Whether you are new to Python programming or returning to it after a break, you may need to learn or re-learn about decision-making and branching statements in Python. Decision-making and branching often involve using the Python if else if, and else statements and is one of the most essential concepts in computer programming.
In this tutorial, you will learn about different if, else if, else scenarios that may arise while writing a Python program. Let’s start!
Prerequisites
This tutorial will be a hands-on demonstration. You’ll learn through examples that may come up when coding a barebones Weather app. If you would like to follow along, be sure you have the following:
- Python 3 is installed where this tutorial uses Python 3.8.
- A code editor to run Python code, where VS Code is recommended.
Testing for a Single Condition with the If Statement
As you write your programs, you will need your program to perform actions only when certain conditions are met. You will need to change the flow of your programs accordingly.
Let’s start with a construct that lets you change the flow of the code: the if statement. Let’s check how to perform actions by testing for a single condition.
An if statement executes code when the conditional statement evaluates to true. In Python, the :
symbol comes after the condition as you can see in the syntax below.
if condition:
statement(s)
Be mindful of whitespaces (or the lack of them) in Python. Python employs whitespace indentation to form code blocks. Python interprets that statements with the same number of preceding whitespace as a block code.
In case the condition evaluates to false, the program skips the code contained under the if statement which then continues with the code after the if statement.
Python interprets all non-zero values as true and all other values as false.
When talking about decision-making and branching, flowcharts help you to understand the concept better. See the below flowchart for testing a single condition.
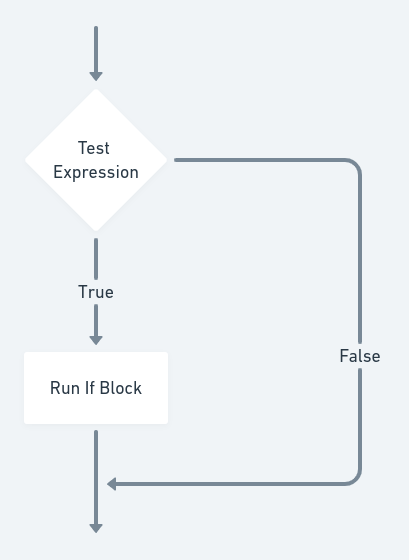
Demonstrating a Simple If Statement in Code
To better understand the concepts in the article, you will code for features that may be present in a barebones Weather App. The first feature will show a welcome message to the new users.
1. Open your code editor, create a new file, save the file to a folder of your preference with the name index.py.
2. Edit the index.py file and add the following code. As you only want to present a message to new users, this will be the single condition tested. The if condition evaluates the new_user
variable, and if true prints a welcome message to the console.
# Set the new_user variable to True, indicating that the user is visiting the application for the first time
new_user = True
if new_user:
print('Welcome to WeatherApp') # Prints a message to the new user
3. Run the index.py Python program by executing the following command in your terminal as shown in the following screenshot.
python3 index.py

Covering All Outcomes with the Else Block
In the previous section, the code you developed only acted on a single condition. In your coding adventures, there will be times when you also need to execute a set of statements in case the first if condition evaluates to false.
When the first statement evaluates to false, an else statement executes a different block of code as a fallback. In the below syntax, statement(s)_1
runs if the condition
is True
. If the condition
evaluates to False
, then statement(s)_2
runs.
if condition:
statement(s)_1
else:
statement(s)_2
To further illustrate this point, see the flowchart below.
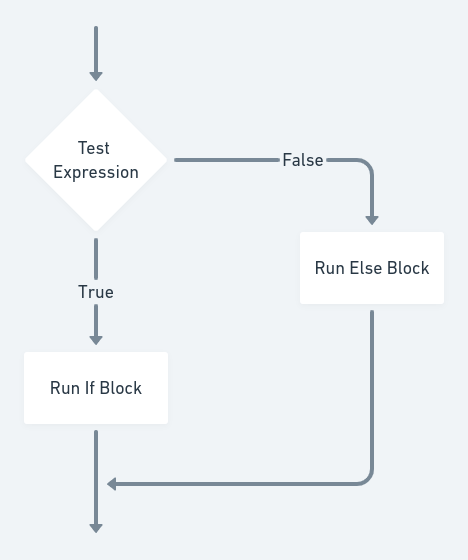
Demonstrating a Simple If-Else Statement in Code
Moving forward with the example of welcoming the user, you will now add a feature that will welcome old users back by displaying a welcome back message.
1. Open your code editor and the previously created index.py file.
2. While editing the index.py file update to the following code. Unlike before, here the new_user
variable is set to False
and triggering the else code block.
# Set the new_user variable to False, indicating that the user is visiting the application again and triggering the else clause
new_user = False
if new_user:
print('Welcome to WeatherApp') # Prints a message to the new user
else:
print('Welcome Back') # Prints a message to the returning user
3. Run the index.py Python program by executing the following command in your terminal as shown in the following screenshot.
python3 index.py

