If you find yourself creating a lot of AWS Lambda functions in larger automation routines, building AWS Lambda in Terraform might be the right way to go. Terraform and AWS Lambda? Yep. You Can do that. Building Lambda functions in Terraform eliminates manual effort and allows you to speed up your automation efforts.
Not a reader? Watch this related video tutorial!In this tutorial, you will learn how to build and run a configuration to build an AWS Lambda function with Terraform from scratch!
Let’s dig in!
Prerequisites
This post will be a step-by-step tutorial. If you’d like to follow along, ensure you have the following in place:
- An Amazon Web Service account(AWS).
- Terraform – This tutorial will use Terraform v0.14.9 running on Ubuntu 18.04.5 LTS, but any operating system with Terraform should work.
- A code editor – Even though you can use any text editor to work with Terraform configuration files, you should have one that understands the HCL Terraform language. Try out Visual Studio (VS) Code.
Building the Terraform Configuration
Terraform is an infrastructure as code tool that allows you to build, change, and version infrastructure via a configuration. Let’s start off this tutorial by building that configuration to eventually build an AWS Lambda function.
1. Create a folder named ~/terraform-lambda-function-demo then change (cd
) the working directory to that folder. This folder will contain your configuration file and all associated files that Terraform will create.
mkdir ~/terraform-lambda-function-demo
cd ~/terraform-lambda-function-demo
2. Open your favorite code editor and copy/paste the below configuration and save the file as main.tf inside of the ~/terraform-lambda-function-demo directory.
The main.tf file below creates a few necessary resources:
- An AWS Lambda IAM role – Grants the soon-to-be-created function permission to access AWS services and resources. This role is used while creating the Lambda function and used while running or invoking the function.
- An AWS Identity and Access Management (IAM) Policy – Allows the Lambda function to create the logs and events. This policy will be attached to the Lambda role.
- The Lambda function – The actual function which will be invoked when required. The Lambda function needs code and an IAM role to run a function.
# Creating IAM role so that Lambda service to assume the role and access other
AWS services.
resource "aws_iam_role" "lambda_role" {
name = "iam_role_lambda_function"
assume_role_policy = <<EOF
{
"Version": "2012-10-17",
"Statement": [
{
"Action": "sts:AssumeRole",
"Principal": {
"Service": "lambda.amazonaws.com"
},
"Effect": "Allow",
"Sid": ""
}
]
}
EOF
}
# IAM policy for logging from a lambda
resource "aws_iam_policy" "lambda_logging" {
name = "iam_policy_lambda_logging_function"
path = "/"
description = "IAM policy for logging from a lambda"
policy = <<EOF
{
"Version": "2012-10-17",
"Statement": [
{
"Action": [
"logs:CreateLogGroup",
"logs:CreateLogStream",
"logs:PutLogEvents"
],
"Resource": "arn:aws:logs:*:*:*",
"Effect": "Allow"
}
]
}
EOF
}
# Policy Attachment on the role.
resource "aws_iam_role_policy_attachment" "policy_attach" {
role = aws_iam_role.lambda_role.name
policy_arn = aws_iam_policy.lambda_logging.arn
}
# Generates an archive from content, a file, or a directory of files.
data "archive_file" "default" {
type = "zip"
source_dir = "${path.module}/files/"
output_path = "${path.module}/myzip/python.zip"
}
# Create a lambda function
# In terraform ${path.module} is the current directory.
resource "aws_lambda_function" "lambdafunc" {
filename = "${path.module}/myzip/python.zip"
function_name = "My_Lambda_function"
role = aws_iam_role.lambda_role.arn
handler = "index.lambda_handler"
runtime = "python3.8"
depends_on = [aws_iam_role_policy_attachment.policy_attach]
}
3. Create another folder files in the ~/terraform-lambda-function-demo directory and create a Python file index.py inside the files folder. This Python script will contain the code that the function will execute.
mkdir files
cd /files
touch index.py
4. Now, open the index.py Python script and paste the below Python code*.* The below code contains a Python function lamda_handler
. This function uses event data that will be passed to the Lambda function at runtime. It will then parse the first_name
and last_name
and return a message
response.
