When you need to troubleshoot or take a quick peek into a Docker container, SSH is a great option. SSH allows you to quickly connect to a running container and see what’s going on. But connecting to a Docker container first involves some setup, and you have a few different options.
In this tutorial, you will learn how to SSH into Docker containers using the docker run
command and a Dockerfile.
Let’s start!
Prerequisites
If you’d like to follow along step-by-step, ensure you have the following:
- A Linux host. This tutorial uses Ubuntu 18.04.5 LTS.
- Docker installed on the Linux host. This tutorial uses Docker v19.03.8.
Starting a Container and SSH into Docker Containers with docker run
The docker run
command is a Docker command that runs a command when a new container first comes up. Using docker run
, you can launch an interactive SSH session to a container using the steps below.
Before you start this section, be sure you have a Docker image downloaded and available. This tutorial uses the latest Ubuntu Docker image available on Docker Hub.
To SSH into Docker containers with docker run
:
1. Open a terminal on your local machine.
2. Run the docker run
command providing:
- The
name
of the container to run (ubuntu_container_ssh
) - The
i
flag indicating you’d like to open an interactive SSH session to the container. Thei
flag does not close the SSH session even if the container is not attached. - The
t
flag allocates a pseudo-TTY which much be used to run commands interactively. - The base image to create the container from (
ubuntu
).
# Creating the container named ubuntu_container_ssh and start a Bash session.
sudo docker run --name ubuntu_container_ssh -i -t ubuntu

At this point, you’re SSHed to the container and can run any commands you’d like.
3. Next, run any command, such as the touch
command. Touch command will create a new folder named myfolder
in the tmp directory, as shown below.
touch /tmp/myfolder

You can now run any commands you’d like!
Finally, when you’re done running commands, type exit
to close the session.
SSH into Running Docker Containers with docker exec
In the previous section, you learned how to run SSH commands when starting a new Docker container. But what if you need to SSH into Docker containers that are already running? You run the docker exec
command.
The docker exec
command creates a Bash shell inside a running container and is a great way to send SSH commands into a container.
Before you start this section, be sure you have a Docker image downloaded and available. This tutorial uses the latest NGINX Docker image available on Docker Hub.
To SSH into a running Docker container with docker exec
:
1. Open a terminal on your local machine.
2. Next, run the docker run
command to start the container. Be sure to specify the -d
flag to run the container in the background to keep it alive until you remove it. The command below starts a container called nginx-testing
.
sudo docker run --name nginx-testing -d nginx

3. Now, run the docker ps command to verify the container is running. The docker ps
command will list all the running containers running on the Docker host.

4. Finally, run docker exec
, as shown below, to SSH into the running container called nginx-testing
. In the below code snippet:
docker exec
command runs (/bin/bash
) to get a Bash shell in the container.-it
flag allows you to run a container in interactive mode, that is, you can execute commands inside the container while it is still running.nginx-testing
is the name of the container.
sudo docker exec -it nginx-testing /bin/bash

Setting up an OpenSSH Server and Connecting with a Dockerfile
Until now, the tutorial has assumed you’re connecting to a container that already has some SSH server installed. But what if it doesn’t? Perhaps the image you’re using doesn’t already have OpenSSH installed, and you need to configure it first?
Using a Dockerfile, you can configure all of the tasks necessary to not only SSH into Docker containers but set up an OpenSSH server from scratch too.
Assuming you still have your local terminal open:
1. Optionally create a directory to store the Dockerfile. This tutorial will use the ~/DockerFileContainerTest directory.
2. Open your favorite text editor, copy/paste the below Dockerfile inside and save the file as Dockerfile inside the ~/DockerFileContainerTest directory. This Dockerfile contains all the commands and configurations to build a new Docker image on top of any base image and set up OpenSSH.
The DockerFile below contains various steps/instructions that will build the container:
FROM
– Defines theubuntu:16.04
base image to use.RUN
– Executes commands in a new layer on the top of the base image.CMD
– CMD allows you to run the commands. There are two ways in which commands are executed either via exec or using shell formats.
EXPOSE
– Informs Docker that the container listens on the specified network ports at runtime. The container will be exposed on pot22
.
# Instruction for Dockerfile to create a new image on top of the base image (ubuntu)
FROM ubuntu:16.04
RUN apt-get update && apt-get install -y openssh-server
RUN mkdir /var/run/sshd
RUN echo 'root:mypassword' | chpasswd
RUN sed -i 's/PermitRootLogin prohibit-password/PermitRootLogin yes/' /etc/ssh/sshd_config
RUN sed 's@session\s*required\s*pam_loginuid.so@session optional pam_loginuid.so@g' -i /etc/pam.d/sshd
EXPOSE 22
CMD ["/usr/sbin/sshd", "-D"]
3. Next, run the docker build
command to create the Docker image. The t
flag tags the image sshd_container
and.
allows Docker to pick all the necessary files from the present working directory.
# Building the docker Image
sudo docker build -t sshd_tagged_image .
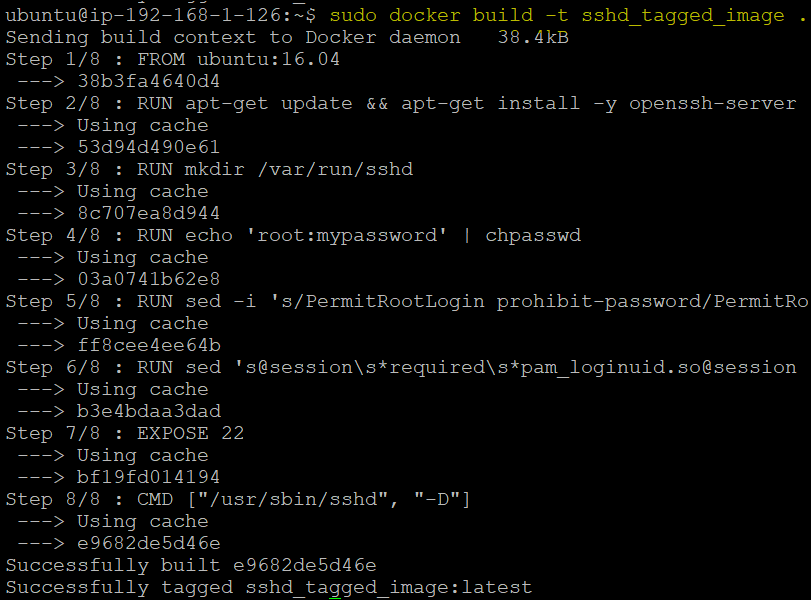
4. Now, run the docker images
command to inspect the created image. Note the REPOSITORY attribute. This attribute is the tag created with the -t
flag in the previous step.

