Do you spend hours just merging multiple text files? If yes, whether you are a writer, coder, or data analyst, let PowerShell come to the rescue. With PowerShell, appending to files (even read-only files) can be quick.
In this tutorial, you will explore how PowerShell helps you work smarter in text editing, freeing up your time to focus on more critical tasks.
Ready? Say goodbye to tedious manual work and power up your text editing skills!
Prerequisites
This tutorial will be a hands-on demonstration. To follow along, you will need a Windows system with PowerShell installed. This tutorial uses Windows 11 with PowerShell 7.
Working with PowerShell Appending to Files
Updating a text file without realizing you are overwriting its content can be catastrophic. But worry not. With PowerShell, appending to files while keeping the existing content is a quick task with the Add-Content
cmdlet.
Below is the syntax for appending texts to a text file, where -Path
specifies the text file’s path (FILE_PATH
) and -Value
specifies the text to append.
Add-Content -Path "FILE_PATH" -Value "New text to append”
Suppose you have a log file called error.log
that records errors that occur during a script’s execution. You want to append new errors to the end of the file each time the script is run.
To see how the Add-Content
cmdlet works, follow these steps:
1. Run the below Set-Content
command, which does not provide output to the console but creates a sample file called error.log
in the current directory (.
) with some content.
Set-Content -Path "./error.log" -Value "This is a sample error message.”
2. Next, run the code below that tries to divide one by zero (1/0
) and append a message to the end of your error.log
file when an error occurs.
Alternatively, you can create a PowerShell script file, populate the code below to the file, and run it.
The code below does not provide output to the console since the Add-Content
cmdlet appends any error message to the end of the error.log
file.
try {
# Put your code here
$result = 1/0
} catch {
# If an error occurs, set the error message to a string variable
$ErrorMessage = "An error occurred: $_"
# Write the error message to the error.log file
Add-Content -Path "./error.log" -Value $ErrorMessage
}
3. Now, run the following Get-Content
command to verify that the error message was successfully appended to the error.log
file.
Get-Content -Path "./error.log”
Below, the new error message is appended to the end of your error.log file.

Appending Texts via Redirection Characters in PowerShell
In PowerShell, a redirection character is a special symbol used to redirect the output of a command to a file or another command.
The most commonly used redirection characters in PowerShell are as follows:
Redirection Character | Function |
---|---|
> – Greater-than symbol. | Redirects a command’s output to a file, overwriting any existing content. |
>> – Double greater-than symbols. | Redirects a command’s output to a file, appending the output to the end of the file without overwriting any existing content. |
To demonstrate how the double greater-than symbol works in appending text:
1. Run the below Set-Content
command to create a file called running_process.log
in the current directory with some texts.
Set-Content -Path "./running_process.log" -Value "Running processes go here."
2. Next, run the Get-Process
command to get all running processes and append the result (>>
) to the end of the running_process.log
file’s contents.
Since the result is appended to a file, this command does not produce output to the console.
Get-Process >> running_process.log
Get-Process >> running_process.log
3. Lastly, run the cat
command below to verify that the running_process.log
file has been appended with the new running processes.
cat running_process.log
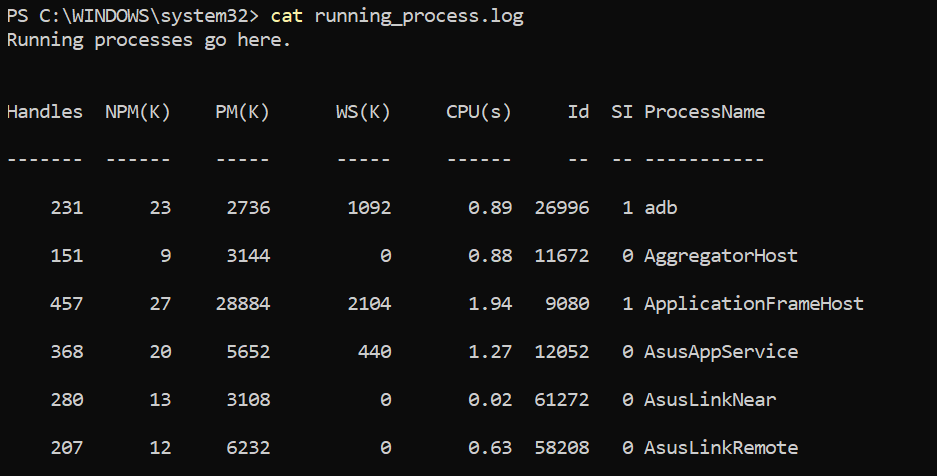
Appending Contents of Existing Files to Another
So far, you have seen how to append texts (command results) to a file. But how about appending the contents of an existing file to another? Appending the content of an existing file to another can be a proper technique when working with text-based files in PowerShell.
