With automation, reading data from a text file is a common scenario. Most programming languages have at least one way of reading text files, and PowerShell is no exception. The PowerShell Get-Content
cmdlet, a PowerShell tail equivalent, reads a text file’s contents and imports the data into a PowerShell session.
The PowerShell Get-Content
cmdlet is an indispensable tool when you need to use text files as input for your script. Perhaps your PowerShell script needs to read a computer list to monitor or import an email template to send to your users. PowerShell Get-Content
easily supports these scenarios!
How about following a log file in real-time? Yes, the PowerShell Get-Content
can do that, too!. Continue reading this article, and you will learn how to use the Get-Content
cmdlet to read text files in PowerShell.
Prerequisites
If you’re interested in following the examples in this tutorial, you will need the following requirements.
- You’ll need a computer that is running on Windows 10. This tutorial uses Windows 10 version 20H2. But don’t worry, you’ll be okay with the Windows 10 version that you have.
- You should have at least Windows PowerShell 5.1, or PowerShell 7.1 installed on your computer. Here PowerShell 7.1 is used, but either will version will work!
- You’ll be writing and testing commands, so you’ll need a code editor. The recommended editors are Windows PowerShell ISE, built-in to Windows, and Visual Studio Code (VSCode). This article uses VSCode.
- It will also help if you create a working directory on your computer. The working folder can be anywhere you want. However, you’ll notice that the examples in this tutorial reside in the C:\demo folder.
- To get started, you need some content! Create a file, in your working directory, with the name fruits.txt that includes ten different fruits for simplicity. You will be using this text file in all the examples.
cherry
berry
apricot
papaya
raspberry
melon
peach
tangerine
cantaloupe
orange
Don’t know which PowerShell version you have? Visit the article How to Check your PowerShell Version (All the Ways!).
Reading a Text File and Returning the Result as a String Array
The Get-Content
cmdlet reads content from a file, and by default, returns each line of a text file as a string object. As a result, the collection of PowerShell objects becomes an array of string objects.
The below code reads the contents of the fruits.txt file and displays the result on the PowerShell console as seen in the below screenshot.
Get-Content .\fruits.txt
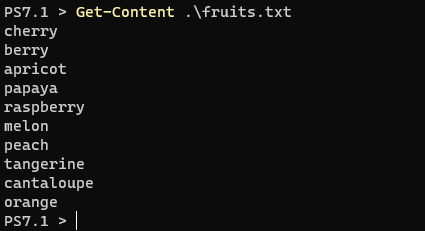
Get-Content
.Get-Content
reads and stores the content as an array, but how do you know that for sure? First, save the content to a PowerShell object which you can then examine to determine the type.
Save the content into to a object
$fruits = Get-Content .\fruits.txt
Display the type of the object
$fruits.GetType()
Retrieve the count of items within the object
$fruits.Count
Output the contents of the object to the console
$fruits
Looking at the screenshot below, the $fruits
variable is an array that contains ten objects. Each object represents a single line of text.
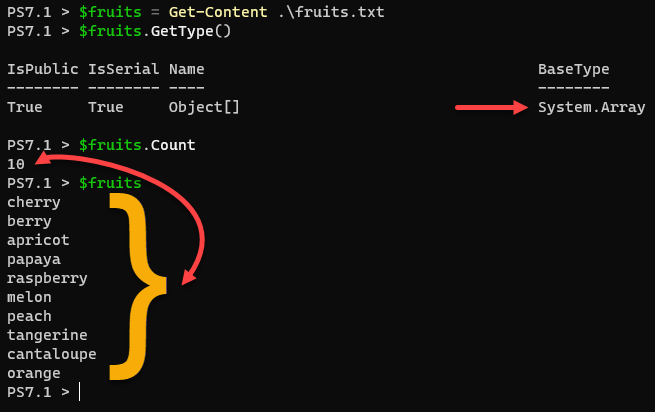
Returning a Specific Line From a Text File
In the previous example, you’ve learned that the default Get-Content
result is an array or a collection of objects. Each item in a collection corresponds to an index number, and PowerShell indexes typically start at zero.
