVariable
to Hello
and modify the value of $myVariable
to Hello, World!
. These commands do not provide output, but you will verify the changes in the following step.
# Define a variable resource
$myVariable = "Hello"
# Modify the value of an existing variable resource
Set-Variable -Name myVariable -Value "Hello, World!"
Now, run the Get-Variable
command below to retrieve information about your resource ($myVariable
).
Get-Variable -Name myVariable
As shown below, the output shows the variable’s name and value.
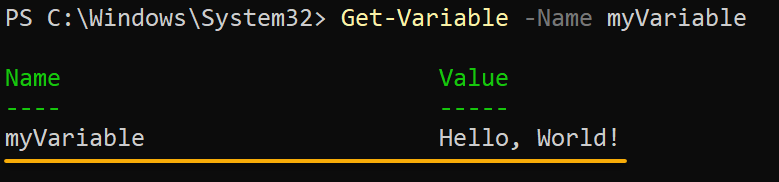
Triggering Actions or Invoking Commands via the Invoke
Verb
Instead of manually running commands, as you did in the previous examples, why not automate them in a PowerShell script? How? The Invoke
verb allows you to initiate actions or invoke commands programmatically.
With a PowerShell script and the Invoke
verb, you can run multiple PowerShell commands at once without typing them all in the console.
To see how triggering actions work via the Invoke
verb:
1. Execute the following Invoke-Expression
command to invoke the Get-Date
command, which lets you retrieve the current date and time.
Invoke-Expression -Command "Get-Date"
The output below shows a successful execution of the Get-Date
command via the Invoke
verb.

Invoke
verb2. Next, create a PowerShell script file (i.e., InvokeVerbDemo.ps1) with your preferred code editor, and populate the following code.
This code displays (Write-Host
) a welcome message and invokes the Get-Service
cmdlet to list all services in your system.
# Display a warm welcome message
Write-Host "Welcome to the Invoke Example!"
# Invoke the Get-Service cmdlet
Invoke-Command -ScriptBlock { Get-Service }
3. Now, run the following commands to invoke (Invoke-Expression
) your PowerShell script (InvokeVerbDemo.ps1
). Replace the $scriptPath
variable’s value with your script file’s path.
$scriptPath = "C:\PowerShellScripts\InvokeVerbDemo.ps1"
Invoke-Expression -Command $scriptPath
The output below shows a welcome message and a list of all services in your system, which confirms a successful execution of your script.
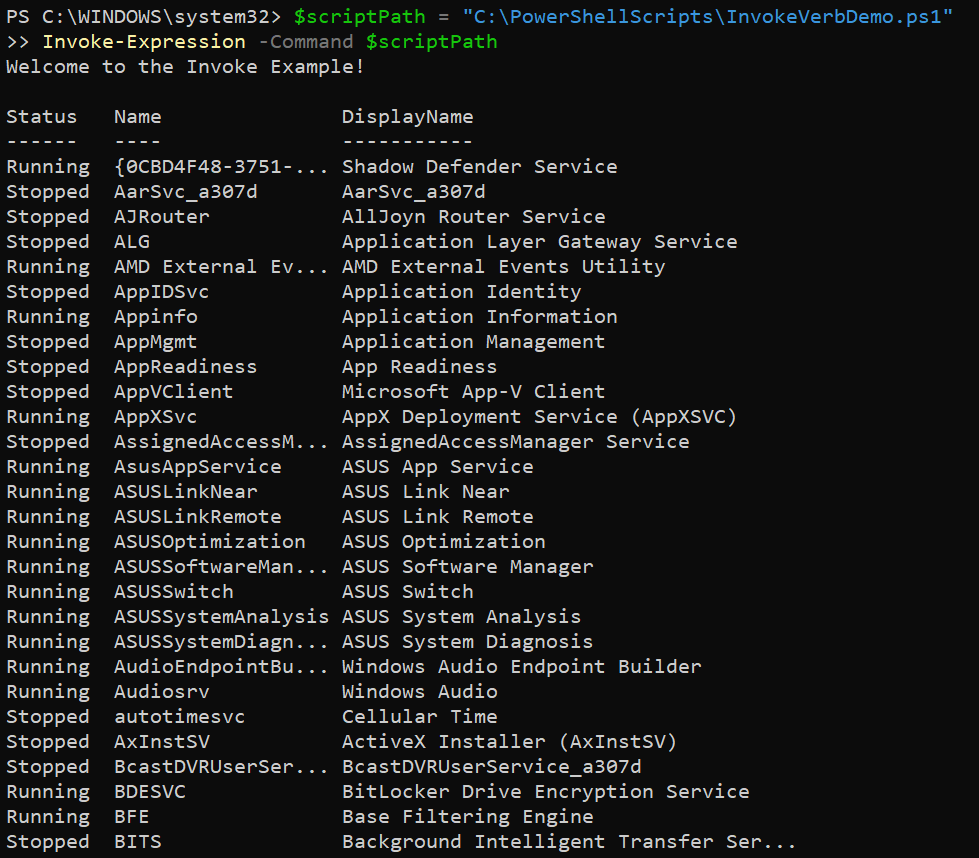
Invoke
verb4. Instead of just commands, write a reusable function (i.e., Invoke-MyTask
) that you can call at any time, as shown below. Essentially, functions enhance the maintainability and reusability of your PowerShell scripts.