Suppose you have multiple log files. You can combine them into a single file to streamline your workflows and manage your files more efficiently.
To append an existing file’s contents to another:
Run the code below to create three log files (Out-File
), each with some contents, and append the contents of all three log files to a single destination log file.
Like the previous examples, the code below does not provide output to the console, but you will verify the appended contents in the following step.
# Define the paths of the source and destination files
$logFile1Path = "C:\logs\log1.txt"
$logFile2Path = "C:\logs\log2.txt"
$logFile3Path = "C:\logs\log3.txt"
$destinationFilePath = "C:\logs\combinedLogs.txt"
# Create three log files with some content
"Log entry 1" | Out-File -FilePath $logFile1Path
"Log entry 2" | Out-File -FilePath $logFile2Path
"Log entry 3" | Out-File -FilePath $logFile3Path
# Get-Content - Read the contents of each source log file.
# Add-Content - Append the contents of the log files to the destination log file.
Add-Content $destinationFilePath (Get-Content $logFile1Path)
Add-Content $destinationFilePath (Get-Content $logFile2Path)
Add-Content $destinationFilePath (Get-Content $logFile3Path)
Now, run the cat
command below to verify that the contents of all the log files have been successfully appended to the destination log file.
cat "C:\logs\combinedLogs.txt"

Appending Contents to Read-Only Files
A read-only file is a file you can view/open but is locked for editing or modification. But in some cases, it may be necessary to append text to a read-only file, like when updating a configuration file. With these restrictions, how can you append contents to read-only files?
To append contents to a read-only file involves three steps; temporarily remove the file’s read-only attribute, perform modifications, and restore the read-only attribute.
Note that the first two codes in this section do not provide output to the console, but you will verify the appended contents later.
1. Run the code below to create (Out-File
) a config file (config.xml
) with some contents, and set the file’s read-only attribute (Set-ItemProperty
) to $True
.
# Define the path and name of the config file
$configFilePath = "C:\config\config.xml"
# Create the config file with some initial content
@"
<config>
<setting1>true</setting1>
<setting2>false</setting2>
</config>
"@ | Out-File -FilePath $configFilePath
# Set the config file as read-only
Set-ItemProperty -Path $configFilePath -Name IsReadOnly -Value $True
2. Now, run the following code to remove the read-only attribute ($False
), append some new content (Add-Content
) to the file, and set the read-only attribute back to $True
.
# Check if the file is read-only
$isReadOnly = (Get-ItemProperty -Path $configFilePath -Name IsReadOnly).IsReadOnly
if ($isReadOnly) {
# Remove the read-only attribute from the file
Set-ItemProperty -Path $configFilePath -Name IsReadOnly -Value $False
# Append some new content to the file
Add-Content -Path $configFilePath -Value "<setting3>New Setting</setting3>"
# Restore the read-only attribute on the file
Set-ItemProperty -Path $configFilePath -Name IsReadOnly -Value $True
}
else {
Write-Host "The file is not read-only."
}
3. Finally, run the below cat
command to verify that the new setting has been appended to the config.xml
file successfully.
cat "C:\config\config.xml"
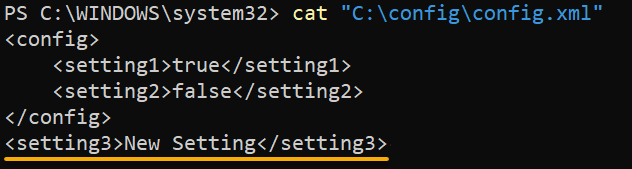
Conclusion
PowerShell provides a powerful way to append text to files using different cmdlets and redirection characters. And in this tutorial, you have seen PowerShell appending texts to a file, combining multiple contents into one, and even modifying read-only files.
With some practice and creativity, you can use PowerShell to streamline your workflows and work more efficiently with text-based files.
Now, why not explore a real PowerShell text editor to improve productivity and make your work more enjoyable?