Probably one of the biggest call contributors to your service desk is Active Directory (AD) user password reset. Building an Active Directory password reset tool would help your service desk and admins handle these requests more efficiently.
Luckily, some PowerShell cmdlets allow you to manage aspects of Active Directory, including resetting passwords. In this article, you’ll learn how to use PowerShell to build an Active Directory password reset tool.
This post is kindly sponsored by Specops Software. Be sure to check out their handy password reset tool for a lot more features that you can build in a simple PowerShell script!
Prerequisites
No tool building can begin without meeting needed requirements first. If you plan to follow this article, you need the following:
- A Windows computer where you’ll write and run your code joined to an Active Directory domain.
- The ActiveDirectory module must be installed on the computer.
- The computer must also have Windows PowerShell 5.1.
- A script editor such as Visual Studio Code or Windows PowerShell ISE. This article uses Windows PowerShell ISE.
Finding the User in Active Directory
Before you can reset a user password, you must find the account first. Your first step should be checking if an account exists. To do that, use the Get-ADUser
cmdlet providing the name of the account using the Identity
parameter.
The
Identity
parameter accepts one of the four accepted identifiers; distinguished name, GUID (objectGUID), A security identifier (objectSid), or SAM account name (sAMAccountName).
For example, if you received a request to reset the password for user_a, you should confirm if the user exists before you issue a reset of the account. To do that, copy the command below and run it in your PowerShell console. Make sure to change the user name to the correct one first.
Get-ADUser -Identity user_a
As you see in the screenshot below, the command returned the user properties, confirming that the account exists and the username is correct.
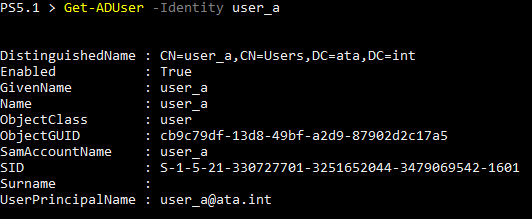
Resetting the Active Directory User Password
Now that you’ve learned how to verify a user account with Get-ADUser
, you know that the result is unique and that the user account exists. You now need to reset the user’s password with the Set-ADAccountPassword
cmdlet.
The
Set-ADAccountPassword
cmdlet changes a user’s password if you also provide the old password. But if you do not have the old password, the cmdlet resets a password instead by using theReset
parameter.
The code below will reset the user_a’s password. The new password will be a random password that is 12-characters long with five non-AlphaNumeric characters. Refer to the code comments for explanations of each line.
Open a PowerShell window, copy the code, paste it in PowerShell, and press Enter to execute.
# Specify here the username whose password to reset.
$username = 'user_a'
# Import the System.Web .NET assembly
Add-Type -AssemblyName 'System.Web'
# Generate a random password that is 12-characters long with five non-AlphaNumeric characters.
$randomPassword = [System.Web.Security.Membership]::GeneratePassword(12, 5)
# Convert the plain text password to a secure string.
$newPassword = $randomPassword | ConvertTo-SecureString -AsPlainText -Force
# Reset the user password
Set-ADAccountPassword -Identity $username -NewPassword $newPassword -Reset
# Display the new password
$randomPassword
The screenshot below shows you the expected result after running the code.
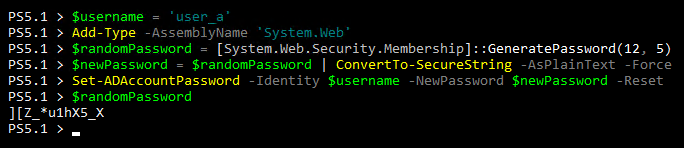
Writing the Active Directory Password Reset Tool
Now you have a working script to reset a user’s password. Next is to turn your script into a tool. A tool is reusable and executes the same set of actions with minimum manual user actions.
When building a tool, one important aspect to consider is that whoever would run the tool should not have to edit the code every time. So far, your code still requires the user to edit the $username
value.
Instead of letting your tool users change the values manually, why not write a script that accepts parameters instead? To do so, follow these steps:
- Open your chosen script editor on your computer. This tutorial is using Windows PowerShell ISE.
- Create a new file and save the file under the name Reset-ADUserPassword.ps1. Save the script in your preferred location. This tutorial saves the file in the C:\scripts folder.
- Copy the code below, paste it into your script editor, and save the script. Refer to the code comments for explanations of each line.
