Counting or calculating numeric properties of objects, like characters, words, and lines in string objects, can be exasperating. Fortunately, the PowerShell Measure-Object
cmdlet is up to the task.
In this tutorial, you will learn the power of the Measure-Object
cmdlet in automating measuring object properties in PowerShell.
Dive right in to enhance your object-measuring skills!
Prerequisites
This tutorial is a hands-on demonstration. To follow along, you will need a system with PowerShell installed. This tutorial uses Windows 10 with PowerShell v7.
Counting Files and Folders
Knowing the number of files and folders in a directory can be helpful in various scenarios. One example is when you need to monitor a folder’s size or check the number of files in a directory.
The Measure-Object
cmdlet counts the number of files and folders in a directory. The exact count helps you better manage and optimize your system resources.
To see how the Measure-Object
cmdlet works in counting files and folders:
1. Open PowerShell, and run the following Get-ChildItem
command to count and return the total files and folders collectively (Measure-Object
) in your current directory.
Get-ChildItem | Measure-Object
The output below indicates 4852 files and folders in the current directory.
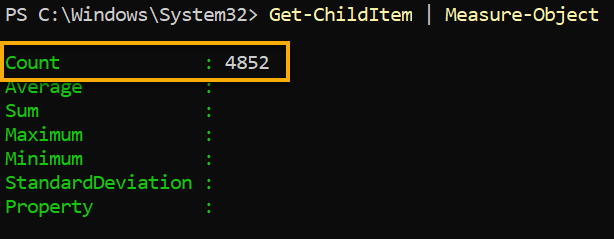
Perhaps you wish to include all files and subdirectories in the directory tree recursively. If so, append the
-Recurse
parameter, as shown below.Get-ChildItem -Recurse | Measure-Object
2. Next, run the same command below as you did in step one. But this time, append the -Directory
parameter to only count the total directories within the working directory.
Get-ChildItem -Directory | Measure-Object
As you can see below, the total count is now lower (135) since the command only counted directories, excluding all other objects.
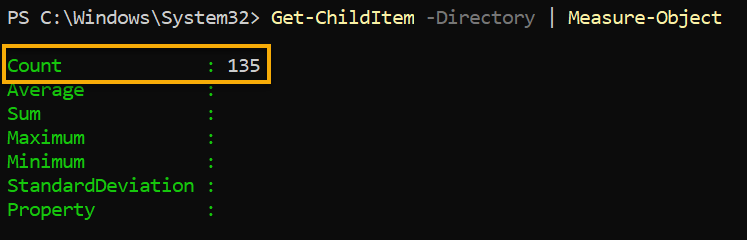
3. Now, run the below command to count the executable files (.exe
) in your current directory.
The -Filter
parameter tells the Get-ChildItem
cmdlet to filter the results and only return the files with the extension you specify (i.e., *.txt
, *.jpg
, *.csv
).
Get-ChildItem -Filter *.exe | Measure-Object
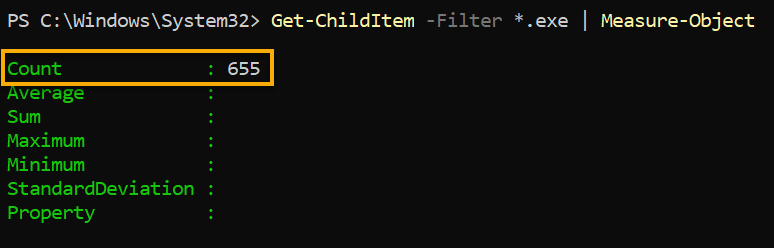
Measuring File Sizes
Measuring object lengths and sizes can help better manage your system since large files affect your system’s performance. In addition to counting properties, you can also measure the length and size of objects via the Measure-Object
cmdlet.
Run the code below to retrieve all files in the current directory (Get-ChildItem
) and display (Write-Host
) their respective lengths in bytes by default.
The code below outputs each measurement to the console, including the minimum, and maximum file sizes, average file size, the sum of file sizes, and the total number of files.
# Set the threshold for the average file size
$threshold = 5000000
# Measure the size of each file in the current directory
$files = Get-ChildItem | Measure-Object -Property length -Minimum -Maximum -Sum -Average
Write-Host "Minimum file size: $($files.Minimum) bytes"
Write-Host "Maximum file size: $($files.Maximum) bytes"
Write-Host "Sum of file sizes: $($files.Sum) bytes"
Write-Host "Average file size: $($files.Average) bytes"
Write-Host "Total number of files: $($files.Count)"
# Check whether the average file size is above or below the threshold
if ($files.Average -ge $threshold) {
# If the average file size is above the threshold, play a high-pitched sound
[console]::beep(1000, 500)
} else {
# If the average file size is below the threshold, play a low-pitched sound
[console]::beep(500, 500)
}
Below, you can see the sizes displayed in bytes.
