Those new to Visual Studio (VS) Code might just see a code editor. The true power of VS Code lies in it’s extensions, integrated terminal and other features. In this hands-on tutorial, you’re going to learn how to use Visual Studio Code by working with a Git repo.
Using built-in VS Code tooling and a few extensions, you’re going to write code and commit that code to source control using a single interface.
This blog post is a snippet of a chapter from the eBook From Admin to DevOps: The BS Way to DevOps in Azure. If you like this chapter and want to learn about doing the DevOps in Azure, check it out!
Tutorial Overview
In this tutorial, you’re going to learn how to use various VS Code features on Windows by building a project using Visual Studio Code and Git. You’ve been tasked with figuring out how to build an Azure VM with Terraform as a small proof of concept (POC) project. You have VS Code and have heard of its capability as a full IDE and want to put it to the test.
You’re going to:
- Create a VS Code workspace to share with your team
- Install the Terraform extension
- Modify the Terraform configuration file to fit your naming convention and Azure subscription
- Create a snippet for a common task you’ve found yourself typing over and over
- Commit the Terraform configuration file to a Git repo
This tutorial will not be meant to show how to use Terraform to deploy Azure VMs. We already have an article on Terraform and Azure VMs for that. This tutorial will focus on learning Visual Studio Code.
Does this sound like an interesting project? If so, read on to get started!
Prerequisites
To follow along with this Visual Studio Code Git tutorial, please be sure you have the following:
- VS Code – All examples will be using VS Code 1.44 although earlier versions will likely work as well.
- Terraform – All examples will be using Terraform for Windows v0.12.24.
- Git for Windows installed – All examples will be using v2.26. If you’d like VS Code to be Git’s default editor, be sure to select it upon installation.
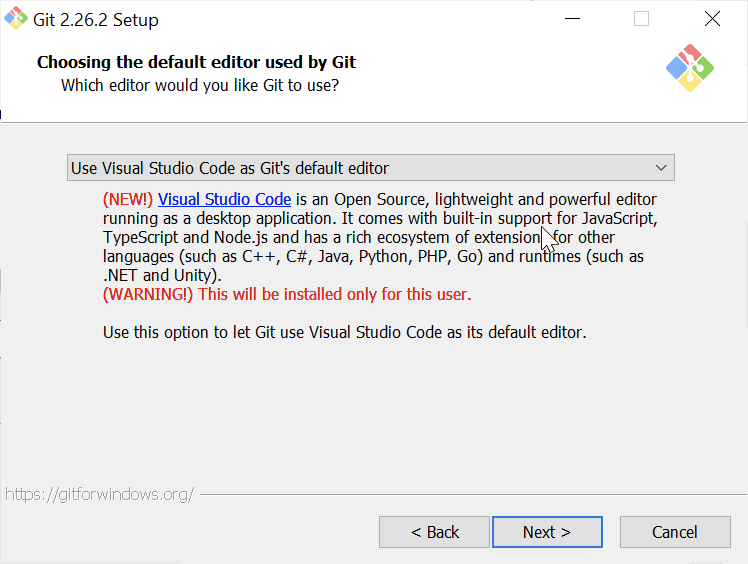
Clone the Git Repo
Since this tutorial is going to be focusing on working with code in a GitHub repo, your first task is cloning that GitHub repo to your local computer.
For this project, you’ll be working from a GitHub repo called VSCodeDemo. Since VS Code has native Git integration, you can clone a Git repo with no additional configuration. To do so:
- Open the command palette with Ctrl-Shift-P, type git where you will notice various options, as shown below.

2. Choose Git: Clone which VS Code will then prompt you for the repo’s URL. Here, provide the URL https://github.com/NoBSDevOps/VSCodeDemo.git and hit Enter.
3. Choose a folder to place the cloned project files. This project will place the repo folder in the root of C:\. Once you select the repository location, VS Code will invoke git.exe in the background and clone the repo to your computer.
4. When it’s finished, VS Code will prompt if you would like to open the cloned repository immediately as shown below, click Open to do so.

You now have an open folder in VS Code for the Git repo. You now need to “save” this open folder and all settings you’ll be performing in a workspace.
Creating a Workspace
Now that you have a folder opened containing a Git repo, save a workspace by going up to the File menu and clicking on Save Workspace As….
