Python has always remained the first choice for most of the IT folks who love automation. With Python, you only need a few lines of code to automate various IT tasks, one of those is working with files. Python read files, create them, and a whole lot more.
In this tutorial, you will learn how to write Python code to open, read and write to text files with tons of examples.
Let’s get started!
Prerequisites
This tutorial will be a step-by-step tutorial. If you’d like to follow along, ensure you have the following in place:
- Python v3.6 or later – This tutorial will be using Python v3.9.2 on a Windows 10 machine.
- A code editor – This tutorial will use Visual Studio (VS) Code.
Opening a Text File with Python
Let’s get this tutorial started by first learning how to open a file for reading in Python.
1. Open your favorite code editor; preferably one like VS Code.
2. Create a simple text file inside the home directory (~) and name it as devops.txt with the text *”*Hello, ATA friends.”
3. Now, create a file and copy the Python code shown below to it and then save it as a Python script called ata_python_read_file_demo.py in your home directory.
# newfile is the file object , devops.txt is the file to be opened and default Access mode is read
newfile = open('devops.txt')
# Declaring the Access mode "read" exclusively
# newfile = open('devops.txt' , 'r')
print(newfile)
The above script contains the Python built-in method open()
that opens the devops.txt file for reading without declaring an access mode. The open()
method then returns a file stream which gets captured in the newfile
variable.
Once you have a file object, that object contains methods and attributes to perform various operations. When opening files, depending on what you intend to do with the file, you must define a specific mode as explained above in the inline comments. There are various modes to open files in Python.
r
– Open the file for reading. This is the default access mode.w
– Open the file for writing.x
– This option creates a new file if it no file exists but fails if already present.a
– Opens the file for writing and appends the data at the end of file.b
– Opens the file in binary mode. This mode is used to work with files other than text such as pdf, images, etc.t
– Open the file in text mode. This is the default mode.+
– Opens the file to read or write.
4. Now, execute the Python script.
python ata_python_read_file_demo.py
The output will display the file stream with details such as type of file, access mode, and encoding.

Once you have the file open, you can then read, write or modify it many different ways.
Reading a Text File with Python
Once you have a file open in Python, it’s time to do something to it. Let’s first cover how to read a text file. If you need to read the content inside the file, you’ll need to use a Python function called read()
.
With the ata_python_read_file_demo.py Python script open in your code editor from above, replace the text with the following Python code, save and execute it.
The script below reads the entire file as a file stream, reads each line of the file one at a time and reads the first five characters of each line.
# Reading the file as one file stream
file = open('devops.txt', 'r')
# Reads the entire file at once and places the contents in memory.
read_content = a.read()
print(read_content)
# Iterating over each line in the text file with the with keyword
# and reading each line one at a time
with open('devops.txt', 'r') as line:
# Reads a specific line of text in the file.
line_text = c.readline()
print(line_text)
# Iterating over each line in the text file with the with keyword
# and reading the first five characters of each line
with open('devops.txt', 'r') as line:
# Reads a specific number of characters from a single line of text in the file.
read_char = line.read(5)
print(read_char)
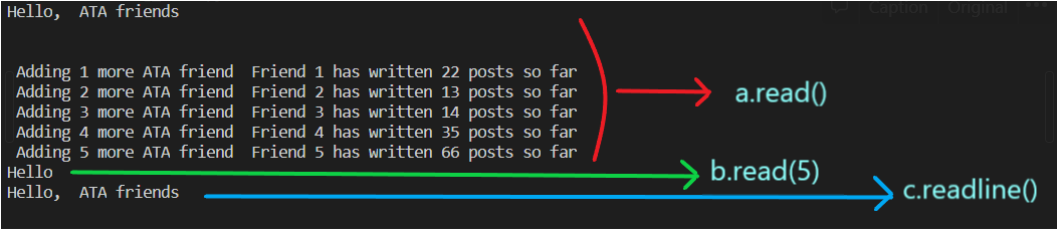
Reading a CSV File with Python
You’ve learned that Python can open simple text files. CSV files are, in actuality, just text files with a specific schema. You could use the open()
method using the read()
or readline()
method to read CSV rows but it wouldn’t be too useful. Why? Because the read
methods don’t understand a CSV file’s schema.
