If you need to transfer files securely with SFTP and know a little about PowerShell, you’re in luck. In this tutorial, you will learn how to work with files remotely via SFTP and dig into some of the different PowerShell SFTP modules you can use.
Not a reader? Watch this related video.Let’s dive in!
Prerequisites
This tutorial will be a hands-on demonstration. If you’d like to follow along, be sure you have the following:
- A remote SFTP server – This tutorial will use Windows Server 2019’s FTP server.
- PowerShell – You can use both Windows PowerShell 5.1 or PowerShell v7+.
Managing Files over PowerShell SFTP with the Posh-SSH Module
The Posh-SSH module is a handy module to work with files over SFTP. To get started, open PowerShell as administrator and run Install-Module
to download and install the module from the PowerShell Gallery. If prompted about an untrusted repository, type Y or A to confirm.
Install-Module -Name Posh-SSH
Downloading Files and Folders from the SSH server
Now that you have installed the module let’s look at downloading files. To download files, you must first set up a session. The session is a persistent connection you’ll use to perform various SFTP operations.
To set up a session, provide a PSCredential object via Get-Credential
and specify the remote SFTP server you’d like to connect to using the New-SFTPSession
command. When invoked via the command below, PowerShell will prompt you for a username and password.
$SFTPSession = New-SFTPSession -ComputerName 10.0.0.10 -Credential (Get-Credential)
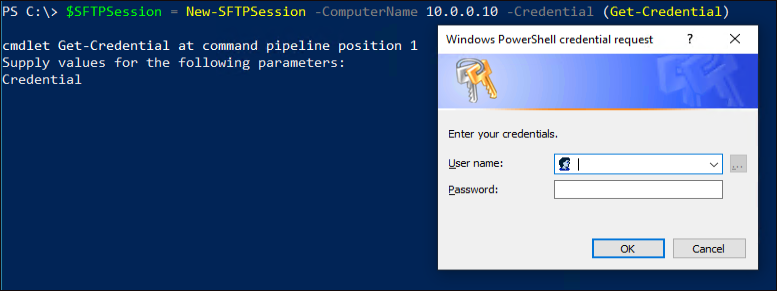
If the connection is successful, the command will prompt you to accept the Server SSH Fingerprint. This certificate fingerprint will be how the server trusts the connection from your client.
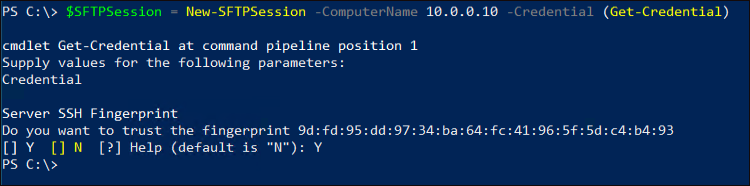
Now that you’ve connected to the remote server run the below command to download a file from the remote server. This Get-SFTPItem
command downloads the /home/user01/Downloads/test.txt file on the remote server to the C:\temp location on the local computer. You’ll also see that the command below uses the SessionId
property on the SFTPSession
object captured in the last step.
Get-SFTPItem -SessionId $SFTPSession.SessionId -Path /home/user01/Downloads/test.txt -Destination c:\temp
You can also download entire folders by providing a path such as
/home/user01/Downloads
to thePath
parameter.
Uploading Files and Folders to the SSH server
Now that you’ve seen how to download files and folders from your SSH server let’s look at how to upload files and folders. The method to upload files is nearly identical to downloading files, as you can see below.
Invoke the Set-SFTPItem
command providing the session to connect to, the file to upload, and the location on the SSH server to upload the file to.
Set-SFTPItem -SessionId $SFTPSession.SessionId -Path C:\Temp\test.txt -Destination /tmp
You can also upload entire folders by providing a folder path such as
C:\Temp\TestFolder
to thePath
parameter.
Disconnecting the Posh-SSH Session
Finally, once you are done using the Posh-SSH session, disconnect and remove it from memory with the Remove-SFTPSession
. Although this step isn’t mandatory, doing so will cleanly disconnect the server’s connection and free up memory in PowerShell.
