Interested in using the PowerShell Get-Process cmdlet to display the running processes of a system? With Get-Process
you can find the process owner, the process ID, or even where on disk the process is located.
In this article, you will learn how to use PowerShell’s Get-Process
cmdlet through real-world examples. If wrangling processes to bend them to your will on Windows or Linux sounds like fun, then keep reading!
Related: How to Kill a Process in Linux Using ps, pgrep, pkill and more!
Prerequisites
Before going any further, below are the necessary prerequisites to follow along with the examples in this article.
- Although Windows PowerShell 5.1 is sufficient for most examples here, PowerShell 7.1 and greater is necessary for Linux support.
Related: Upgrading to PowerShell 7: A Walkthrough
- This article uses Windows 10 and Ubuntu 20.04 LTS, but any OS that PowerShell runs on will work.
Ready? Let’s dive in and manage some processes!
Displaying Running Processes
Get-Process
manages local processes. In this first example, you are using the PowerShell Get-Process c
mdlet. This command displays all running processes.
Get-Process
returns a point-in-time snapshot of a system’s running process information. To display real-time process information Windows offers Windows Task Manager and Linux offers the top command.
To get started, open up your PowerShell console and run Get-Process
. Notice, that Get-Process
returns the running process information, as shown below. The output format is identical for the Windows and Linux operating systems.
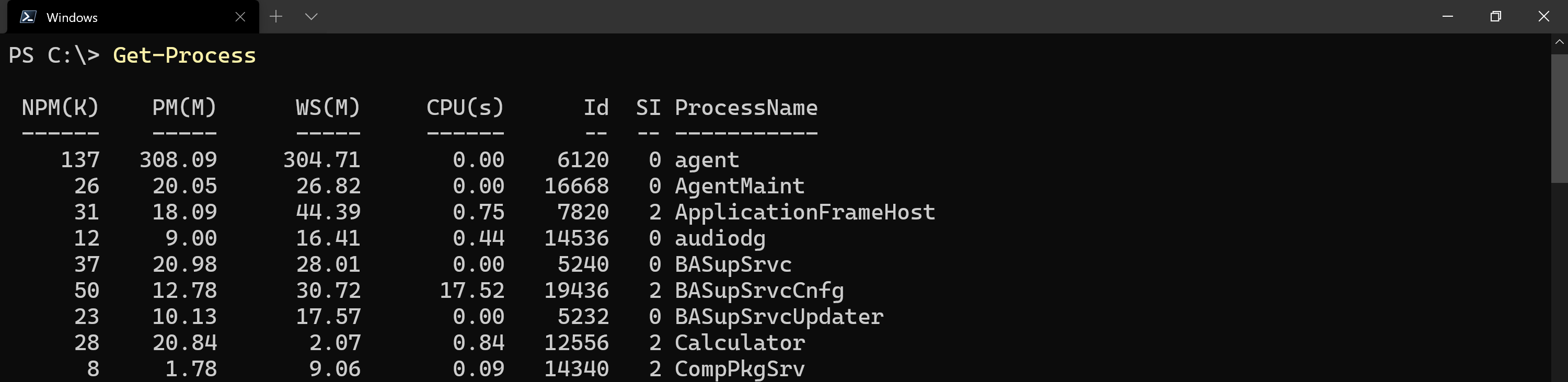
Get-Process
cmdlet on Windows to display local processes.By default, the
gps
orps
exist as command aliases forGet-Process
. As PowerShell 7 is cross-platform, theps
command conflicts with a built-in Linux command. Thereforeps
will not work on Linux, only thegps
alias.
The meaning of Get-Process
output may not be immediately obvious. The default Get-Process
properties are described in more detail below.
- NPM(K) – The amount of non-paged memory a process is using, displayed in kilobytes, as indicated by the
(K)
notation. - PM(M) – The amount of pageable memory a process is using, displayed in megabytes, as indicated by the
(M)
notation. - WS(M) – The size of the working set of the process, displayed in megabytes. The working set consists of the pages of memory that were recently referenced by the process.
- VM(M) – The amount of virtual memory that the process is using, displayed in megabytes. Includes storage in the paging files on disk.
- CPU(S) – The amount of processor time that the process has used on all processes, displayed in seconds.
- Id – The process ID (PID) of the process.
- SI – Session Identifier of the running process. Session
0
indicates the process is available for all users,1
indicates the process exists under the first logged in user, and so on. - ProcessName – The name of the running process.
To display a list of property aliases mapped to full property names, use the command
Get-Process | Get-Member -MemberType 'AliasProperty'
.
Below is another great example. For each instance of the brave process it finds, it uses that process’s ID ($_.id
) and passes it to Get-NetTCPConnection
. PowerShell then uses Get-NetTCPConnection
to find information about each network connection the brave process has open.
Run the following code in your PowerShell session when the Brave browser is running.
Get-Process -Name brave | ForEach-Object { Get-NetTCPConnection -OwningProcess $_.Id -ErrorAction SilentlyContinue }
Thank you to Jay Adams over at SystemFrontier!
Congratulations, you can now view all the running processes on both Windows and Linux using Get-Process
!
Finding Specific Process Attributes
Get-Process
returns many different properties on running processes as you have seen earlier. Like with all other PowerShell objects, you can selectively pick out properties on objects.
Let’s now step through a simple example of how you can retrieve specific properties for a specific process:
- Fire up your Windows calculator.
2. With a PowerShell console open, run Get-Process
using the Name
parameter to only show all running processes with Calculator as the name. You’ll see the same output you’ve seen previously.
Get-Process -Name 'Calculator'
Get-Process
returns many properties as expected. Maybe you only want to find CPU utilization with the value under the CPU(s)
column. Surround the Get-Process
command with parentheses and reference the CPU
property as shown below. You’ll see that it only returns the value for the CPU
property.
(Get-Process -Name 'Calculator').CPU
Notice that
Get-Process
returns a name calledCPU(s)
and the code snippet above used the name of justCPU
. Sometimes PowerShell doesn’t show the real property name in the output. This concept is performed with a PS1XML formatting file.
The CPU time is expressed as a total of seconds across cores. To get that to a more human-readable number, round it to the nearest tenth using a Math
method as shown below.
$cpu = (Get-Process -Name 'Calculator').CPU
[math]::Round($cpu,2)