To read a CSV file so that Python understands the data the CSV stores, use the CSV Python module.
Assuming you are still in your code editor:
1. Open another tab and paste in the following CSV data and save it as ata_csv_demo.csv. You’ll see that this CSV data contains four columns and four rows.
Date, PreviousUserCount, UserCountTotal, sitepage
02-01-2021,61,5336, ATA.com/blog
03-01-2021,42,5378, ATA.com/blog1
04-01-2021,26,5404, ATA.com/blog2
05-01-2021,65,5469, ATA.com/blog3
2. Now, open another tab, create the following Python script save it as a Python script with a name of your choosing. This Python script below:
- Imports the
csv
module to make the methods available. - Opens the ata_csv_demo.csv file for reading.
- Reads each row in the CSV file with the
reader()
method telling Python that the row is delimited with a comma. - Uses a
for
loop to read the text in each row returning a Python list for each CSV record.
# Import the CSV module
import csv
# Open the CSV file for reading and begin iterating over each row
with open('ata_csv_demo.csv' , 'r') as csv_row:
## Parse the line as comma-delimited
csv_data = csv.reader(csv_row, delimiter=',')
# Output specific rows from csv files using the for-loop and iterates over the range() function
for _ in range(5):
print(next(csv_data))
print(read)
In the output below, you’ll see where the script has iterated through the CSV file to show only the first 5 rows.
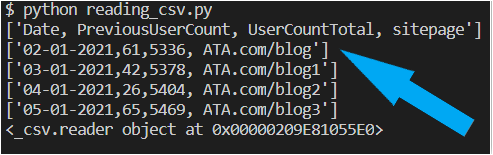
Appending to a Text File with Python
When you open a text file in Python, you aren’t just relegated to reading. You can also write to text files. Let’s cover in this section how to append text to an existing file.
You can write to a text file via one of two methods:
write()
– Writes the contents as a string to the file.writelines()
– Writes multiple strings simultaneously to the file.
Now, let’s learn to append data into a text file. To do so, open another tab in your code editor, copy and paste the following Python code, save it as a Python script name of your choosing, and execute it.
The below script is opening the devops.txt file you created earlier and appending a single line to it with the write()
method. It’s then using the writeLines()
method to append multiple lines at once.
# Open the file for appending (a) and begin reading each line
with open('devops.txt', 'a') as file:
file.write("\nAdding 5 more ATA friend")
file.writelines(['\nAdding 5 more ATA friend', '\nAdding 15 more ATA friend'])
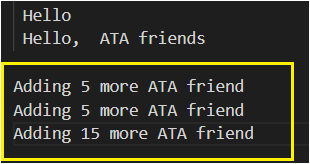
Writing Data in a CSV File with Python
By now, you have learned how to open a CSV file using Python. There are numerous times when you’ll need to add data into CSV files, such as adding your customer’s data, employees’ salary records, employee ID, etc. Python makes your life easier writing to CSV files.
To append rows into the same file devops.csv which you created earlier, open a new code editor tab and paste the following Python script into your code editor, save and execute it.
# importing the csv module
import csv
# open function within with statement
Here you used newline since the csv module does its own (universal) newline handling.
with open('devops.csv','a', newline='') as csv_file: # open() function along with append mode "a"and newline=''
write_csv = csv.writer(csv_file, delimiter=',') # csv.writer() is used to write content in the CSV file
write_csv.writerow(['08-08-2021','68','8888', 'ATA.com/blog8']) # csv.writerow() to add a row and append the contentExecute the writing_into_csv.py script using Python,
Open devops.csv and check out the last row!
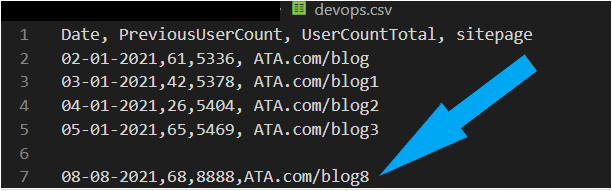
Conclusion
Today you’ve learned how to open, write and read different file types using Python. Using Python drastically reduces the chances of human errors compared to when you perform these mundane tasks manually.
Now that you can quickly and easily process data at speeds that you’ve never thought possible before, why not automate more of your data handling tasks with Python?