AppVeyor, a continuous integration solution for Windows and Linux is a great tool to build automated CI/CD pipelines. Using its built-in REST API with both the cloud and on-prem version, an AppVeyor API token, and a little PowerShell, we can build some cool automation around this!
This post is sponsored by AppVeyor. If you’re looking for a complete continuous integration platform capable of running both in the cloud and on-prem, I highly recommend checking them out.
AppVeyor is a CI/CD tool that allows developers to build automated pipelines for their software. I’m a PowerShell developer and marrying AppVeyor with PowerShell, to me, is a no-brainer.
I recently had the opportunity to start managing AppVeyor with their API and so far, it’s been straightforward. In this article, I’m going to cover how to get started working the AppVeyor API and PowerShell.
AppVeyor API Versions
As of 07/06/19, AppVeyor has two versions of their API in use v1 and v2. These two API versions can be differentiated by how you make API calls. You’ll see these differences below including some terminology you may see used in the documentation when referring to each API version.
Version | Endpoint Suffix | Label |
---|---|---|
1 | /api/ | Classic/Account-Level |
2 | /api/account/<account-name>/ | N/A |
Also, you’ll find that if you’re inspecting REST calls with Fiddler or similar HTTP inspection tool by navigating appveyor.com, you will see v2 references when the documentation is still v1. Watch out for this!
Related: Wrangling REST APIs and JSON with PowerShell
Prerequisites
To get started using PowerShell with AppVeyor, you’re going to need an AppVeyor account (appveyor.com). I use the free account because all of my projects are public but they also offer a paid account for private GitHub repos.
Once you have an account, you’ll then need to set up a project (appveyor.com) if you don’t have one already. In my case, I have everything in GitHub so that consists of creating an AppVeyor project that links to a GitHub repo.
Authenticating to the AppVeyor API with PowerShell
Assuming you’ve got a project created, you now need to find your AppVeyor API token. You’ll find this by clicking in the upper-right corner of the page and then going to My Profile. Once there, click on API keys on the left and then on the dropdown in the middle. This dropdown will display All accounts and <account-name>.
The All accounts options references the v2 API while the <account-name> option references the v1 API. The v2 option has complete control over all accounts while the v1 option only has control over a single account.
I will be using the v2 Appveyor API token in this tutorial but if you’re concerned about ensuring the utmost security, it would probably be better to use the v1 API key.
Ensure that the dropdown is set to All accounts.
You’ll then see the API key represented as the Bearer token as shown below. Copy that to your clipboard.
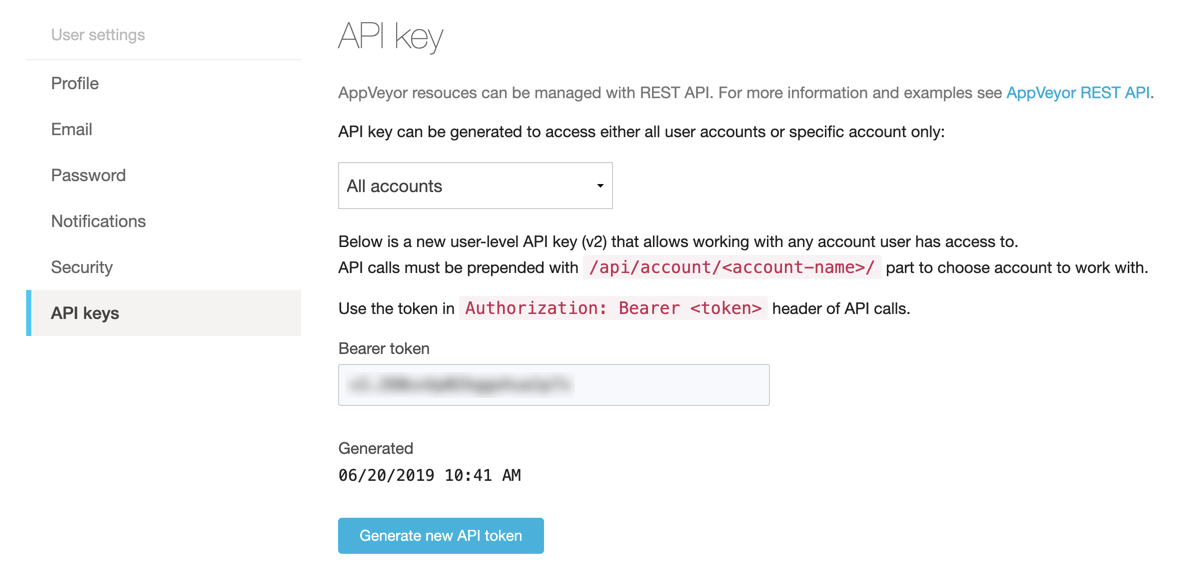
Next, you’ll need to drop down into your favorite PowerShell editor. I like to store all of the common variables I’ll be working with up top. Below, I have:
- My AppVeyor account name (
$accountName
) which may be different depending on if you’re using a cloud environment or on-prem. - The
$apiUrl
which represents the API endpoint all calls will point to. You can see that I have both the cloud and on-prem tokens defined as they will be different depending on which platform you use. - The Appveyor API token is copied from above (
$authToken
) with separate references for cloud and on-prem usage. - A PowerShell hashtable with the appropriate keys and values to pass to the API (
$authHeaders
)
## Cloud
# $cloudAccountName = 'adbertram'
# $apiUrl = "https://ci.appveyor.com/api/account/$cloudAccountName"
# $authToken = 'v2.XXXXXXXXXXX'
## On-prem
$onPremAccountName = 'AppVeyor'
$apiUrl = "http://localhost:8050/api/account/$onPremAccountName"
$authToken = 'v2.XXXXXXXXX'
$authHeaders = @{
"Authorization" = "Bearer $authToken"
"Content-type" = "application/json"
}
Note that I’m using the v2 API key here thus using the v1 API URL. If you are using the v1 API key, the value for
$apiUrl
will be simply be https://ci.appveyor.com/api.