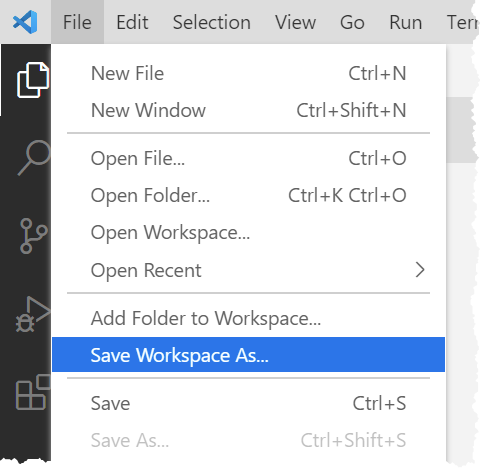
Save the workspace as project in the project folder. VS Code will then create a file called project.code-workspace in the Git repo folder. This workspace now knows what folder you had opened. Now when the workspace is opened in the future, it will automatically open the C:\VSCodeDemo folder.
Now, instead of a folder name, you will see the name of the workspace.
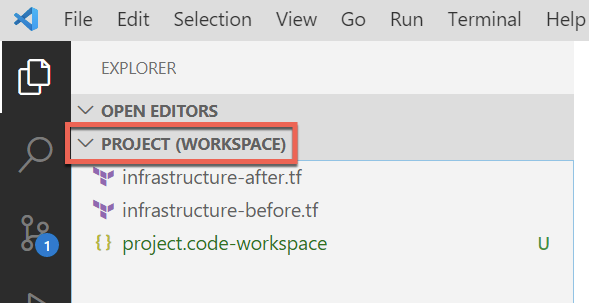
Setting up Extensions
Extensions are one of the most useful features of VS Code. Extensions allows you to bolt on functionality to help you manage many different projects. In this tutorial, you’re going to be working with Terraform.
Open up one of the Terraform configuration files in the workspace along the left sidebar. Notice how an editor tab opens up and shows the text but that’s about it. There’s no usual syntax highlighting or any other features. VS Code thinks this is a plain-text file and displays it accordingly. Let’s remedy that.
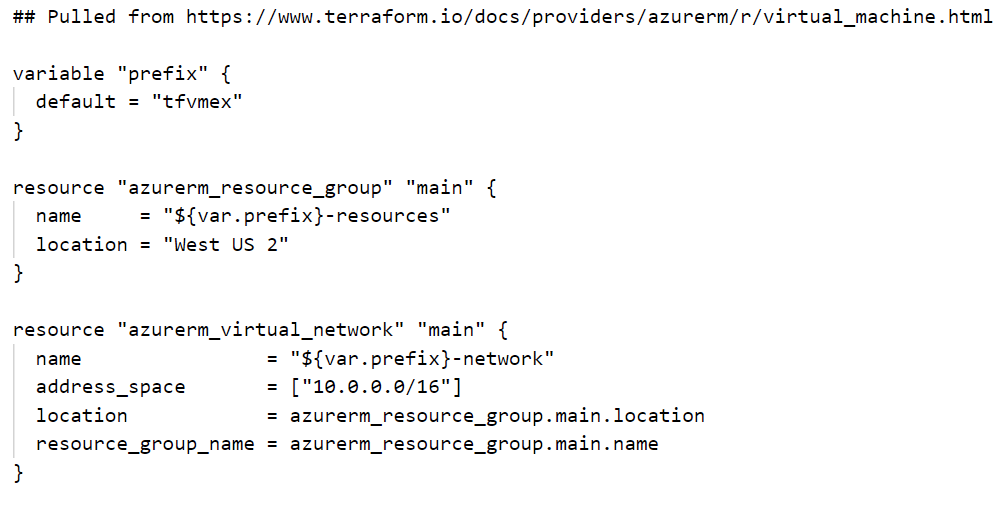
For VS Code to “understand” a Terraform configuration file, you need an extension. Extensions are a huge part of VS Code that opens up a world of new functionality. In this case, you need the Terraform extension to assist in building Terraform configuration files and deploying infrastructure with Terraform.
To install the Terraform extension, click on the extensions button on the Activity Bar and search for terraform. You’ll see multiple extensions show up but for this project, click on Install for the top result created by Mikael Olenfalk. VS Code will then install the extension.
