Python is one of the most widely used languages today. Like nearly all other programming languages, Python has the loop construct. Python loops such as the for loop and while loop allow developers to iterate collections or based on various conditions.
In this tutorial, you will learn how to create and use each type of Python loop and also how to control loops using the break
, pass
, and continue
Python statements.
Let’s begin!
Prerequisites
This post will be a step-by-step tutorial. If you’d like to follow along, ensure you have Python v3.6 or later installed. This tutorial will be using Python v3.9.2 on a Windows 10 machine.
Understanding Iterable Python Constructs
Before you can begin to learn loops, you must learn about the concept of Python iterables. Loops process collections of elements like lists, tuples, and dictionaries. Each of these constructs contains multiple elements that you can “iterate over”. As such, each construct is an iterable with elements that a loop can process individually.
When you hear someone talk about something being iterable, know that this is an element you can read each object inside of that collection with a loop.
Python For Loops
Let’s get this tutorial started by first learning for loops. For loops repeat the execution of statements or block of statements controlled by an iterable expression. In other words, they execute code for each item inside of a collection of elements.
For Loops and Strings
For example, perhaps you have an iterable item such as a string. In Python, the string is a sequence of characters. Each string consists of a combination of characters. Maybe you have a string ADAM
. This string consists of four iterable characters, A
, D
, A
, and M
.
As one string, Python only sees one element; ADAM
, as shown below.
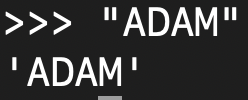
But, since Python strings are iterable, Python can “break apart” that string into an array of characters and then process each character one at a time. To do that, you’d create a for loop like below.
The for loop has a target or iterator (letter
in this case) to represent each iteration. You’d then tell Python what iterable element you’d like to process (ATA
) using the in
keyword. Each character in the character array is in the string.
Then, inside of the for loop, you’d create any code you’d like to run for each iteration. In this example, Python is simply printing each character in the character array (string).
ATA = "ADAM"
for letter in ATA:
print(letter)
You can see below that Python prints each character in the string one at a time.
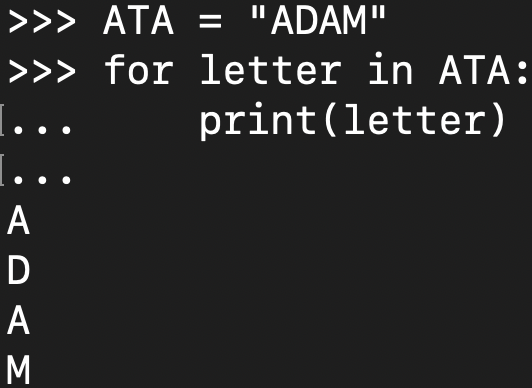
For loops can iterate over any iterable element such as lists and ranges also. Replace
ATA
in this section’s example withrange(5)
and you’ll see the same result.
Python While Loops
While for loops execute code for each item in a collection of elements, the while loop executes code based on a specific condition. More specifically, Python continues to execute a while loop while a condition is false.
For example, a common requirement in Python is to create a counter and execute code a defined number of times. To create this situation, you’d need to define a condition that would return True
when the current count exceeds the maximum count.
Let’s say you start out with a variable called count
storing an integer 0. You’d like to increment this integer by 1 but not exceed 5. You could create something like this:
count = 0
count = count + 1
count = count + 1
count = count + 1
count = count + 1
count = count + 1
print(count)
The above could work but it’s not efficient at all. You’re not using the DRY principle. You’re repeating yourself. Instead, reduce code length by using a while loop and telling Python to add one to count
while count
is less than six.
The below examples using a while loop to define a condition (count < 6
) and to execute an expression (the print
statement and increment the count
by 1 while the condition is True
.
count = 0
while (count < 6):
print('The count is:', count)
count = count + 1
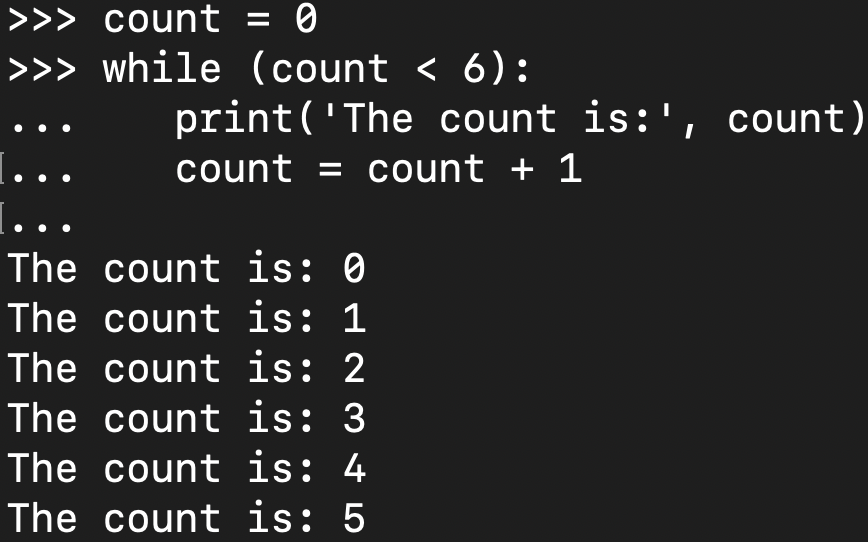
Flow Control in Loops
In the previous two examples, both the Python for and while loops started and ended all on their own. The for loop terminated because it reached the end of the iterable collection and the while loop ended because its condition evaluated to be True
.