Remove-SFTPSession -SFTPSession $SFTPSession
Managing Files over SFTP with the WinSCP Module
If you’d prefer not to use the Posh-SSH module for whatever reason, you always have another option; the WinSCP module! The WinSCP module is another handy module that comes from the popular WinSCP GUI utility.
To get started, like the Posh-SSH module, first download and install it from the PowerShell Gallery, as shown below.
Install-Module -Name WinSCP
Inspecting Files and Folders with the WinSCP Module
Now that you’ve installed the module, you can get started using it. Let’s first make a connection to the server. Like the Posh-SSH module, you must first always establish a connection to the remote server.
The first time you attempt to establish a connection to the SFTP server, the New-WinSCPSession
command will prompt you to add the server’s public key to your local certificate repository. You must do this so that the SFTP server will trust your local computer.
Click the Copy key fingerprints to clipboard and paste the fingerprint into a text window to add your key. You’ll need this in the next step. Next, copy the line that looks like “ssh-ed25519 255 48:b4:4e:3d:67:ca:25:e4:c7:77:fe:3c:df:ae:d9:b9” anCopy and paste that fingerprint in the script as shown in below script.
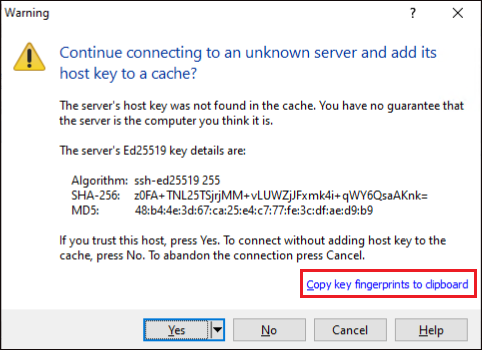
To establish a connection, below you’ll see an example script. This script creates a PSCredential object that is passed to the Credential
parameter of the New-WinSCPSessionOption
command and the remote server to connect to and the protocol sftp
.
#Enter credentials to connect to FTP server.
$FTPUsername = "UserID"
$FTPPwd = "UserPwd"
$Password = ConvertTo-SecureString $FTPPwd -AsPlainText -Force
$Credential = New-Object System.Management.Automation.PSCredential ($FTPUsername, $Password)
#Import WinSCP module
Import-Module WinSCP
#Create WinSCP session to your FTP server. 10.0.0.3 is the FTP server.
$WinSCPSession = PS C:\> New-WinSCPSession -SessionOption (New-WinSCPSessionOption -HostName 10.0.0.3 -Protocol Sftp -Credential $Credential -SshHostKeyFingerprint "ssh-ed25519 255 48:b4:4e:3d:67:ca:25:e4:c7:77:fe:3c:df:ae:d9:b9")
Now that you’ve established a connection to the server run the Get-WinSCPChildItem
command and provide the session you just created. This command will enumerate all the files and folders on the remote server from the remote server user’s home directory.
Get-WinSCPChildItem -WinSCPSession $WinSCPSession
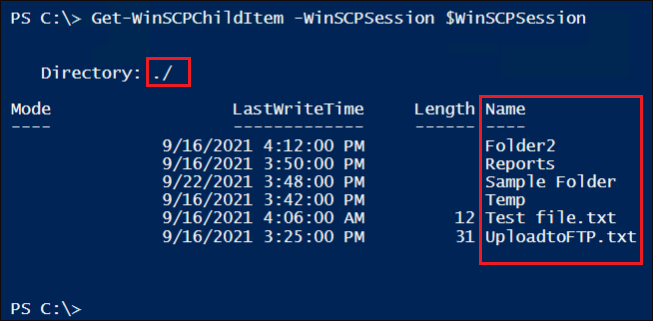
To only list files in a particular directory, specify the Path
parameter as shown below.