# index.py
# Declaring the Python Function lambda_handler with event paramater
def lambda_handler(event, context):
message = 'Hello {} {}!'.format(event['first_name'], event['last_name'])
return {
'message' : message
}
5. Create another Terraform configuration called output.tf in ~/terraform-lambda-function-demo and paste the below content into it. This configuration file tells Terraform to reference the lambda_role
and lambda_logging
resources defined in the main.tf configuration file and output the values to the console when you run Terraform in the next section.
output "lambda_role_name" {
value = aws_iam_role.lambda_role.name
}
output "lambda_role_arn" {
value = aws_iam_role.lambda_role.arn
}
output "aws_iam_policy_lambda_logging_arn" {
value = aws_iam_policy.lambda_logging.arn
}
6. Create one more file inside the ~/terraform-lambda-function-demo directory called provider.tf and paste in the following code. This Terraform configuration file defines the Terraform AWS provider so that Terraform knows how to interact with all of the AWS resources you’ve defined in the earlier steps.
The tutorial will be creating resources in the us-east-2 region.
provider "aws" {
region = "us-east-2"
}
7. Now, open your favorite terminal and verify all of the required files below are contained in the folder by running the tree
command.
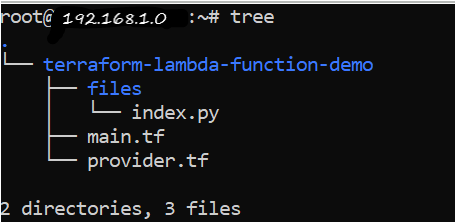
Initiating Terraform and AWS Lambda Function
Now that you have the Terraform configuration files built, it’s time to initiate Terraform and create the AWS Lambda function! To provision a Terraform configuration, Terraform typically uses a three-command approach in sequential order:
Let’s run each of the stages now.
- Open a terminal and navigate to the
~\\terraform-lambda-function-demo
directory.
cd ~\terraform-lambda-function-demo
- Run the
terraform init
command in the same directory. The terraform init command initializes the plugins and providers which are required to work with resources.
terraform init
If all goes well, you should see the message Terraform has been successfully initialized
in the output, as shown below.
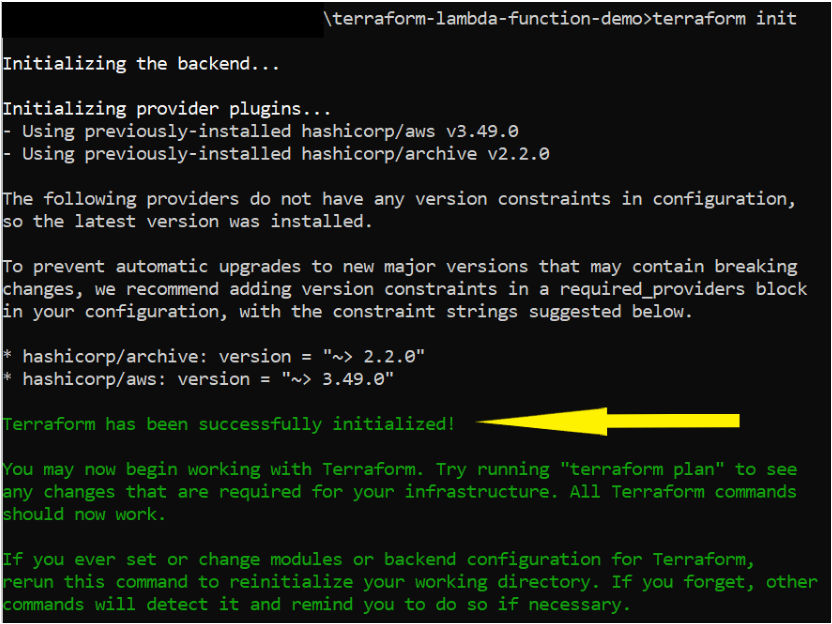
Initializing the terraform
Now, run the terraform plan
command. This Terraform command ensures your configuration’s syntax is correct and gives you an overview of which resources will be provisioned in your infrastructure.
terraform plan
If successful, you should see a message like Plan: "X" to add, "Y" to change, or "Z" to destroy
in the output to indicate the command was successful. You will also see every AWS resource Terraform intends to create.