Although it’s common for Python loops to end “normally”, you can also change the behavior of the loop to terminate prematurely or skip one or more iterations.
The Break Statement
When you need to end a loop prematurely, you can do so by using the break
statement. When executed inside of a loop, the break
statement stops the loop at the current iteration.
Typically, you’ll use the break
statement when a specific condition is met inside of a loop. For example, in the previous for loop example, you learned a for loop will iterate over an array of characters in a string. Maybe you’d like to only process all characters up to the first D
.
To stop a for loop (or even a while loop), define a certain condition you’d like to match on and then include a break
statement, as shown below.
In the below example, Python terminates the for loop the moment when the iterator variable letter
equals D
.
ATA = "ADAM"
for letter in ATA:
print(letter)
if letter == "D":
break
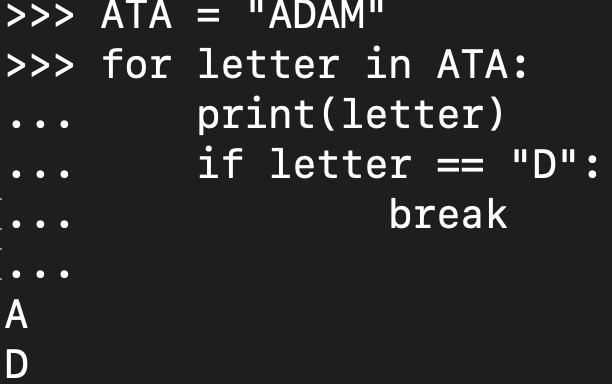
The Continue Statement
Perhaps you need to create a loop based on a specific condition but don’t necessarily want to process every iterable element. In that case, you can skip iterations with the continue
statement.
Unlike the break
statement that terminates the entire loop, the continue
statement skips the current iteration. Using the example from above, maybe you’d like to iterate over each character in the string ADAM
but skip the D
character. You could skip the D
character using a condition (if letter == "D"
) and the continue
statement, as shown below.
ATA = "ADAM"
for letter in ATA:
if letter == "D":
continue
print(letter)
You can see below Python didn’t return the D
character because the continue
statement skipped the print
statement when it encountered the D
character.
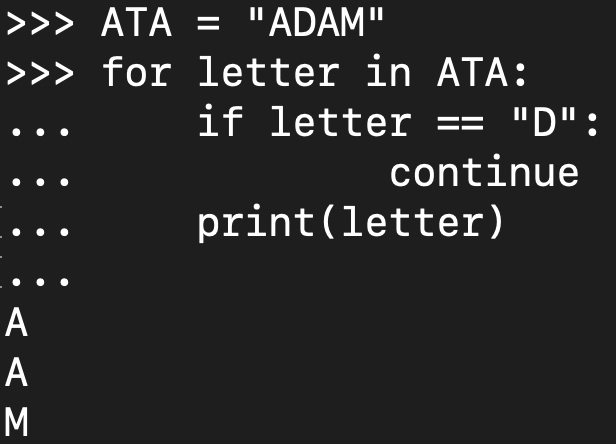
The Pass Statement
Let’s now finish off the flow control section with the pass
statement. The pass
statement is a bit unique in that it’s actually just a placeholder. The pass
statement is a way to define a loop in a Python script that actually does nothing.
Let’s say you have a for loop that you’d prefer to leave in your Python script for whatever reason. But, you don’t actually have an expression to execute inside it, like below.
for value in "adam":
When you define a for loop in Python with an expression inside, Python will return an IdentationError, as shown below.
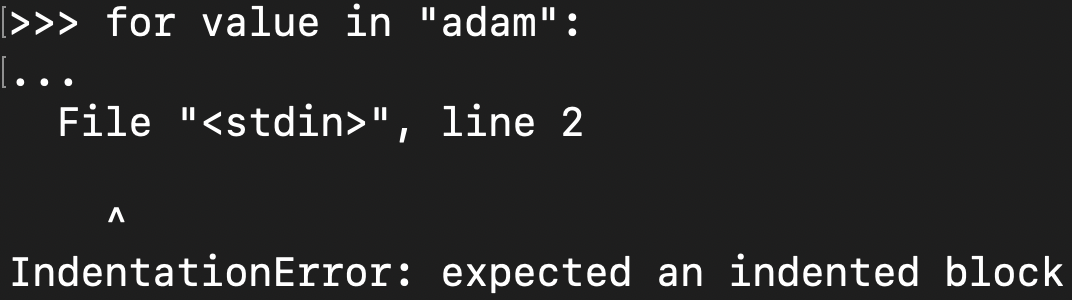
But, add the pass
statement as an expression inside of the for
loop and you’ll see Python executes the for loop essentially doing nothing.
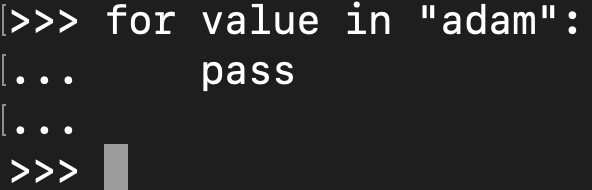
Conclusion
In this tutorial, you learned how to get started with Python loops. Learning how Python loops work and how to control them gives you way more opportunities to create and build solid Python programs.
With your newfound knowledge, where will you insert a loop in your code?