# Add a parameter called username.
param (
$username
)
# If the user did not provide a username value, show a message and exit the script.
if (-not($username)) {
Write-Host "You did not enter a username. Exiting script"
return $null
}
# Check if the user exists or if the username is valid. Do not show the result on the screen.
try {
$null = Get-ADUser -Identity $username -ErrorAction Stop
}
# If the username cannot be found, show a message and exit the script.
catch {
Write-Host $_.Exception.Message
return $null
}
# Generate a random password that is 12-characters long with five non-AlphaNumeric characters.
$randomPassword = [System.Web.Security.Membership]::GeneratePassword(12, 5)
# Convert the plain text password to a secure string.
$newPassword = $randomPassword | ConvertTo-SecureString -AsPlainText -Force
# Try to reset the user's password
try {
# Reset the user's password
Set-ADAccountPassword -Identity $username -NewPassword $newPassword -Reset -ErrorAction Stop
# Force the user to change password during the next log in
Set-ADuser -Identity $username -ChangePasswordAtLogon $true
# If the password reset was successfull, return the username and new password.
[pscustomobject]@{
Username = $username
NewPassword = $randomPassword
}
}
# If the password reset failed, show a message and exit the script.
catch {
Write-Host "There was an error performing the password reset. Please consult the error below."
Write-host $_.Exception.Message
return $null
}
Running the Active Directory Password Reset Tool (Examples)
Now that you have an Active Directory password reset tool, how do you test that the tool works? Run it in PowerShell.
If you’re using Windows PowerShell ISE, there already is a PowerShell console pane available. If not, then you should open a PowerShell session separately. Either way, make sure to change the current working directory to the same folder where you saved the script, such as Set-Location C:\scripts
.
Resetting the Password of One User
Suppose you need to reset one user’s password. Run the Active Directory password reset tool and specify which username to target. To reset a single user’s password, run the script as shown below.
.\Reset-ADUserPassword.ps1 -username user_a
As a result, the script resets and displays the new password on the screen. This output is useful for copying the new password to give to the affected user.
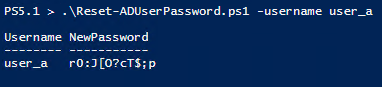
Resetting the Password of Multiple Users
What if you need to reset passwords for many users? Does it mean having to run the Active Directory password reset tool multiple times, too? Technically, yes, but not manually.
Fortunately, in PowerShell, you can loop through a list using arrays and the foreach loop. You can process multiple items in PowerShell with the ForEach-Object
cmdlet. This cmdlet allows you to pass multiple items over the pipeline.
To reset the password of multiple users, you need to create an array containing two or more usernames. Next, pass the array items over the pipeline where the ForEach-Object
cmdlet runs the password reset tool on each username.
@('user_a','user_b') | ForEach-Object {.\Reset-ADUserPassword.ps1 -username $PSItem}
Below you can see your AD password reset tool reset two user passwords.

The same concept applies if you’re reading a list of usernames from a text file. Perhaps you have a text file named users.txt that contains the usernames, like the one shown below.
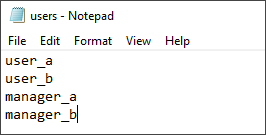
You need to read that text file of user accounts and run your AD password reset tool on each one. To do that, use the Get-Content
cmdlet to import the text file’s content into PowerShell. Next, pass the content to the pipeline and run the Active Directory password reset tool on each username.
Get-Content .\users.txt | ForEach-Object {.\Reset-ADUserPassword.ps1 -username $PSItem}
After running the command, you’ll have performed a reset of multiple user passwords in one execution.

Exploring Specops uReset
Building your own Active Directory password reset tool certainly has its benefits. You’ll give your first-line agents a tool that does a specific job that they can use for one user at a time or in bulk.
However, having such a tool does not help reduce calls for user password resets. Instead of your service desk handling more complicated cases, they may end up drowning in password reset requests. For this reason, you may want to look into third-party tools that offer self-service password reset.
One such excellent tool is called Specops uReset. Specops uReset offers users the option to unlock, change, and reset their passwords. With proper user education, this feature would significantly decrease password request calls through the service desk.
Additionally, you can integrate Specops uReset with identity providers or existing multi-factor authentication (MFA) solutions. With this feature, users can complete password-related tasks securely.
Specops uReset enables the service desk to verify the user’s account through their chosen identity provider. Or by sending a message to the user’s registered mobile number. This Specops uReset feature can significantly improve protection against impersonation and social engineering attacks.
In terms of data security, Specops uReset does not store passwords and other related data anywhere. All password-related data remains in your organization’s Active Directory.
Choosing an Active Directory Password Reset Tool
Specops uReset clearly offers more features relating to the password reset process over a tool created in PowerShell. But is it appropriate to compare a full-featured third-party tool like Specops uReset with a PowerShell tool that does one specific job?
What do you think? As for myself, it all boils down to weighing the need (for the features) and capacity (to buy). But ultimately, the decision depends on you or your organization’s assessment of whether or not your choice will bring value.