5. Run docker run
to create and run the container from the image telling Docker to run the image in the background (-d
),
The command below instructs Docker to create and run the container called test_sshd_container
in the background (-d
), using the sshd_tagged_image
newly built image that you created in step 3 and to publish all ports defined in the Dockerfile as random ports.
# Running the container using the newly built image
docker run -d -P --name test_sshd_container sshd_tagged_image
After the successful execution of the Docker run command, you will see that the container ID is generated below.

6. Run docker port
to verify SSH connectivity between the Docker host and the container. The docker port
command list’s the port mappings or a specific mapping for the container.
sudo docker port test_sshd_container
You should see the output of 22/TCP → 0.0.0.0:32769
, which indicates the container’s port 22 is mapped to the external port 32769
.

7. Next, find the IP address of the container. To do that, run the docker inspect
command. The docker inspect
command queries Docker information and renders the results in JSON array using a format
parameter.
You’ll see the format
parameter argument below uses the range
attribute to find the container’s IP address by checking in NetworkSettings
→Networks
→ IPAddress
.
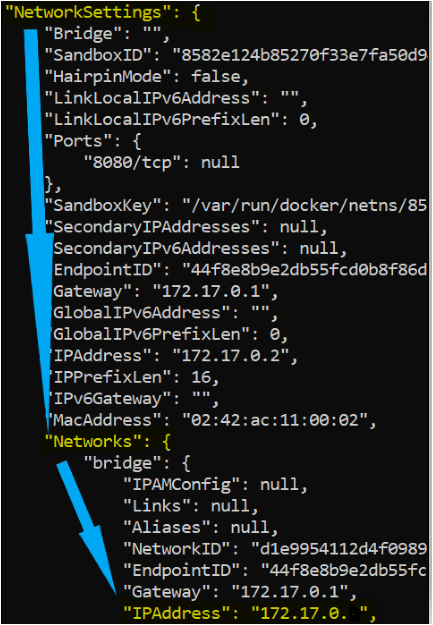
docker inspect --format='{{range .NetworkSettings.Networks}}{{.IPAddress}}{{end}}' test_sshd_container

8. Finally, now that you have the IP address to SSH to, try to SSH to the container, and it should work!
ssh root@Ip-Address-of-the-container
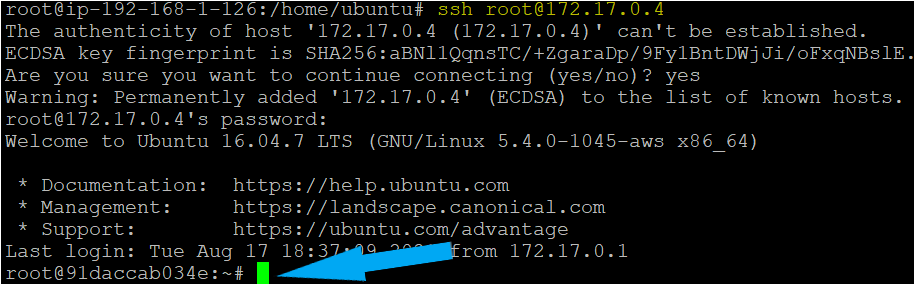
Conclusion
You show now know a few ways to SSH to a Docker container using a few different approaches. Using one of these approaches, you should be able to troubleshoot and manage your containers.
With this newfound knowledge, how do you plan to SSH to your container now?