There are two types of quotes that can be used in PowerShell: single and double-quotes. In this article, you’re going to learn a bit about quotes and how to use PowerShell to escape double quotes.
Some critical differences between the two can make or break a script. Knowing these differences will make you a more effective PowerShell scriptwriter and help you avoid a rather simple mistake.
In this post, you will learn these differences and see examples of each scenario.
‘Single Quotes’
Single quotation strings are what you will most often use and encounter when creating or troubleshooting PowerShell scripts.
Consider the following example:
# Assign a variable with a literal value of 'single'.
$MyVar1 = 'single'
# Put the variable into another literal string value.
Write-Host -Message 'Fun with $MyVar1 quotes.'
Now examine the output:
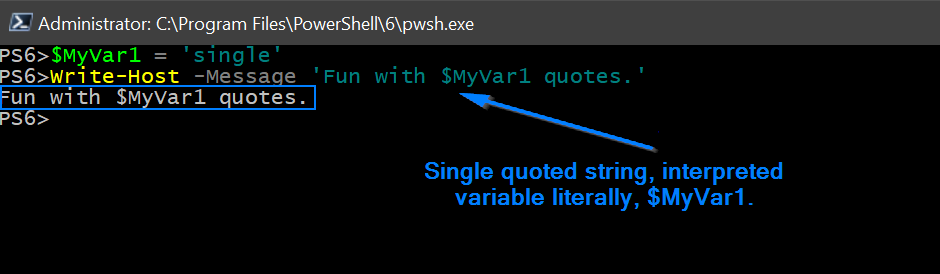
In the above case, PowerShell ignores $MyVar1
and treats the variable literally as $MyVar1
, exactly what was typed. There is no substitution here.
But how do you get PowerShell to recognize the variable value within a quoted string value? That’s where double quotation comes in.
“Double Quotes”
Double quotes gives you a dynamic element to string values. You will encounter this type of string quotation when the string contains dynamic data from variables stored in memory or dynamically generated.
Consider the following example:
# Same as previous example. Create a variable with a simple value.
$MyVar2 = 'double'
# Now to demonstrate double quotes magical power of interpretation!
Write-Host -Message "Fun with $MyVar2 quotes."
Now examine the output:
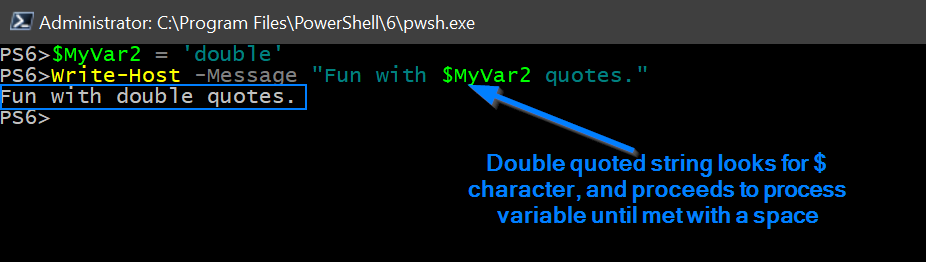
In the above case, PowerShell processes $MyVar2
because it was enclosed by a double-quoted string. Double quotes make PowerShell parse for text (the variable) preceded by a dollar sign and substitutes the variable name the corresponding value.
Real World Scenario
Now, apply this knowledge to a real scenario. Let’s say that you need to create a small function that will give an operator on your team some real basic information:
- Date / Time
- Disk % Used
- Disk % free
You need to return this information visually to an operator. Simple.
First, some pseudo code. We need to display the date time as today’s date and time. Think about how this string value will work. We can use Get-Date
cmdlet and the Uformat
parameter to give us the required date/time by using the correct patterns:
$date = Get-Date -UFormat "%m / %d / %Y:"
Testing the code in a PowerShell terminal confirms this works:
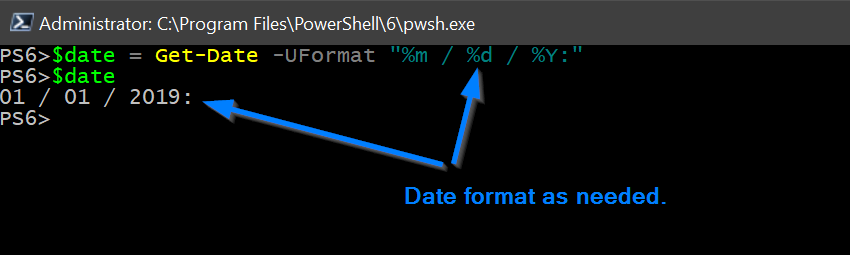
That takes care of the first part of the script. Now, I need to gather some disk information to also output to the terminal. The key metric I’m looking for is the percentage of free space remaining. I’ll display this information using Write-Host
again, but this time I’ll need to insert additional code inside the double-quoted string.