The screenshot below shows that there are ten items in the string array. The array indexed the ten items from zero to nine.
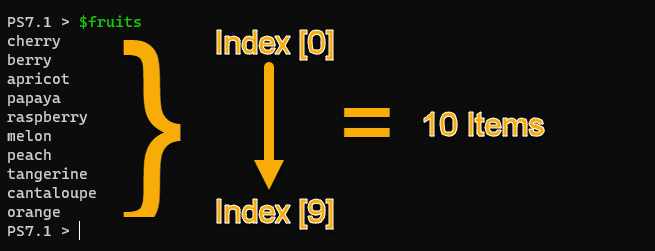
To only display the fifth line of content, you’ll need to specify the index number 4
, enclosed in square brackets (known as array notation).
(Get-Content .\fruits.txt)[4]
You may notice that the
Get-Content
command is enclosed in a parenthesis. This notation tells PowerShell to run the command enclosed in the parenthesis first before other operations.
In the screenshot below, you’ll see that the only returned result is raspberry
, which is the item at index 4
and corresponds to the fifth line in the text file.

Get-Content
results.What if you need to get the content in the last line? Thankfully, you do not need to know the total number of lines. Instead, use [-1]
as the index, and Get-Content
will display only the last line of the file.
(Get-Content .\fruits.txt)[-1]
Limiting the Number of Top Results Returned by Get-Content
Use the TotalCount
parameter of Get-Content
to retrieve a specified number of lines from a text file. The TotalCount
parameter accepts a long value which means a maximum value of 9,223,372,036,854,775,807.
For example, the command below reads the content and limits the result to three items.
Get-Content .\fruits.txt -TotalCount 3
As you would expect, the result below displays only the top three lines from the beginning of the text file.
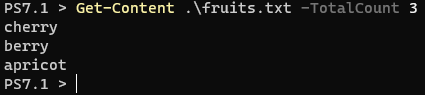
Get-Content
command and the TotalCount
parameter.Use the PowerShell Tail Parameter to Return Results From the End of a File
In the previous example, you used the PowerShell Get-Content
cmdlet to read a text file and limit the top results. It is also possible to achieve the opposite with PowerShell Get-Content
. Use the PowerShell Tail
parameter to read a specified number of lines from the end of a file.
The example code below reads the text file and displays the content of the bottom four lines.
Get-Content .\fruits.txt -Tail 4
After running the PowerShell tail
command, the expected outcome will be limited to the last four lines of content, as shown in the image below.
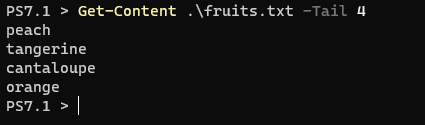
Get-Content
Tail
parameter.The Tail
parameter is often used together with the Wait
parameter. Using the Wait
parameter keeps the file open and checks for new content once every second. The demonstration below shows the Tail
and Wait
parameters in action. To exit Wait
, use the key combination of CTRL+C
.