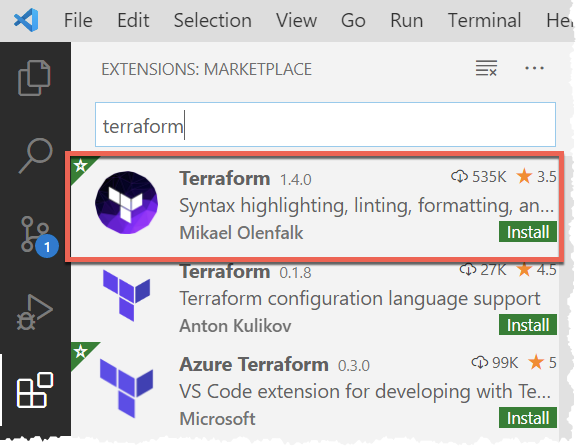
Once installed, navigate back to the workspace and click on one of the TF files in the workspace. You’ll immediately see one of the most obvious differences when using an extension, syntax coloring.
Now you can see in the following screenshot that VS Code “knows” what a comment is (by making it green), what a string is (by making it red) and so on. It’s now much easier to read a Terraform configuration file.
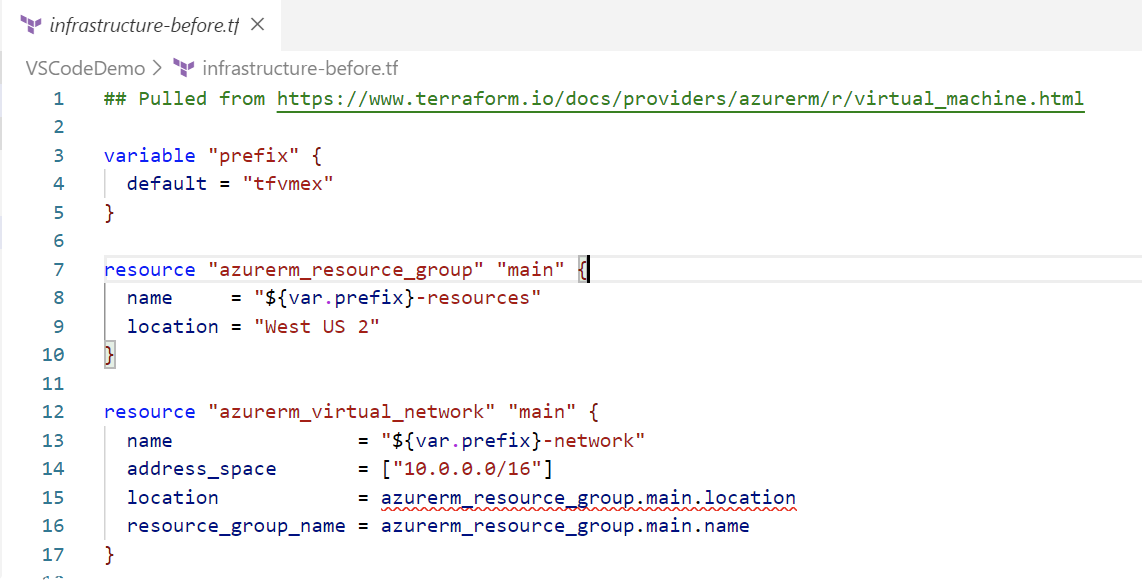
There’s a lot more functionality included with Mikael’s Terrafom extension. Be sure to investigate all of the potential benefits you can get from this extension if using Terraform.
Code Editing
Chances are when you find a script or configuration file on the Internet, it’s not going to be exactly how you need it. You’re going to need to modify it in some way.
In this tutorial’s example, you’d like to change the main
block label in the infrastructure-before.tf. Terraform configuration file to perhaps project
. To do that, you’ll need to find and replace some text. In VS Code, there are multiple ways to do that.
One of the most common ways to find a string and replace it with another is the good ol’ find and replace functionality.
Hit Ctrl-F and you’ll see a dialog similar to the following screenshot. Here you can type in the string you’d like to find and if you click on the down arrow, it will expand and provide a spot to input a string to replace it with. In the screenshot below, you can see options like Aa and Ab| for case-sensitive searching and also regular expressions.

You can also perform a “find and replace” using Ctrl-D. Simply select the text you’d like to find and begin hitting Ctrl-D. You’ll find that VS Code will begin to highlight each instance of that string with a blinking cursor.
When you’ve selected all items, start typing and VS Code changes all instances at once just as if you had selected each one individually.