Testing Additional Conditions With Python If Else If Statements
There will be times in programming when you need to check for multiple conditions and execute the corresponding code blocks. To that end, Python has the elif
, else-if in plain English, keyword allowing additional conditional tests.
Python evaluates each condition in the order presented, starting with the initial if statement condition. When a condition evaluates as true, the corresponding code block runs, and all subsequent conditions are skipped. If no conditions evaluate as true, the else code block runs.
The syntax for if-elseif-else is as follows and as shown in the following flowchart.
if condition1:
statement(s)_1
elif condition2:
statement(s)_2
else:
statement(s)_3
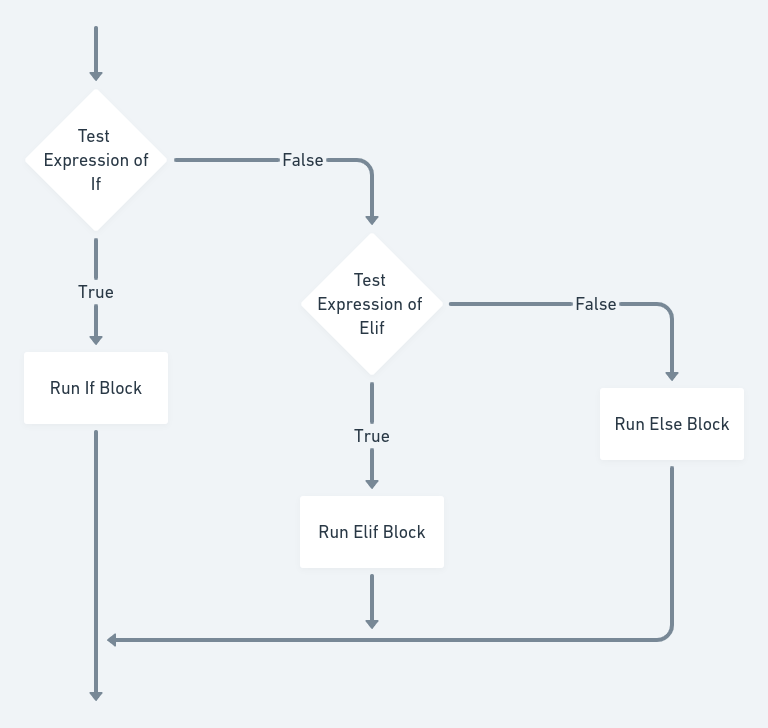
Demonstrating a If-ElseIf-Else Statements in Code
Back in the example Weather application, perhaps you want to add a feature to greet users with the text of “Good Morning”, “Good Afternoon”, or “Good Evening” determined by the time of day. In a real-world app, you would check the current hour of the day. To keep things simple, the code below assumes that the hour of the day is 15 (3 pm).
1. Open your code editor and the previously created index.py file.
2. Replace the existing code with the below code example. Here the hour
variable is set to the integer value of 15. The if statement first checks if the hour
variable is less than 12 and displays “Good Morning”, next if the hour
variable is less than 16 and displaying “Good Afternoon”, finally displaying “Good Evening” if the previous two conditions are false.
# Create and set a variable to the integer value of 15 corresponding to 3 pm.
hour = 15
if hour < 12:
print('Good Morning') # Display if the hour integer variable is less than 12
elif hour < 16:
print('Good Afternoon') # Display if the hour integer variable is less than 16
else:
print('Good Evening') # Display if the hour integer variable is not one of the above conditions
3. Run the index.py Python program by executing the following command in your terminal as shown in the following screenshot.
python3 index.py

