Working with cron jobs manually is most commonplace with low volumes of cron jobs. But what if you’re working with high volumes of cron jobs? PHP is the answer! Why PHP? Simply because it can automate the tasks needed to add, remove and list cron jobs.
Not a reader? Watch this related video tutorial!In this tutorial, you will learn how PHP can save time by automating adding, removing, and listing cron jobs for a Linux system.
Ready? Jump right in!
Prerequisites
This tutorial will be a hands-on demonstration. If you’d like to follow along, be sure you have the following:
- Crontab – Crontab is typically installed on Linux operating systems by default.
- Most Linux distributions utilize the same cron system. The examples are shown here on a macOS using a BSD-based underlying system.
Creating the Crontab File
Before you can manage cron jobs, you first need a file to store those cron jobs. All cron jobs are stored in one system-wide crontab file.
Open your terminal and run the command below to change directory to /usr/local
and create subdirectories (jobs
and scripts
). You can name these directories differently as you prefer. These directories will store your cron job files and scripts later.
cd /usr/local && sudo mkdir jobs scripts
Now run the command below to create the crontab file (crontab -e
). The crontab file is created automatically inside your ~/tmp directory the first time you invoke a crontab
command.
# Sets default text editor to nano
export EDITOR='nano'
# Initializes the crontab file and opens it in nano
crontab -e
Adding Single Cron Jobs
Now that you’ve created the crontab file, you can start managing your cron jobs. But you first need to focus on adding them via PHP. How? You’ll create and execute a PHP script to add cron jobs.
Cron jobs are scheduled with five parameters, as shown below. Each parameter has a default value of *, which indicates that no value was specified for that parameter, whether for hourly, daily, weekly, or monthly cron jobs.
<minute> <hour> <day(month)> <month> <day(week)> <command-line operation>
If you need a refresher on the cron job syntax with examples, take a look at crontab’s website.
1. Create a file in your text editor, copy/paste the code below and save it in the /usr/local/jobs directory. For this example, the file is named newjob.py, but you can name it differently. The code below prints a text (Executed!
) when executed.
print("Executed!")
2. Next, create a PHP script file, then copy/paste the code below to that PHP file, and save it in the /usr/local/scripts. For this tutorial, the script is named add.php.
Throughout the tutorial, bear in mind to save the script files in the /usr/local/scripts directory and the cron job files in the /usr/local/jobs directory.
The code below adds the newjob.py file as cron job in the crontab file.
<?php
// sort - Sorts the string in memory alphabetically.
// uniq - Removes any duplicate cron jobs from the string in memory.
// crontab - Writes the string in memory to the crontab file
shell_exec("(crontab -l ; echo '* * * * * python3 /usr/local/jobs/newjob.py') | sort | uniq | crontab");
?>
The
shell_exec
operation stores the list of cron jobs to a string in memory before adding a new one, so you won’t lose any of the existing cron jobs.
Does
shell_exec
not work? You may have this function disabled in your php.ini file. Look for thedisable_functions
configuration in your php.ini and removeshell_exec
from the list. Keep in mind that this can be abused, so be careful with this function!
3. Run the command below to execute the add.php
script from the /usr/local/scripts directory. php add.php
php add.php
Ensure you’re in the /usr/local/scripts directory when executing scripts throughout the tutorial.
4. Finally, run the below crontab -l
command to check if the new cron job exists.
crontab -l

Listing Cron Jobs
Perhaps you want to keep track of the cron jobs you’ve added, so you can either update or remove them. If so, your first step is to list the existing cron jobs. Listing cron jobs come in handy to keep track of the cron jobs you have.
Create a new PHP script, give it a unique name, and paste the code below to the script file. For this example, the script is named list.php.
The below code’s shell_exec
operation gathers and displays (echo()
) all cron jobs (crontab -l
) from the crontab file.
<?php
echo(shell_exec("crontab -l"));
?>
Now, run the command below to execute the list.php script.
php list.php