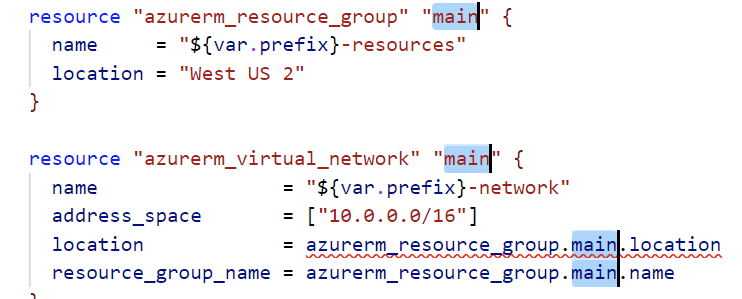
Saving Time with Snippets
Let’s say you’re really getting into Terraform and Azure and are tired of typing out the Terraform configuration file block to create a new Azure resource group in the following code snippet.
resource "azurerm_resource_group" "<element name>" {
name = "<resource group name>"
location = "<region>"
}
To save time creating these blocks, create a VS Code snippet.
To create a VS Code snippet:
- Copy the
azurerm_resource_group
block from the Infrastructure-before.tf Terraform configuration file.
2. Open the command palette with Ctrl-Shift-P.
3. Type “snippets” to filter the list of options.
4. Select Preferences: Configure User Snippets. This brings up a list of all the snippet files typically separated by language.
5. Type “terraform” to filter by the Terraform snippets.
6. Select terraform (Terraform) to open the Terraform snippets file (terraform.json).
With the Terraform snippets file open, remove all of the comments and copy/paste the following JSON element inside.
{
"Create Azure Resource Group Block": {
"prefix": "rg",
"body": [
"resource \"azurerm_resource_group\" \"${1:block label}\" {",
"\tname = \"${2:name}\"",
"\tlocation = \"${3:region}\"",
"}"
],
"description": "Creates a Terraform Azure Resource Group block"
}
}
Note the use of
\t
and the backslashes. You can’t directly place tab characters inside of a snippet. To represent a tab character, you must use\t
. Also, you must escape characters like double quotes, dollar signs, curly braces, and backslashes with a backslash.
8. Save the terraform.json file.
9. Go back to the Terraform configuration file and type “rg”. Notice now you see an option to expand a snippet.

10. Select the rg snippet as shown above. Notice that it now expands to the snippet you just created with three items highlighted.
VS Code highlighted each of the words to act as placeholders due to the variables defined in the terraform.json snippets file (${1:block label}
).
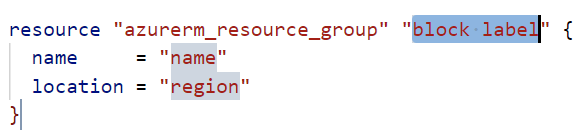
At this point, you can hit Tab and simply type in the values you need without worrying about how to create the block itself.
For a full breakdown on snippet syntax, be sure to check out the Snippets in Visual Studio Code documentation.
Commit Code to Git
At this point, you’ve cloned a public GitHub repo that contains a couple of Terraform configuration files. You’ve edited some files and now you’re ready to get those changes back up to the GitHub repo.
To get changes back up to the GitHub repo, you must first use Visual Studio Code and Git to commit changes to your local cloned Git repo. When you cloned the GitHub repo earlier, you downloaded not only the configuration files but also a Git repo.
If you’ve been following along, you should now have the cloned Git repo open with a couple of pending changes, two to be exact. How do you know that? By noticing the number in the Activity Bar, as shown below.
When you have a Git repo opened in Visual Studio Code, you’ll get a glimpse on the number of files that you can stage and commit into a local Git repo in the Activity Bar.
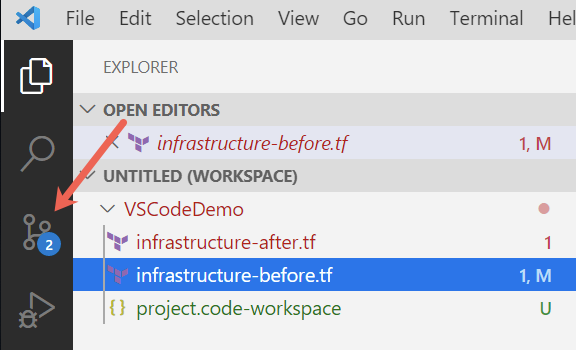
Click on the Source Control item on the left and you’ll see two items; the infrastructure-before.tf Terraform configuration file and the workspace you saved earlier (project.code-workspace). The configuration file will have a red M to the right indicating it’s been modified. The workspace file will have a green U to the right of it because it’s untracked meaning it’s currently not under source control.