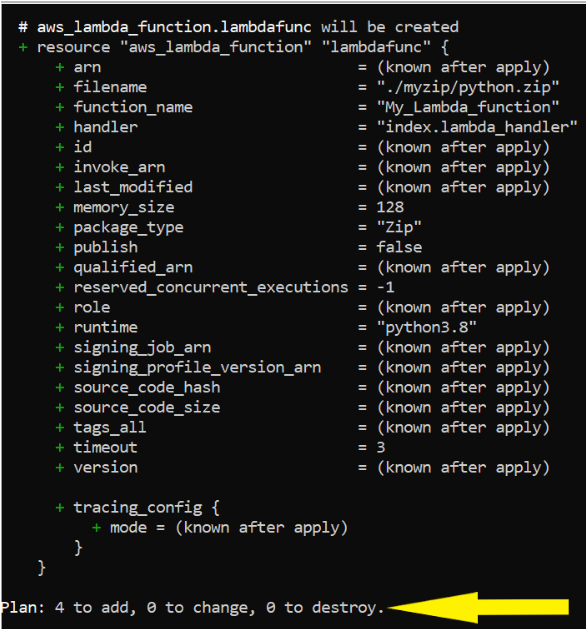
Next, run the terraform apply
command to provision the AWS Lambda function. When you invoke, terraform apply,
Terraform will read the configuration (main.tf) and the other files to compile a configuration. It will then send that configuration to AWS as instructions to build the Lambda function and other components. terraform apply
terraform apply
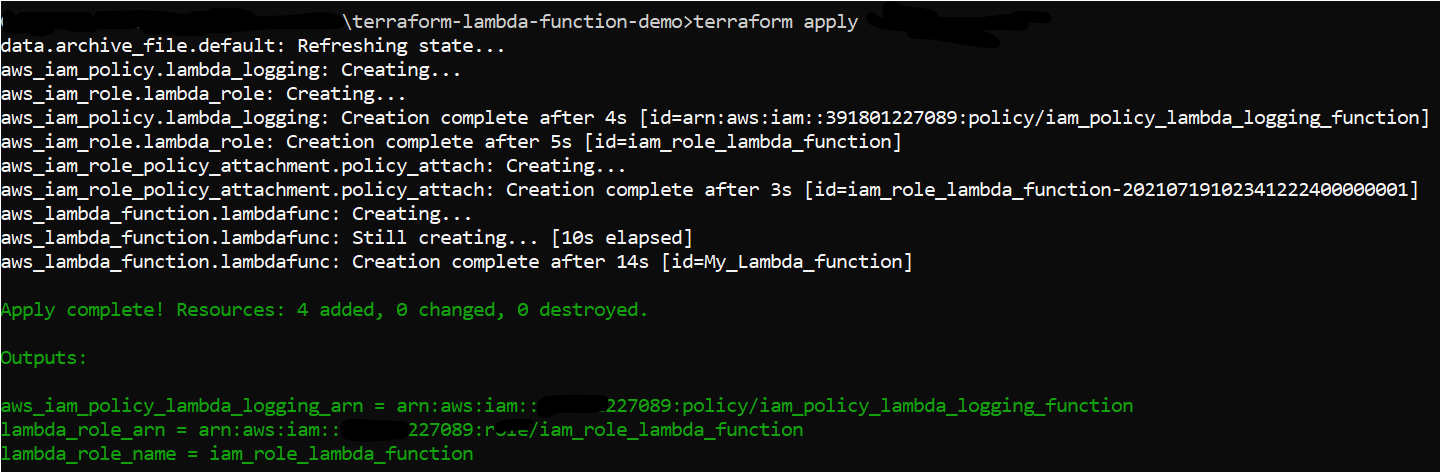
Verifying the AWS Lambda function
Now that you have created the Lambda function with Terraform, let’s now verify it’s actually there by manually checking for the Lambda function in the AWS Management Console.