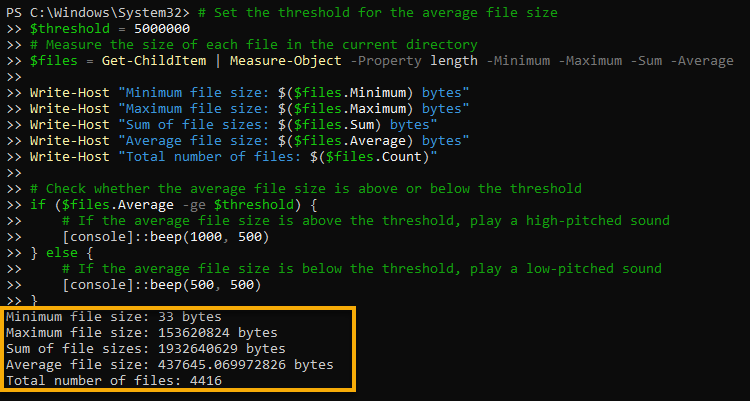
Perhaps pasting the code to the console is not your thing. If so, save the code to a PowerShell script file and run it.
Now, run the following commands to get a file in the current directory, where (Where-Object
) its size ($_.Length
) is equal (-eq
) to the maximum file size ($files.Maximum
).
# Get and store the file the same size as the $files.Maximum
$max = Get-ChildItem | Where-Object -FilterScript {$_.Length -eq $files.Maximum}
# Display the value of the maximum size ($files.Maximum) and the file with that size
Write-Host "Maximum file size: $($files.Maximum) bytes `nFile: $max"
The output below shows the name of the most extensive file in the current directory.

Measuring Text File Contents
Instead of measuring file sizes, you may need to determine the number of lines, words, or characters in a text file. If so, the Measure-Object
cmdlet has many other parameters to measure text file contents.
Run the code below to perform the following measure the text file’s content (Measure-Object
) by -Character
, -Line
, and -Word
, and output (Write-Host
) the measurements.
Ensure you change the values of the $file_path
and $file_content
variables with your preferred ones.
# Define the file path and content
$file_path = "C:\Temp\measure-object.txt"
$file_content = "One fish, two fishes, three fishes"
# Create the file against the defined file path and content
Set-Content -Path $file_path -Value $file_content
# Measure the contents of the text file
$measurements = Get-Content -Path $file_path | Measure-Object -Character -Line -Word
# Output the measurements to the console
Write-Host "The file '$file_path' contains $($measurements.Lines) lines, $($measurements.Words) words, and $($measurements.Characters) characters."
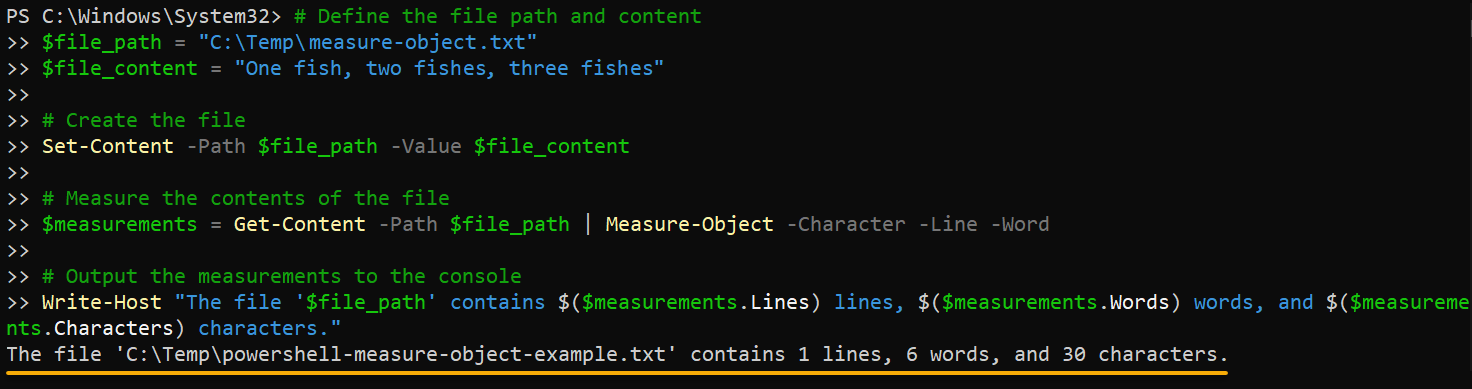
Measuring Boolean Values
Boolean values are values that can only be true or false. In PowerShell, Boolean values are often used to control the flow of a script, determine a condition (true or false), or indicate a command’s success or failure.