You can use the above approach to find any other properties too like
Id
if you’d like to only see a process’ ID.
Leave the Calculator application running. You’ll be using this application for the remainder of the examples.
Retrieving Process Memory Usage
Troubleshooting slow running systems can be a challenge, with constrained memory often a cause. Continuing with the Calculator app, retrieve the Calculator
process, and display only the VM
property. As seen below, the memory used is displayed in megabytes (MB).
(Get-Process -Name 'Calculator').VM

Calculator
process memory usage.To aid in understanding the memory usage, utilize the built-in PowerShell conversion multipliers to change megabytes (MB) to gigabytes (GB). In the below example, you will convert the memory used to GB and then use the .NET math library Round
method to round the value, as seen in the below screenshot.
$ProcessMemoryGB = (Get-Process -Name 'Calculator').VM
$ProcessMemoryGB / 1GB
# Use the .NET Math type Round method
[Math]::Round($ProcessMemoryGB / 1GB)
Using built-in PowerShell utilities to convert the values makes it easier to understand the output. Read on to learn how to locate a process’s ID.

Exposing Lesser-Known Properties
Not all properties are included or shown by default with Get-Process
. Read on below to learn more about the Path
and UserName
properties and how to use them!
Discovering Where a Process Binary Lives
There are many places on a system that a process executable can be stored. If a process is currently running, Get-Process
makes finding the process file system path easy, despite Path
not displaying by default. As shown below, the Path
property contains the filesystem location of the process executable.
(Get-Process -Name 'Calculator').Path

Get-Process
to display a process’s full file system path on Windows.Just as in Windows, Get-Process
in Linux also returns the filesystem path. In the example below, the gnome-calculator
process is running with the path displayed in the console output.
(Get-Process -Name 'gnome-calculator').Path

Get-Process
to display a process’s full file system path on Linux.Crafty bad actors may name a process the same or similar as a trusted one. Therefore, the ability to locate the filesystem path aids in a security incident response (IR) scenario. Read on to discover how to locate the process owner, as UserName
is not included in the default output.
Finding the Process Owner
To include the UserName
value in the output, you will need to use the IncludeUserName
parameter. It is important to know the process owner, especially to avoid unwittingly terminating another user’s process. As shown below, the UserName
property is now included in the process output.
Get-Process -Name 'Calculator' -IncludeUserName

Calculator
process on Windows.Finally, read on to learn about using Get-Process
on a remote computer to retrieve process information!
Finding Processes on Remote Computers
Although in Windows PowerShell, Get-Process
doesn’t have any remote capabilities on its own, you can always leverage PowerShell Remoting and the Invoke-Command
to run it on remote computers.
Related: How to Set up PSRemoting with Windows and Linux
But, if you’re on Linux or are running PowerShell 6 on Windows, you now have a ComputerName
parameter you can use to query processes on remote computers.
Get-Process -ComputerName 'remote_computer_name' -ProcessName 'process'
The
-ComputerName
parameter was removed in PowerShell 7.x as the cmdlet is not directly related to remoting. To achieve the same, you can wrap the same in anInvoke-Command
, like so:Invoke-Command -ComputerName "ComputerName" -ScriptBlock { Get-Process -ProcessName 'process' }
When the above command is run against a remote computer, the same output is shown as if the Get-Process
command was run locally.
Below is an example of remoting to another computer and getting running processes:

You can target multiple computers by separating them with a comma e.g.
Get-Process -ComputerName SRV1,SRV2.
Next Steps
In this article, you’ve learned how to use the PowerShell Get-Process
cmdlet to find running processes with PowerShell on local and remote computers both Linux and Windows.
Now, what will you do with this knowledge? Try passing a process retrieved by Get-Process
to Stop-Process
on a local or remote computer to terminate!