1. Open your favorite web browser and navigate to the AWS Management Console and log in.
2. While in the Console, click on the search bar at the top, search for ‘Lambda,’ and click on the Lambda menu item.
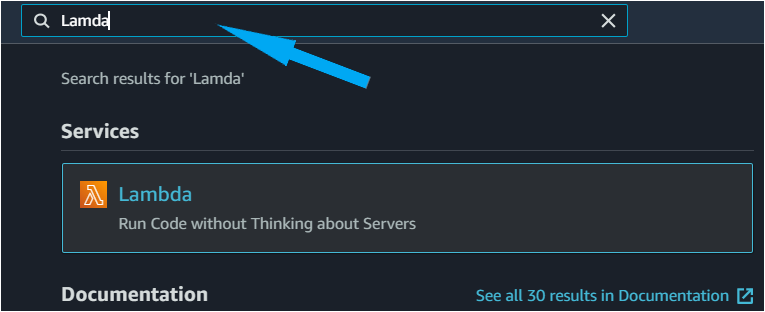
- Once on the Lambda page, click on Functions. You should see the My_Lambda_function created successfully.
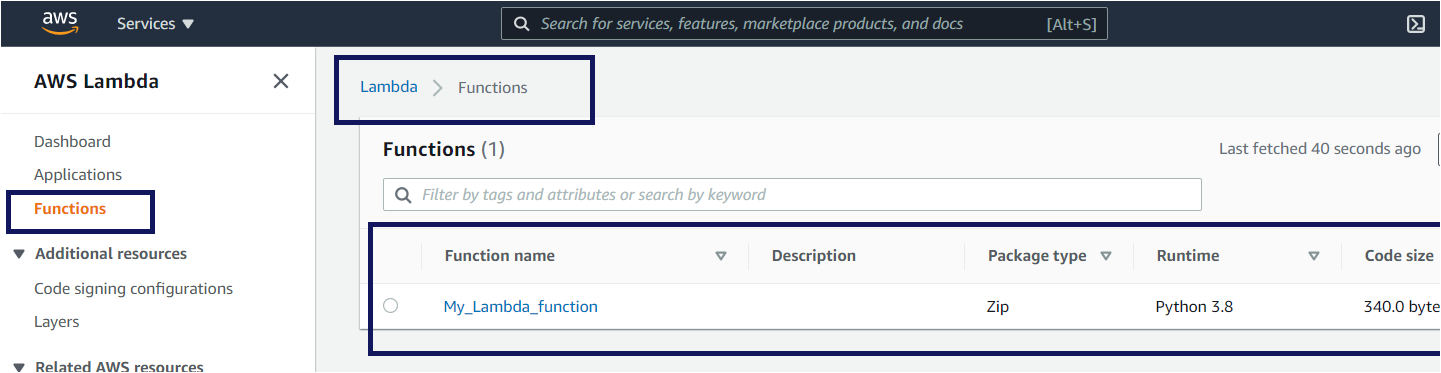
4. Next, click on the Test button to test the Lambda function that you created, as shown below.
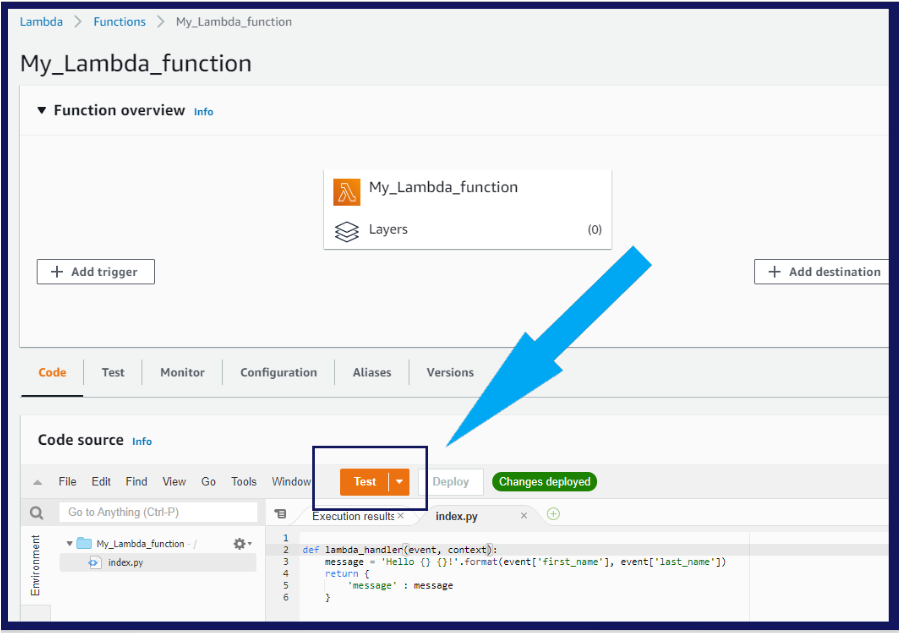
5. After you hit the Test Button for the first time, you will be prompted to configure test event details. These test events will be used as a parameter to run the Python code in the Lambda function that you created earlier in step 4 of Building the Terraform Configuration for an AWS Lambda functions section.