Remember, this information will be dynamic. For the purposes of this example, I’m going to create a variable, then utilize an available member type property to get the value I’m looking for:
$disk = Get-DiskSpace | Where-Object -Property Name -EQ 'C:\'
Testing the code in a PowerShell terminal confirms this works:
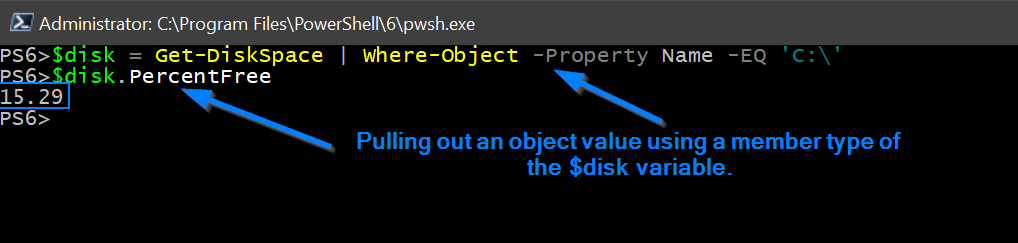
Perfect. We now have two variables that we can place in the strings that the operator will see when running this function. So let’s assemble the bits into the final script that will become our function:
function Get-CurrentDiskPercentageUsed {
$date = Get-Date -UFormat "%m / %d / %Y:"
$disk = Get-DiskSpace | Where-Object -Property Name -EQ 'C:\'
Write-Host "Storage report for $date"
Write-Host -ForegroundColor Yellow "There is $($disk.PercentFree)% total disk space remaining."
}
Testing again in a PowerShell terminal, here is what the operator would see:
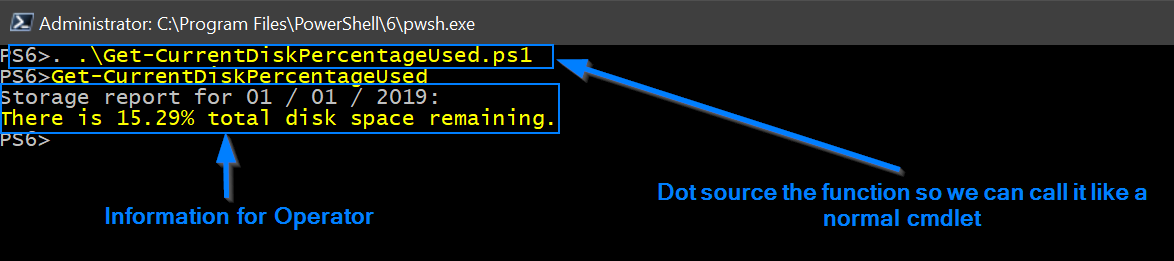
Notice what I did inside the last Write-Host
line with the $disk
variable. PowerShell evaluates the $( )
subexpression operator as an entire subexpression then replaces the result. Doing it this way also helps you avoid having to create more variables, which saves memory and can even make your script faster.
The function still needs some work. So let’s finish it off by adding some math to show a full calculation to the operator:
function Get-CurrentDiskPercentageUsed {
$date = Get-Date -UFormat "%m / %d / %Y:"
$disk = Get-DiskSpace | Where-Object -Property Name -EQ 'C:\'
Write-Host "Storage report for $date"
Write-Host -ForegroundColor Red "There is $(100 - $disk.PercentFree)% total disk utilization on drive $($disk.Name)."
Write-Host -ForegroundColor Yellow "There is $($disk.PercentFree)% total disk space remaining."
}
Results:
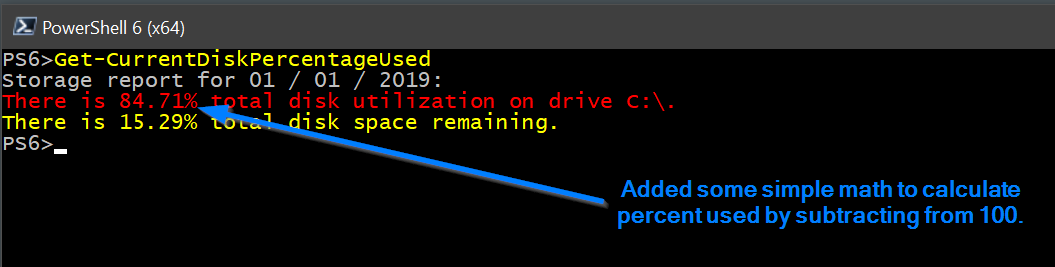
The operator can now make some faster decisions while supporting a remote system by using this function.
Using PowerShell to Escape Double Quotes
Now that you know all about how single and double quotes work in PowerShell, let’s cover a more advanced topic; escaping double quotes in strings.
Since you now know that double quotes expand variables inside of strings, what happens when you need to include literal double quotes inside of a string? In that case, you need to escape them or use single quotes.
Escaping is a term that refers to refers to making making non-literal elements literal. It’ll be much easier to understand with an example below.
Let’s say you need to create a string with double quotes inside of it like below. Notice that, as is, "string"
doesn’t actually include the double quotes.
PS51> "string"
string
To include the double quotes inside of the string, you have two options. You can either enclose your string in single quotes or escape the double quotes with a symbol called a backtick. You can see an example of both below of using PowerShell to escape double quotes.
Notice that "string"
now includes the double quotes.
PS51> '"string"'
"string"
PS51> "`"string`""
"string"
Summary
There’s not much to quotes in PowerShell. The one key concept to remember is that you need to know when to be literal ' '
, and when to be dynamic " "
. By default, you should always use single quotes unless there is a requirement for dynamic data in the string construct.
Additional Resources
To learn more about quotation rules, visit the about_Quoting_Rules PowerShell documentation from Microsoft or this excellent MSDN article. For even more examples of single/double quote usage, read Kevin Marquette’s “Everything you wanted to know about variable substitution in strings” .
You can also check out many of the other blog posts here on Adam the Automator on strings: