Data is rarely perfect. Strings are used extensively in many data files. Often, scripts will need to modify string data to remove extraneous characters at the beginning or end of the content. Luckily, we have the PowerShell Trim()
method!
The PowerShell trim()
method makes trimming these characters easy with several methods for trimming strings, such as Powershell trim()
and Powershell trimend()
methods.
In this article, we explore the various techniques available to us and examples of how to get string data in just the format you need.
Related: Back to Basics: PowerShell Strings
Prerequisites
If you’d like to use the techniques in this tutorial, we’ll assume that you have PowerShell. The tutorial will be demonstrating these concepts with PowerShell 7 but all steps should work in Windows PowerShell and earlier versions of PowerShell Core as well.
Using the PowerShell Trim() Method
One of the most common ways to trim strings in PowerShell is by using the trim()
method. Like all of the other trimming methods in PowerShell, the trim()
method is a member of the System.String
.NET class. This method allows you to trim all whitespace from the front and end of strings or trim certain characters.
The
trim()
method is case sensitive!
Trimming Whitespace
To trim whitespace from strings, simply call the trim()
method with no arguments like below.
When the
PowerShell Trim
() method is not supplied with any parameters then theChar.IsWhiteSpace
method is called and all leading and ending whitespace characters found are removed.
# Expected to remove leading and trailing two spaces.
(" Test ").Trim()
# Expected to remove the leading two spaces and carriage return and newline characters.
(" Test`r`n").Trim()
# Expected to remove the leading two spaces and trailing Unicode Next Line character.
(" Test Test $([Char]0x0085)").Trim()
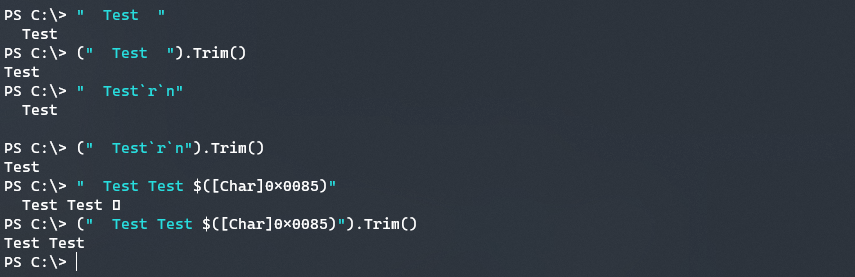
.NET Framework 3.5 SP1 and earlier used a different white-space character list than .NET Framework 4 and above. This means that in earlier .NET versions, the ZERO WIDTH SPACE (U+200B) and ZERO WIDTH NO-BREAK SPACE (U+FEFF) characters were removed whereas in later .NET versions they are not.
Additionally, in earlier versions of .NET the Trim() method does not remove the following three Unicode white-space characters, MONGOLIAN VOWEL SEPARATOR (U+180E), NARROW NO-BREAK SPACE (U+202F), and MEDIUM MATHEMATICAL SPACE (U+205F). These are removed in later versions of .NET
Trimming a Single Character
If you’d like to trim a single character from a string, you can do that with the trim
method. This method removes that character at the start and end of a string in all instances until it encounters a character different from the one supplied.
Take a look at the below example on trimming the character T
and t
.
# Expected to remove nothing as the leading character is a space and the ending is a lowercase "t".
(" Test Test").Trim("T")
# Expected to remove the leading "T" but not the ending lowercase "t".
("Test Test").Trim("T")
# Expected to remove nothing as the leading "T" is uppercase and no ending "t".
("Test String").Trim("t")
# Expected to remove both the leading and ending "t".
("test test").Trim("t")

Trimming an Array of Characters
Perhaps you have multiple characters you’d like to trim from a string. No problem. You can provide the PowerShell trim()
method with an array of characters.
Providing the trim()
method with an array of characters will remove all of those characters until it encounters one, not in that array from the beginning and end of a string object.
The trim()
method accepts an array of characters such as a simple string of characters such as abc123
or a proper array such as @("A","B","C","1","2","3")
.
Using an array with the trim() method can have some unexpected outcomes.
# Expected to remove the leading and trailing "ABC123" string.
("ABC123TestABC123TestABC123").Trim("ABC123")
# Expected to remove the leading and trailing "ABC123" string.
("ABC123TestABC123TestABC123").Trim(@("A","B","C","1","2","3"))
# Expected to remove the leading "A1B2C3" string and trailing "1A2B3C" string.
("A1B2C3Test123TestABC123TestABCTest1A2B3C").Trim("ABC123")
As you can see below, even when the characters are placed out of order, they are still removed as they fit one of the characters in the given array. If you were expecting the exact string to be removed from both the start and end of the string, this will not work using this method.

Using TrimEnd() and TrimStart()
If you’d rather not trim characters from both the start and end of a string, you can get choosier by using the more specific PowerShell TrimEnd()
and TrimStart()
methods. These methods mirror the PowerShell Trim() method
‘s functionality.
The TrimEnd() and TrimStart() methods are case sensitive!
Both TrimStart()
and TrimEnd()
give you more control over what section of a string object you are intending to work on. Depending on the circumstances, these methods may be more ideally suited to your data needs.
Take a look at some of the examples below.
# Expected to only remove the leading two spaces and maintaining the trailing carriage return and new-line characters.
(" Test`r`n").TrimStart()
# Expected to remove the two leading "a" characters and leaving the trailing "a" characters.
("aaTestaa").TrimStart("a")
# Expected to remove the leading "abc123" and leave the trailing "abc123" characters.
("abc123Testabc123").TrimStart("abc123")
# Expected to leave the leading two spaces and remove the trailing carriage return and new-line characters.
(" Test`r`n").TrimEnd()
# Expected to leave the leading "b" characters and remove the trailing "b" characters.
("bbTestbb").TrimEnd("b")
# Expected to leave the leading "abc123" characters and remove the trailing "abc123" characters.
("abc123Testabc123").TrimEnd("abc123")
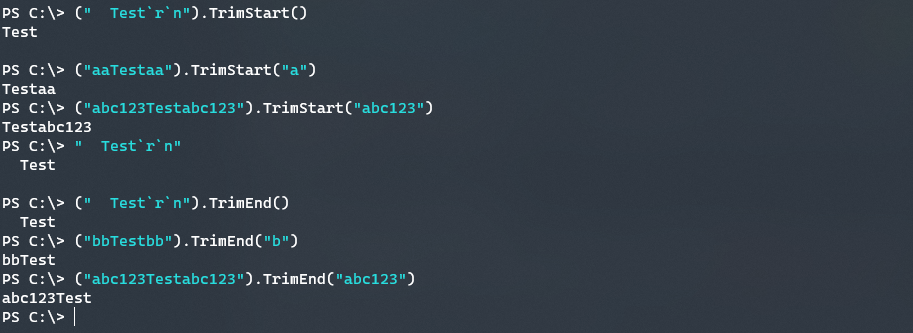
Additional PowerShell Trim Methods
Just like with everything in PowerShell, there are multiple ways to do just about anything; trimming strings is no different. Instead of using the PowerShell trim()
methods, you can use the SubString()
and IndexOf()
methods of a string object to remove a certain string.
Take a look at a comparison between the TrimEnd()
and using the SubString()
and IndexOf()
method below.
# Expected to remove "TeTest" instead of just "Test" at the end of the string.
("StringTeTest").TrimEnd("Test")
# Expected to remove only "Test" at the end of the string.
("StringTeTest").Substring(0,"StringTeTest".IndexOf("Test"))

The SubString method is a bit more cumbersome. By determining the index of the Test string from within the string object, and only returning the characters from the start of the string to that point, you are able to keep the remaining string content while only removing the defined string.
Conclusion
The PowerShell Trim()
, TrimStart()
and TrimEnd()
methods are powerful string utilities for cleaning up data as needed. If those three methods don’t meet your needs, PowerShell provides several different ways to do the same, with differing levels of control. Learn how to trim strings in PowerShell by applying these techniques today!