In the below Configure test event windows, the name of the event is Fullname
, and the key: value
pair is first_name: Adam
and last_name: Lisktek
, respectively.
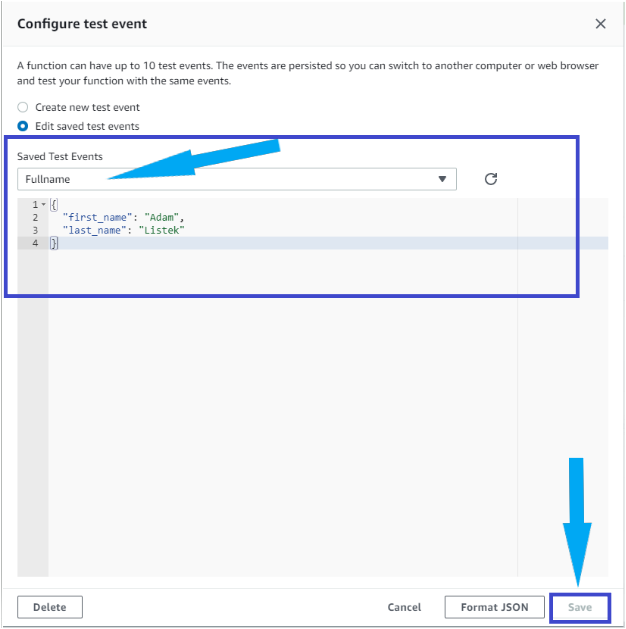
Now, again click on the Test button to test the Lambda function. This time you will see that the Lambda function executed successfully with the response message: Hello Adam listek
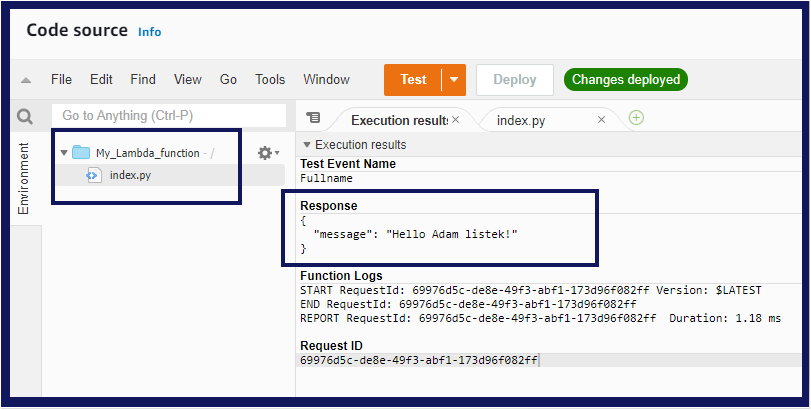
Conclusion
In this tutorial, you’ve learned how to use Terraform to deploy an AWS Lambda function and its components using Terraform.
Building a serverless application with Lambda allows you to scale when needed and is a quick task.
You are now ready to use this knowledge with other AWS services and build powerful services on top of it.