Listing an Array of Cron Jobs
Perhaps you want to print the list of cron jobs in an array instead of a plain list. If so, you’ll need the explode()
functions. These functions let you store cron jobs inside the crontab file as an element inside an array.
Replace the content of the list.php file with the code below. Executing the code below lists an array of cron jobs, and also prints the string length of the cron job.
<?php
// Lists existing cronjobs as string
$job_list = shell_exec("crontab -l");
// Store the list of existing cron jobs ($job_list),
// each in a new line ('\\n') to the $cron_jobs variable
$cron_jobs = explode("\\n", $job_list);
// Prints the list of array structured cron jobs ($cron_jobs)
var_dump($cron_jobs);
?>
Now run the command below to execute the list.php
script.
php list.php
As you see below, the code outputs the list of cron jobs in an array, while also showing the string length.
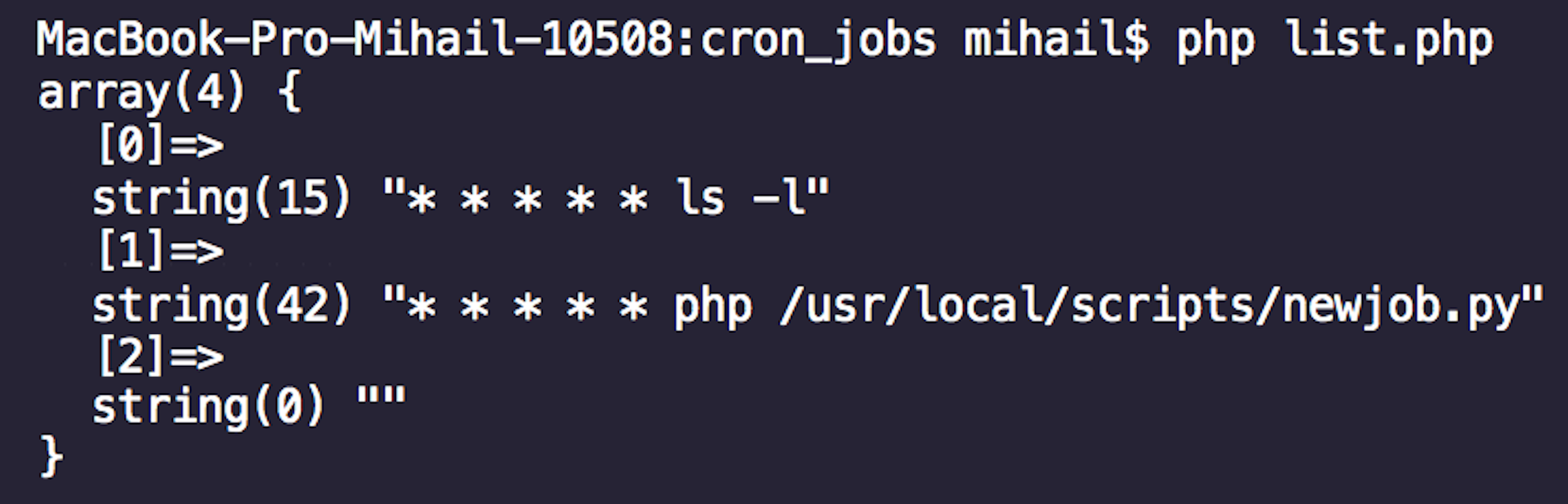
Removing Cron Jobs
Now that you have the list of existing cron jobs, you get to decide what you want to do with each cron job. Perhaps you have cron jobs that you want to remove. If so, you’ll specify the cron job to remove in a string variable.
1. Create a new PHP script called remove.php for this example, then add the code below to the script. You can name the script file differently as you prefer.
The below code removes a specific cron job from the crontab file.
<?php
# Stores the list of cron jobs as string to $jobs variable
$jobs = shell_exec("crontab -l");
# Defines the $to_remove string as the cron job to remove
$to_remove = "* * * * * php /usr/local/scripts/newjob.php";
# Removes the cron job ($to_remove) from the list of cron jobs ($jobs)
# and stores the new list of cron jobs inside the $removed string.
$removed = str_replace($to_remove,"",$jobs);
# Writes the $removed string value to the crontab file.
shell_exec("echo '$removed' | sort | uniq | crontab");
?>
2. Run the following command to execute the remove.php
script.
php remove.php
3. Finally, run the crontab -l
command to see if you’ve removed the newjob.php
cron job. crontab -l
crontab -l

Adding and Removing Multiple Cron Jobs
From adding a single cron job, you can also add multiple cron jobs, perhaps when you have daily tasks you want to automate. How? By adding a for loop in your script.
Adding and removing multiple cron jobs share a similar approach, but the script to remove multiple cron jobs has more use of variables, as demonstrated below.
1. Replace the content of your add.php file with the code below. Executing the code below adds multiple cron jobs to the crontab file by paddling through an array.
<?php
// Define cron jobs (three) as string separated by commas
$to_add = ["* * * * * php /usr/local/scripts/newjob.py", "* * * * * node /usr/local/scripts/newjob2.js", "* * * * * -ls"];
// Loops three times adding cron jobs to the crontab file
// since you defined three cron jobs as string
for ($i = 0; $i < 3; $i++) {
shell_exec("(crontab -l ; echo '".$to_add[$i]."') | sort | uniq | crontab");
}
?>
2. Next, rerun the command below to execute the add.php
script. php add.php
php add.php
3. Run the below crontab -l
command to verify if the cron jobs that you added in the crontab file exist. crontab -l
crontab -l