Get-WinSCPChildItem -WinSCPSession $WinSCPSession -Path '/Folder2'
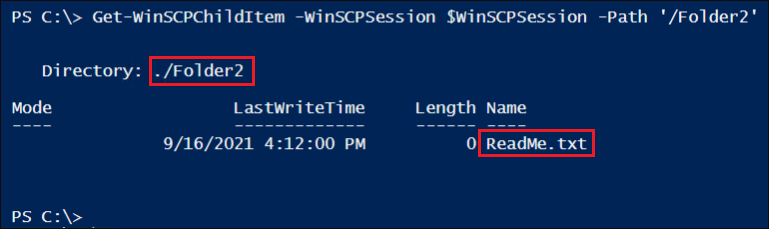
Uploading Files and Folders with the WinSCP Module
Uploading files and folders is similar to listing files with the WinSCP module. To do so, run the Send-WinSCPItem
command as shown below. By specifying the session (WinSCPSession
), the local Path
of the file to upload, and the RemotePath
to where the file should go, Send-WinSCPItem
will upload the file.
By default, if you do not specify a path using -RemotePath
parameter, your files and folders will be uploaded to the user’s home directory you authenticated to the server with.
Send-WinSCPItem -WinSCPSession $WinSCPSession -Path C:\Temp\TestFile.txt -RemotePath '/Folder2/'
When the upload completes, you’ll see that Send-WinSCPItem
displays the remote folder path containing the file uploaded.
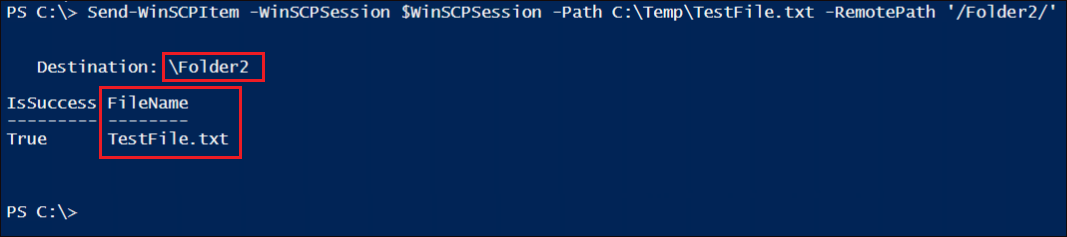
You can upload entire folders by providing a path to the
Path
parameter rather than a single file.
Downloading Files and Folders with the WinSCP Module
Downloading files and files with the WinSCP is possible, too, using the Receive-WinSCPItem
command, as shown below. To download files, specify the WinSCPSession
again, provide the path to the file or folder you’d like to download via the RemotePath
parameter and the local folder you’d like to download to.
Receive-WinSCPItem -WinSCPSession $WinSCPSession -RemotePath '/Folder2/ReadMe.txt' -LocalPath C:\Temp
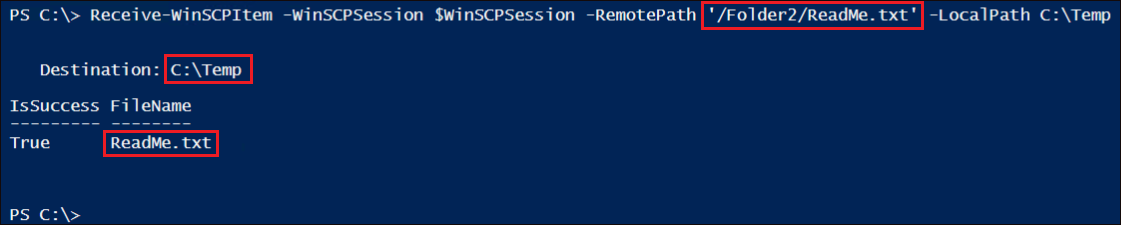
Disconnecting the WinSCP Session
Once you are done using the WinSCP session, disconnect and remove it using the Remove-WinSCPSession
.
Remove-WinSCPSession -WinSCPSession $WinSCPSession
Conclusion
You should now know how to list, upload and download files via PowerShell SFTP. Thankfully, the community has made modules for everyone to use freely to make this job much more manageable.
Which module do you prefer to work with files over PowerShell SFTP?