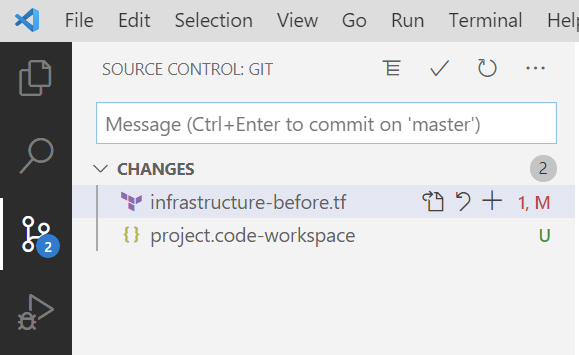
To ensure both of these files get back to the GitHub repo, first create a helpful commit message indicating why you’re committing these files. The message can be any descriptive summary. Once you’ve written a commit message, stage the changes. Staging changes in Visual Studio Code in Git adds the file contents to the staging area preparing for a commit to the repo.
While on the Source Control pane, click on the + icon beside each file to stage them as shown below.
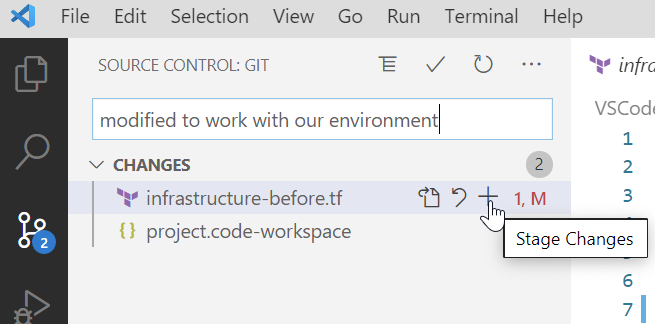
Once staged, click on the check mark to commit all of the staged changed, as shown below.
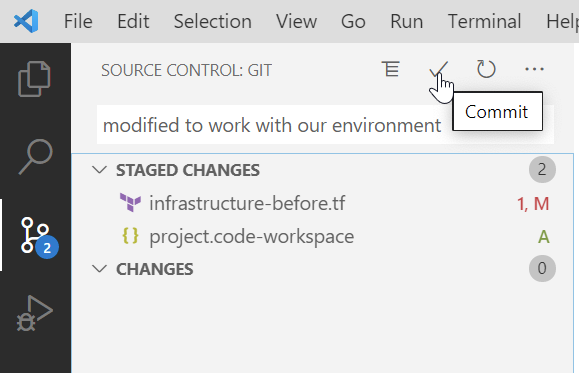
You will probably receive an error message indicating you need to configure a user.name and user.email in Git.
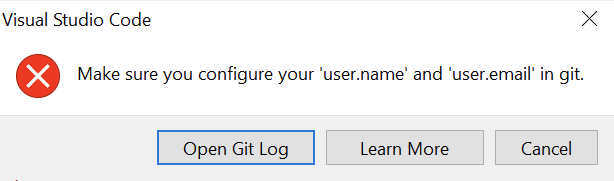
No problem. You simply need to provide Git the information it needs. To do that, go into your VS Code integrated terminal and run the following two commands changing my email address and name for yours.
git config --global user.email "[email protected]"
git config --global user.name "Adam Bertram"
Now try to commit the files. You should now see that the files commit to the repo.
You can stage all changed files without manually clicking on the + beside each file by committing them all at once. VS Code will automatically stage all of the files for you.
If you were working on a team with a shared repo, the next step would be to push these changes back to the GitHub repo or opening a pull request.
Conclusion
VS Code is a feature-rich IDE. It can not only help you write and understand code better, it can also build and make changes to infrastructure, invoke utilities and more. VS Code provides you one place to manage all of your development efforts.
Although this tutorial only covered a portion of what VS Code can do, this IDE is capable of so much more. If you’d like to learn about what VS Code can do, check out What You Need to Know about Visual Studio Code: A Tutorial.