Measuring Boolean values can be useful in various scenarios, such as:
- Counting the number of true or false values in a dataset.
- Determining the percentage of true values relative to the total number of values.
This information can help make sound decisions in processing or analyzing data.
To see how measuring Boolean values work:
Run the following commands to count the sum of Boolean values (true values by default) in the array ($values
).
# Define an array of Boolean values ($true and $false).
$values = $true,$false,$false,$true,$true,$true,$false,$false,$true
# Measure (count) the Boolean values (true values by default).
$values | Measure-Object -Sum
Below, the total Count of the Boolean values is nine (9), while the Sum is five (5) since only the true values are counted.
What about the false values? Jump to the following step, and run a few more commands.
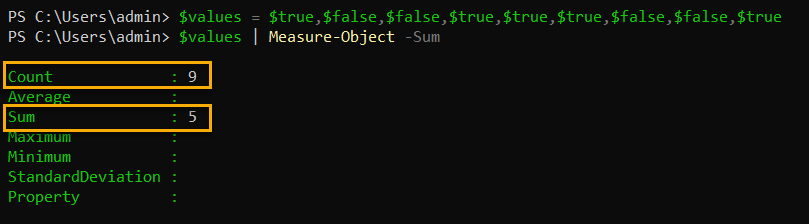
Now, run the code below to subtract the sum of true values ($trueCount
) from the total Boolean values count ($count
). The difference from the equation is the total of false values ($falseCount
).
# Get the total count of Boolean values in an array
$count = $values.Count
# Get the sum of true values
$trueCount = ($values | Measure-Object -Sum).Sum
# Subtract the total of true values from the total Boolean values
# to get the total of the false values
$falseCount = $count - $trueCount
# Display total counts of both true and false values
Write-Host "Number of true values: $trueCount"
Write-Host "Number of false values: $falseCount"
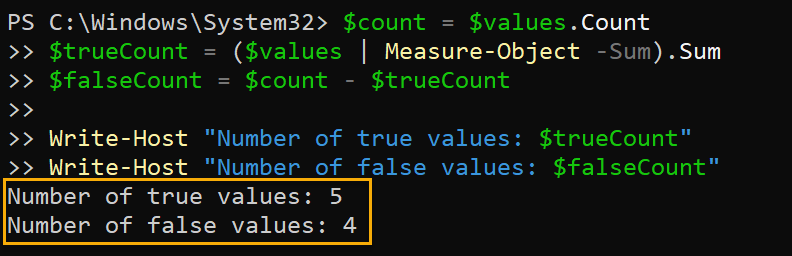
If measuring Boolean values is a daily task, you can create a function you can call anytime to run the task.
Measuring Hashtables
Hashtables in PowerShell (a collection of key-value pairs) allow you to store and retrieve data quickly and efficiently. If you need the number of items in a hashtable or the maximum and minimum values, let the Measure-Object
cmdlet measure your hashtables.
Run the commands below to find the Num
property’s -Minimum
and -Maximum
values in your hashtable ($MyHastable
).
# Create a hashtable containing three hashtables, each with a Num property.
$MyHashtable = @(@{Num=10}, @{Num=20}, @{Num=30})
# Measure the hashtable's Num property by minimum and maximum.
$Stats = $MyHashtable | Measure-Object -Minimum -Maximum Num
# Display the hashtable measurements
$Stats
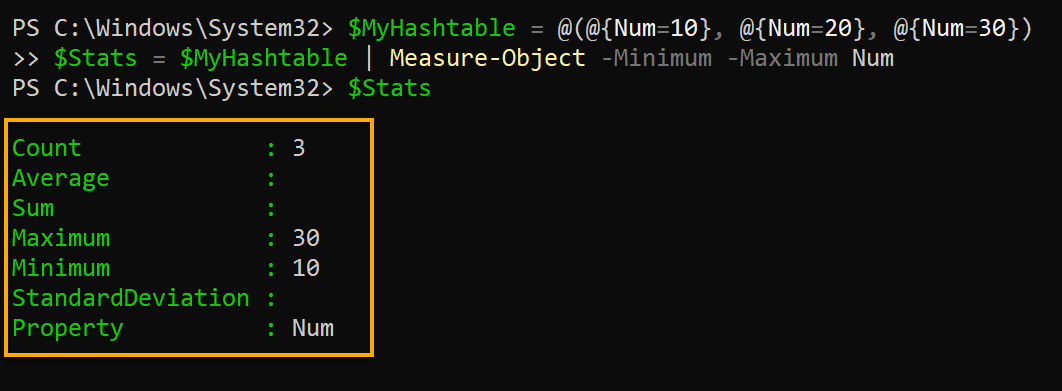
Measuring Standard Deviations
Reviewing data sets can be rambling, but it does not have to be. Measuring the standard deviation is handy when understanding the distribution of data set values. You can also identify any outliers or unusual data points affecting the results.