Get-Content -Path .\fruits.txt -Tail 1 -Wait
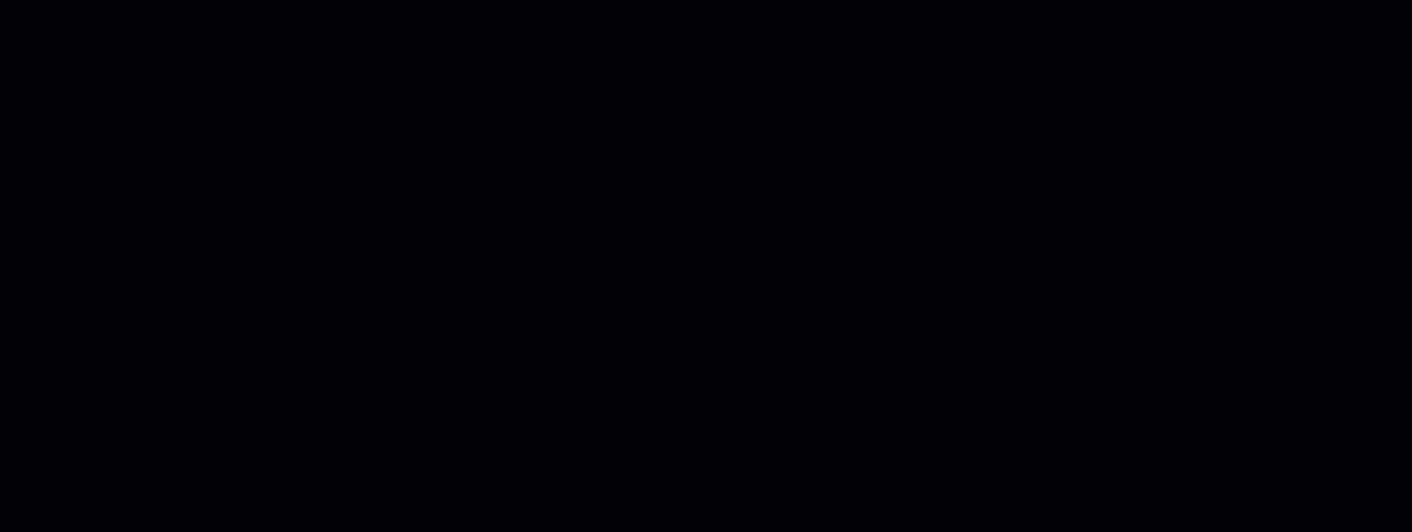
wait
and Tail
parameters with Get-Content
.Returning the Results as a Single String
You may have noticed in previous examples that you’ve been dealing with string arrays as the PowerShell Get-Content
output. And as you’ve learned so far, the nature of arrays allows you to operate on the content one item at a time.
Arrays often work great but can make replacing strings more difficult. The Raw
parameter of Get-Content
reads a file’s entire content into a single string object. Although the code below is the same as used within the first example, the Raw
parameter stores the file content as a single string.
Save the content into to a object
$fruits = Get-Content .\fruits.txt -Raw
Display the type of the object
$fruits.GetType()
Retrieve the count of items within the object
$fruits.Count
Output the contents of the object to the console
$fruits
The screenshot below demonstrates that adding the Raw
parameter to Get-Content
results in treating the content as a single string and not an array of objects.
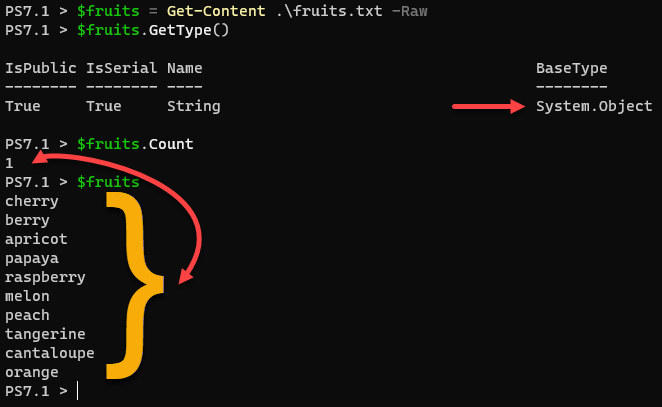
Raw
parameter of Get-Content
reads the file content as a single string object.Once you have the contents of a file in a single string using the Raw
parameter, what can you do with it? Perhaps you need to find and replace a string inside of that file’s content. In the example below, Get-Content
reads the content of a file as a single string. Then, using the replace
operator, replace a specific word with another.