4. Now, replace the content of your remove.php file with the code below, which removes multiple cron jobs that you defined. Similar to adding multiple cron jobs, the same approach applies in removing multiple cron jobs, but with additional variables.
<?php
// Define cron jobs (three) as string separated by commas
$to_remove = ["* * * * * php /usr/local/scripts/newjob.py", "* * * * * node /usr/local/scripts/newjob2.js", "* * * * * -ls"];
// Executes the shell_exec command three times to remove cron jobs
for ($i = 0; $i < 3; $i++) {
// List existing cron jobs as string
$jobs = shell_exec("crontab -l");
// Collects cron jobs to remove from the existing cron jobs in an array
$removed = str_replace($to_remove[$i],"",$jobs);
// Removes cron jobs ($removed)
shell_exec("echo '$removed' | sort | uniq | crontab");
}
?>
5. Run the following command to execute the remove.php
script.
php remove.php
6. Finally, run the crontab -l
command to verify you’ve removed the cron jobs.
crontab -l
If you’ve successfully removed the cron jobs, you won’t get an output like the one below.

Creating Functions to Manage Cron Jobs
So far you’ve seen how can add, remove and list cron jobs in separate scripts. But to effectively manage cron jobs, you can create functions in a single script instead of running three separate scripts.
Create a new script file and paste the code below. Give the script any name you prefer, but for this example, the script is named multi.php.
The code below adds, removes, and lists cron jobs with three separate functions that you can call at the bottom of the script.
<?php
# Adds a crob job to the crontab file
function add_job(){
shell_exec("(crontab -l ; echo '* * * * * php /usr/local/scripts/newjob.py') | sort | uniq | crontab");
}
# Removes a crob job from the crontab file
function remove_job(){
$jobs = shell_exec("crontab -l");
$to_remove = "* * * * * php /usr/local/scripts/oldjob.php";
$removed = str_replace($to_remove,"",$jobs);
shell_exec("echo '$removed' | sort | uniq | crontab");
}
# Lists existing cron jobs
function list_jobs(){
echo(shell_exec("crontab -l"));
}
// CALL THE FUNCTIONS HERE
// Call the functions that adds and removes cron jobs but provide no output
add_job()
remove_job()
// Calls the function that outputs a list all exiting cron jobs
list_jobs()
?>
Now, run the command below to execute the multi.php
script.
php multi.php
Below, you can see the output of list_jobs()
function called in the multi.php script.

Preventing Memory Overload
You’ve seen PHP’s capabilities in managing cron jobs, but having too many cron jobs consumes memory. How to avoid memory overload? Set a count limit to how many cron jobs you can add to the crontab file.
1. Add the following function to the multi.php script. The function below counts all cron jobs by counting all lines inside the crontab file and prints a message to inform you of the current cron job count.
function count_jobs(){
$jobs = shell_exec("crontab -l");
# Counts all cron jobs from the crontab file and prints the result
echo("You currently have ".substr_count( $jobs, "\n")." cron jobs");
}
2. Next, call the count_jobs()
function at the end of the script, as you did in the “Creating Functions to Manage Cron Jobs” section.
3. Run the command below to execute the multi.php
script.
php multi.php
As you can see below, there’s a message that tells you the current cron jobs count.

But perhaps you want to automatically terminate the script if there are too many cron jobs running simultaneously. If so, adding an if
condition will do the trick.
4. Replace the count_jobs()
function in the multi.php file with the one below.
The function below reads the number of lines inside the crontab file, and based on that number, decides whether to terminate the script or not.
function count_jobs(){
$jobs = shell_exec("crontab -l");
# Counts all cron jobs from the crontab file and prints the result
echo("You currently have ".substr_count( $jobs, "\n")." cron jobs\n");
# Checks whether the cron jobs count is over 50 or not
if(substr_count( $jobs, "\n") > 50){
# Prints a message and terminates the script
exit("Too many cron jobs, cannot add any more!");
}
}
5. Finally, execute the multi.php
script as you did in step three. php multi.php
php multi.php
In the output below, you can see the message that tells you the current cron jobs count (63). Since the cron jobs count is more than 50, the script then terminates automatically and not proceed in adding cron jobs.

Conclusion
Throughout this tutorial, you’ve realized that PHP provides a way to automate cron jobs with the shell_exec()
function. You’ve learned how to add, remove and list cron jobs in a single PHP script while ensuring you don’t overload your memory.
Now, will you consider PHP the next time you need to manage cron jobs for your projects?