Example PowerShell AppVeyor API Usage
Once you’ve got your token and all of your common variables set up, it’s time to query the API to see if we can get anything in return. I’ll use the Get Projects endpoint (appveyor.com) as a test. This endpoint returns all of the projects you have set up in your AppVeyor account.
To make an API call, use the Invoke-RestMethod
(microsoft.com) PowerShell cmdlet. Since you’ve already built the headers above ($authHeaders
), that’s already available. Next, you’ll need the URI to call.
Using $apiUrl
above, you can concatenate that base URI with the appropriate endpoint in this case, /projects
. This will give you the URI to query. And providing the headers hashtable just built will build a command reference to query all of the projects you have in your account.
$getProjectsUri = "$apiUrl/projects"
Invoke-RestMethod -Uri $getProjectsUri -Headers $authHeaders
Below is an example output you will receive.
projectId : 584065
accountId : 59292
accountName : <account-name>
builds : {}
name : PSADSync
slug : psadsync
repositoryType : gitHub
repositoryScm : git
repositoryName : <account-name>/PSADSync
repositoryBranch : master
isPrivate : False
isGitHubApp : False
skipBranchesWithoutAppveyorYml : False
enableSecureVariablesInPullRequests : False
enableSecureVariablesInPullRequestsFromSameRepo : False
enableDeploymentInPullRequests : False
saveBuildCacheInPullRequests : False
rollingBuilds : False
rollingBuildsDoNotCancelRunningBuilds : False
rollingBuildsOnlyForPullRequests : False
alwaysBuildClosedPullRequests : False
tags :
nuGetFeed : @{nuGetFeedId=0; id=psjotform-9cwmf027es01; name=Project PSJotForm; accountId=0; publishingEnabled=False;
created=0001-01-01T00:00:00+00:00}
securityDescriptor : @{accessRightDefinitions=System.Object[]; roleAces=System.Object[]}
disablePushWebhooks : False
disablePullRequestWebhooks : False
created : 2019-06-20T15:37:32.6192958+00:00
AppVeyor On-Prem
On May 5th, 2019, AppVeyor announced (appveyor.com) an on-prem version. You can download (appveyor.com) it and take a look at the Getting Started guide (appveyor.com) if you’re interested in getting it set up.
The download and set up was extremely easy mainly because it looks exactly like the cloud version. From the screenshot below, the only way you can tell this is from my on-prem installation is the URL
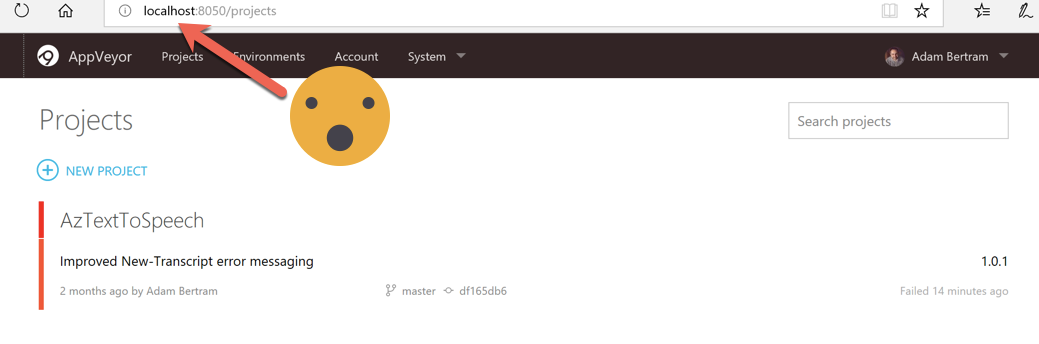
I played with the on-prem version a bit when I was learning the API and it is exactly the same too!
Code
Here’s the complete script used in this post.
## Cloud
# $cloudAccountName = 'adbertram'
# $apiUrl = "https://ci.appveyor.com/api/account/$cloudAccountName"
# $authToken = 'v2.XXXXXXXXX'
## On-prem
$onPremAccountName = 'AppVeyor'
$apiUrl = "http://localhost:8050/api/account/$onPremAccountName"
$authToken = 'v2.XXXXXXXXX'
$authHeaders = @{
"Authorization" = "Bearer $authToken"
"Content-type" = "application/json"
}
$getProjectsUri = "$apiUrl/projects"
Invoke-RestMethod -Uri $getProjectsUri -Headers $authHeaders
Summary
There are loads of other endpoints you can call to perform just about anything with the AppVeyor REST API both via the cloud and on-prem.
Using the techniques you’ve seen here will give you all of the knowledge you need to get started building PowerShell to take advantage of all the AppVeyor CI platform has to offer.