Related: Finding and Replacing Strings
# Get the raw content of the text file
$fruits = Get-Content .\fruits.txt -Raw
# Display the content
$fruits
# Find and replace the word 'apricot' with 'mango'
$fruits -replace 'apricot','mango'
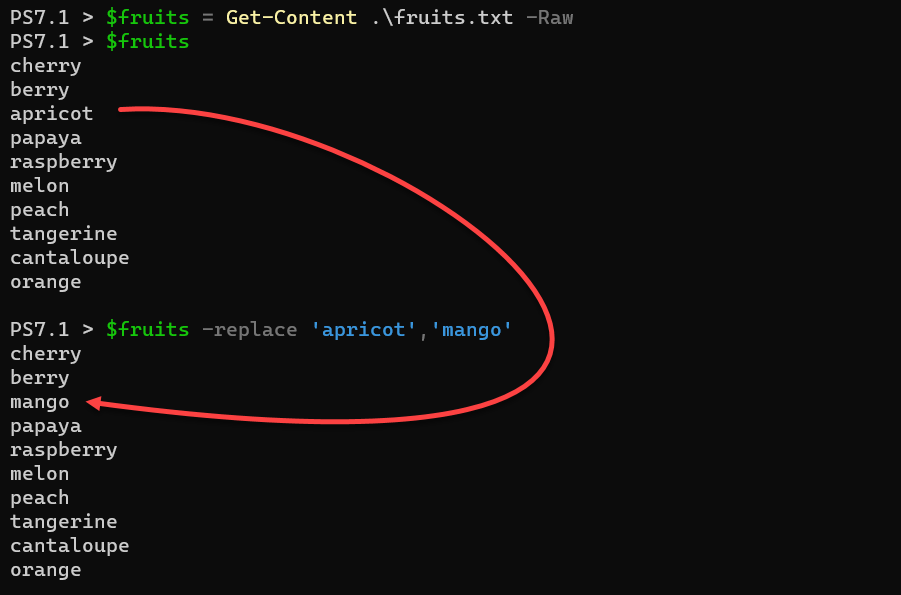
replace
operator.Read Content Only from Files that Matched a Filter
Do you have a folder full of files but need to read the content of a select few? With PowerShell Get-Content
, you do not have to filter the files separately before reading the files’ contents. The Filter
parameter of Get-Content
limits which files the cmdlet reads.
To demonstrate reading the content of only select files, first, create a couple of files to read. As shown below, create the files in your working folder using Add-Content
.
# Add-Content creates the log1.log and log2.log file if they don't exist already and adds the given value
Add-Content -Value "This is the content in Log1.log" -Path C:\demo\Log1.log
Add-Content -Value "This is the content in Log2.log" -Path C:\demo\Log2.log
# Verify that the files have been created
Get-ChildItem C:\demo
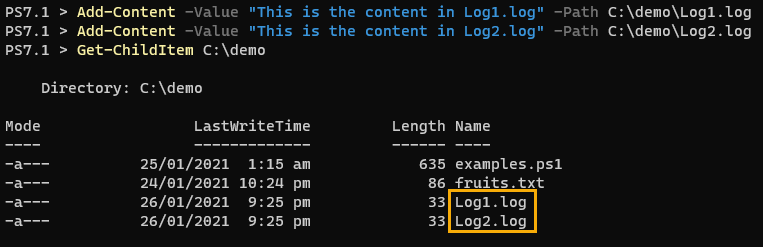
.log
files using Add-Content
.With your test files created, use the Filter
and Path
parameters to only read .log
files in the root directory. The asterisk used in the filter definition indicates to Get-Content
to read any file ending with .log
. The ending asterisk of the path parameter limits the reading of files to only the root directory.
Get-Content -Path C:\demo* -Filter *.log
As shown in the below output, only the content from the .log
files is displayed.

Filter
parameter with PowerShell Get-Content
to limit the read files.Related: Get-ChildItem: Listing Files, Registry, Certificates, and More as One
Reading the Alternate Data Stream of a File
Until now, you have been working exclusively with text files, but Get-Content
can read data from the alternate data stream (ADS) of a file. Feel free to read more about streams, but you can think of a stream as another data attribute stored alongside the typical file contents.
Alternate data streams are a feature of the Windows NTFS file system, therefore this does not apply to
Get-Content
when used with non-Windows operating systems.
You can see alternate data streams by running Get-Item
with the Stream
parameter. When referencing a file using the Stream
parameter, Get-Item
returns a property called Stream
as shown below. This default file content stream is represented with :$DATA
.
To demonstrate the default :$DATA
stream, use the Get-Item
cmdlet to display all available streams in the file fruits.txt. As shown below, Get-Item
displays a single stream.