In this example, the Invoke-MyTask
function encapsulates commands to perform the following when invoked:
- Displays (
Write-Host
) the stringExecuting MyTask...
on the console. - Retrieves the list of all processes (
Get-Process
) and selects only (Select-Object
) theName
andCPU
properties for each process.
# Define a custom function using the Invoke verb
function Invoke-MyTask {
# Your custom task goes here
Write-Host "Executing MyTask..."
Get-Process | Select-Object Name, CPU
}

5. Lastly, execute the below command to invoke your function (Invoke-MyTask
).
Invoke-Expression -Command Invoke-MyTask
You will see a list of all processes running on your system with their names and CPU usage, as shown below.
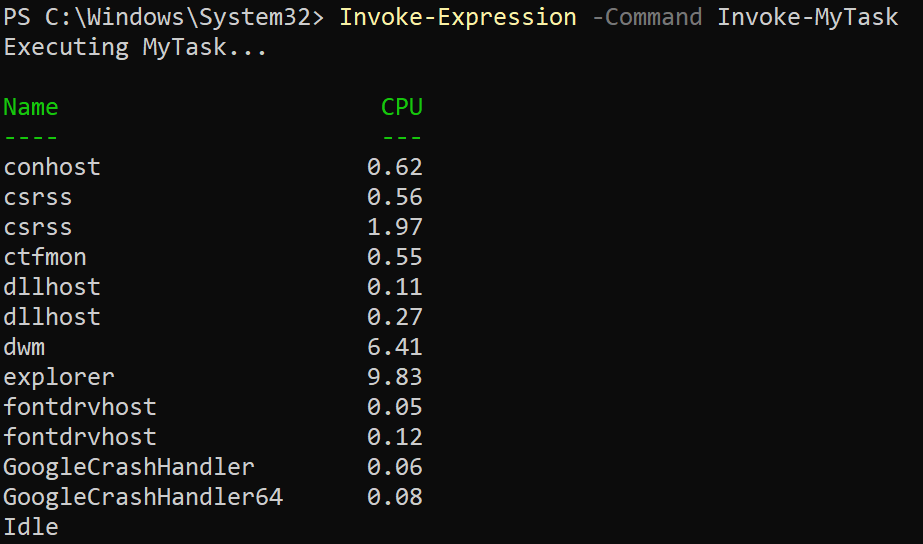
Validating Resources via the Test
Verb
Ensuring the accuracy of input values and conditions is paramount when crafting robust and error-resistant PowerShell scripts. One powerful tool at your disposal for this purpose is the Test
verb.
By employing the Test
verb, you can quickly verify whether a given condition holds a true or false value, enabling you to execute different actions based on the outcome. In this example, you will validate if a file exists via the Test
verb.
Execute the code below to test (Test-Path
) whether a file exists (C:\MyFolder\example.txt
) at the specified $filePath
, and print a message depending on the result.
# Define a file path.
$filePath = "C:\MyFolder\example.txt"
# Test if the file exists, and print a message depending on the result
if (Test-Path $filePath) {
Write-Host "The file exists at $filePath."
}
else {
Write-Host "The file does not exist at $filePath."
}
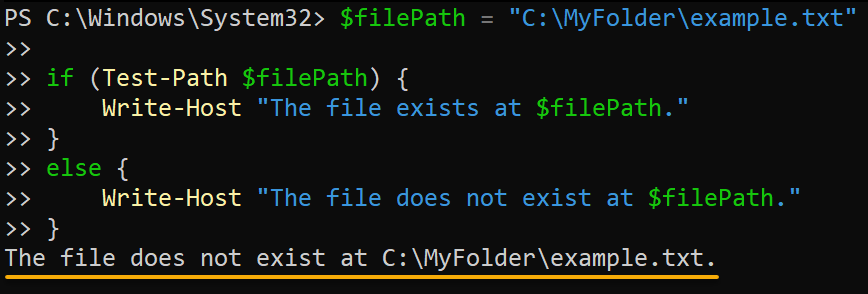
Test
verbConclusion
You now have a basic understanding of PowerShell-approved verbs and how to use them effectively in your scripts and commands. By leveraging these verbs, you can now create robust, efficient, and consistent PowerShell solutions for various tasks and scenarios.
This tutorial is just the beginning of your journey to becoming a proficient PowerShell user. Why not learn other approved verbs, such as data manipulation verbs (i.e., Export
, Import
, Sort
) and lifecycle verbs (i.e., Start
, Stop
)? Take your PowerShell skills to the next level today!