Since the code assumes a fixed hour, the output always displays “Good Afternoon”.
Dealing with Complex Conditional Logic with Nesting
There will be times where you are working on a feature that requires complex conditional logic. In such cases, Python allows nesting of if-elseif-else statements.
To create nested if statements, add an additional if statement inside a code block for any outer if-elseif-else construct. You can nest as many if statements as you want as shown in the below example.
if condition1:
if condition2:
statement(s)_1
else:
statement(s)_2
elif condition3:
if condition4:
statement(s)_3
else:
statement(s)_4
Demonstrating Nested If Statements in Code
In your Weather app, you will implement a feature to help the user deal with rain conditions. If there is little chance of rain, the user will not need to take any action. If there is some chance of rain, you will advise the user to take precautions based on how high the chance of rain is: taking an umbrella when going out or taking an Uber.
1. Open your code editor and the previously created index.py file.
2. Replace the existing code with the below code example. The first if statement checks to see if the rain probability is greater than 0.2
or 20%. If the chance is greater than 20% evaluate the nested if statement to see if the rain probability is less than 0.5
or 50%. As the rain probably is 0.6
, the first if statement evaluates as true, and then the nested if statement evaluates as false ultimately displaying “Take an Uber” to the console.
# Declare the variable rain_probability with the float (decimal) value of 0.6 (60%)
rain_probability = 0.6
if rain_probability >= 0.2: # If the change of rain is greater than 20% (0.2)
if rain_probability < 0.5:
print('Take an Umbrella when going out') # Display if the chance of rain is less than 50% (0.5)
else:
print('Take an Uber') # Display if the chance of rain is greater than 50% (0.5)
else:
print('No rain') # Display if the chance of rain is less than 20% (0.2)
3. Run the index.py Python program by executing the following command in your terminal as shown in the following screenshot.
python3 index.py

Mixing Up If Conditions with the or
and and
Operators
Up to this point, your if-elseif-else statements tested a single conditional statement. Python logical operators, such as or
and and
, allow testing complex conditions. Logical operators combine two or more conditions and evaluate the entire condition as either true or false.
Demonstrating the or
Logical Operator in an If Statement
The first operator is the logical or
operator which evaluates a statement as true when at least one of the conditions is true.
1. Open your code editor and the previously created index.py file.
2. Replace the existing code with the below code example. The today
variable is set to Sunday
which the if statement evaluates whether the today
variable contains either Saturday
or Sunday
. If one of those conditions is true, display the text “Enjoy your Weekend”.
# Declare the today variable set to Sunday
today = 'Sunday'
# If the today variable is set to either Saturday or Sunday display the text
if today == 'Saturday' or today == 'Sunday':
print('Enjoy your Weekend')
3. Run the index.py Python program by executing the following command in your terminal as shown in the following screenshot.
python3 index.py

Demonstrating the and
Logical Operator in an If Statement
Now it is time for the second logical operator, and
, demonstrated below. The and
operator evaluates a statement as true when all of the conditions are true.
1. Open your code editor and the previously created index.py file.
2. Replace the existing code with the below code example. Here two variables are declared, user_type
and is_user_logged_in
. The example demonstrates displaying the text “You can access the premium features” if both variables evaluate as true.
# Declare the user_type variable and set to "pro"
user_type = 'pro'
# Declare the is_user_logged_in variable and set to True
is_user_logged_in = True
if is_user_logged_in and user_type == 'pro':
print('You can access the premium features') # Display if both the is_user_logged_in variable is set to true and the user_type is equal to "pro"
Run the index.py Python program by executing the following command in your terminal as shown in the following screenshot.
python3 index.py

The examples presented here were as simple as possible. Don’t be fooled as you can evaluate more than two conditions together. It is also possible to use
or
andand
in conjunction!
Conclusion
This tutorial taught you how Python if else if statements function, along with different logical operators. You learned these concepts by implementing examples that may come in handy as you write more complex applications. Ready to take it to the next level? Check Python loops and Flow control next!