The standard deviation measures the variation or dispersion data set values from the mean (average) value. This measurement is calculated by finding the square root of the variance of the data, which is the average of the squared differences from the mean.
Run the code below to calculate and display the -Average
and -StandardDeviation
of an array of numbers stored in the $Numbers
variable.
# Define an array of numbers
$Numbers = 10, 20, 30, 40, 50
# Calculate the average and standard deviation of the numbers
$Average = ($Numbers | Measure-Object -Average).Average
$StdDev = ($Numbers | Measure-Object -StandardDeviation).StandardDeviation
# Output the results
"Average: $Average"
"Standard deviation: $StdDev"
In statistics, the mean measures central tendency, describing the typical or most representative value of a data set.
The average is calculated by adding all the numbers in a data set and dividing by the total number of values. In this example, the data set is {10
, 20
, 30
, 40
, 50
} with a sum of 150, and five numbers (5
) are in the set.
Consequently, the average is calculated below, which is 30
.
Average = (10 + 20 + 30 + 40 + 50) / 5 = 30
On the other hand, the standard deviation measures the spread or dispersion of a set of data. The standard deviation is calculated as follows:
1. Find the difference between each data point and the average:
10 - 30 = -20
20 - 30 = -10
30 - 30 = 0
40 - 30 = 10
50 - 30 = 20
2. Square each of these differences:
(-20)^2 = 400
(-10)^2 = 100
0^2 = 0
10^2 = 100
20^2 = 400
3. Add up all the squared differences:
400 + 100 + 0 + 100 + 400 = 1000
4. Divide the sum of squared differences by the total number of values:
1000 / 5 = 200
5. Take the square root of the result:
sqrt(200) = 14.142135623731
The standard deviation of this set of data is approximately 14.14. But
in this case, the result is 15.8113883008419
because of floating-point arithmetic limitations in computers.
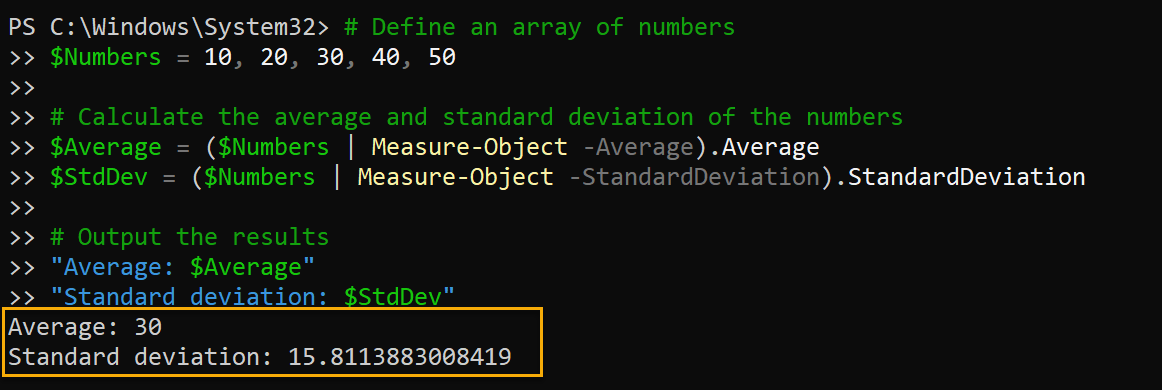
Conclusion
Measuring data is an essential task, and the PowerShell Measure-Object
cmdlet is a powerful tool to complete the task. And in this tutorial, you have learned how to measure data in many ways. Whether you are working with files, text file contents, Boolean values, strings, arrays, or hashtables, measuring them can provide valuable insights.
Now, you can easily calculate the average, maximum, and minimum values of your data and measure the variation or spread of your data. With these features, you can gain a deeper understanding of your data and make informed decisions based on your analysis.
With this newfound knowledge, why not expand your PowerShell toolkit and add Compare-Object
to your arsenal?