Get-Item -Path .\fruits.txt -Stream *
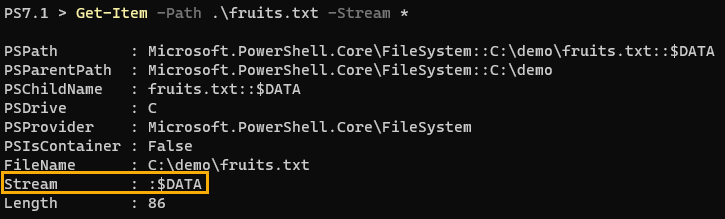
Get-Item
.The Stream
parameter of Get-Content
explicitly reads the content of the default :$DATA
stream as shown below. The returned content is the same as the default Get-Content
output as the :$DATA
stream is read by default.
Get-Content -Path .\fruits.txt -Stream ':$DATA'
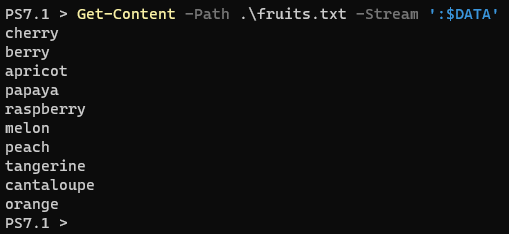
:$DATA
stream using Get-Content
.To demonstrate retrieving an alternate data stream using Get-Content
, modify a file using Add-Content
to add the new stream. Use Get-Item
to show the new stream alongside the default :$DATA
stream, as seen in the below example.
# Add a new ADS named Secret to the fruits.txt file
Add-Content -Path .\fruits.txt -Stream Secret -Value 'This is a secret. No one should find this.'
Get-Item -Path .\fruits.txt -Stream *
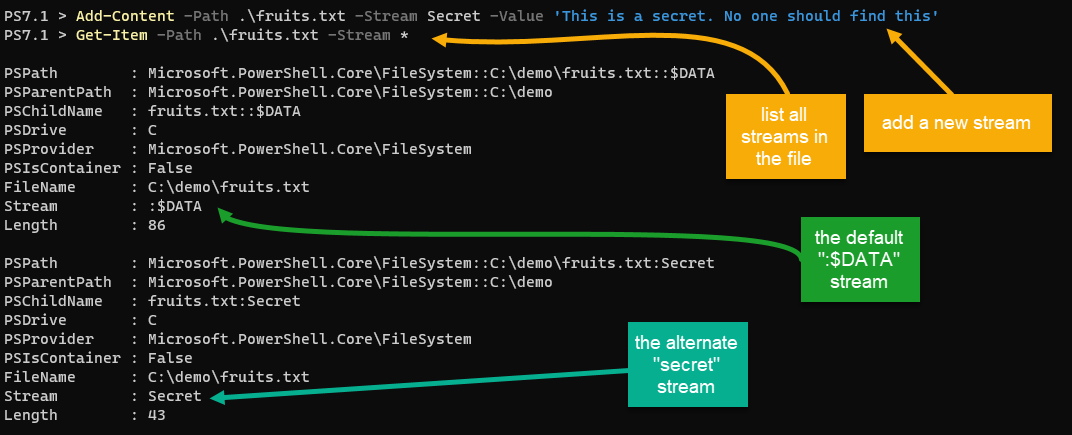
As only the :$DATA
stream is read by default, use the Stream
parameter of Get-Content
to retrieve the new Secret
stream content. As shown below, the Secret
stream content is displayed instead of the default file content.
Get-Content -Path .\fruits.txt -Stream secret

Get-Content
to read the Secret
alternate data stream content.Next Steps With PowerShell Get-Content
In this article, you’ve learned many ways to use Get-Content
to read and manipulate content. You’ve even learned that Get-Content
is flexible enough to read content from alternate data streams!
With what you’ve learned in this article, what other ways can you use Get-Content
in your work? Perhaps you can use Get-Content
to determine if a backup file is outdated and trigger an automatic call to run a backup job?