As you dive deeper into PowerShell, you’ll encounter three core components essential for any PowerShell user: scriptblocks, arrays, and hashtables.
Scriptblocks allow you to encapsulate and execute reusable pieces of code; arrays let you organize and manipulate data collections. At the same time, hashtables provide a powerful way to store and access key/value pairs.
Whether you’re a beginner or looking to refine your skills, understanding these concepts will significantly enhance your ability to write effective PowerShell scripts.
Leveraging Scriptblocks for Reusable Code
When you run any command or code, that code is called an expression. It’s a finite bit of code that PowerShell executes.
For example, to check if a file exists, you can use the Test-Path
command:
Test-Path -Path C:\file.txt

This command is an expression. If you need to run this command in multiple places in your script, you can wrap it in curly braces to create a scriptblock.
$myScriptBlock = { Test-Path -Path C:\file.txt }
The scriptblock looks like a regular string.
$myScriptBlock

But when you append an ampersand (&
), PowerShell runs the code inside the scriptblock.
& $myScriptBlock
Scriptblocks allow you to store and execute code in a variable as needed, making them versatile and reusable. Scriptblocks are used in various areas in PowerShell and are an essential concept to understand.
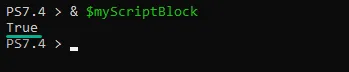
Harnessing the Power of Arrays and Generic Lists
Previously, we worked with single values like strings, numbers, or Boolean values. Let’s now explore collections of objects, starting with arrays.
Arrays serve as a basic data structure in PowerShell, facilitating the management of collections of related items. By using arrays, you can consolidate multiple values into a single variable, enhancing the organization and efficiency of data management.
Consider a color picker script that contains four colors: blue
, white
, yellow
, and black
.
$colorPicker = @('blue','white','yellow','black')
Working with Arrays
Arrays in PowerShell are indicated by the @
symbol, with elements separated by commas inside parentheses. You can read and manipulate these elements as a single set.
For instance, read the entire array.
$colorPicker
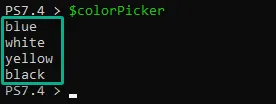
To read a specific element, reference its index starting from zero (0
).
$colorPicker[0]
$colorPicker[2]
$colorPicker[3]
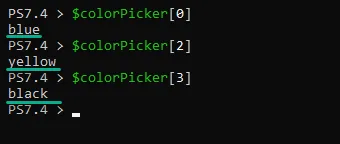
You can also use the range operator to read a sequence of elements.
$colorPicker[1..3]
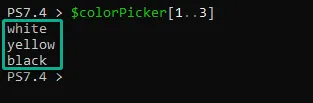
Elements in an array can be added, removed, or modified, just like a scalar value.
To change the first element (0
):
$colorPicker[0] = 'pink'
$colorPicker
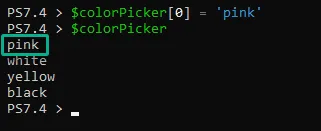
For adding (+
) a new element:
$colorPicker = $colorPicker + 'orange'
$colorPicker
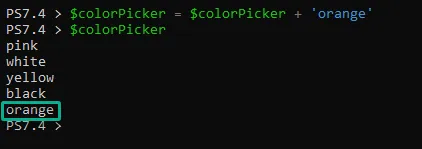
Or use a shortcut to add the element (+=
):
$colorPicker += 'brown'
$colorPicker
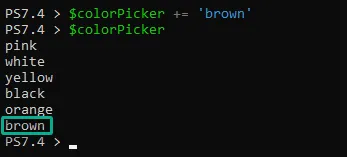
When adding multiple elements at once:
$colorPicker += @('pink','cyan')
$colorPicker
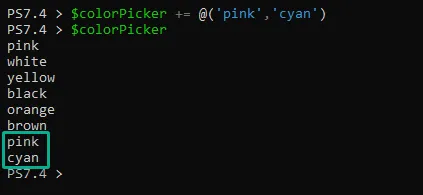
Managing Elements in Generic Lists
Besides arrays, you can use a different type of collection called a list or, more specifically, a generic list.
$colorPicker = [System.Collections.Generic.List[string]]@('blue','white','yellow','black')
$colorPicker
This code converts the array into a System.Collections.Generic.List
of strings.

With this list, you can add elements using the Add()
method.
$colorPicker.Add('gray')
$colorPicker
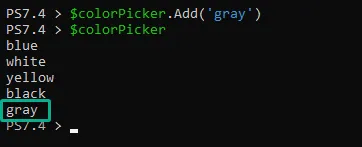
Or remove elements using the Remove()
method.
$colorPicker.Remove('gray')
$colorPicker
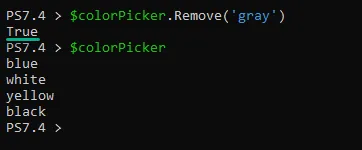
Streamlining Data Management with Hashtables
Another robust data structure in PowerShell is hashtables. While arrays are excellent for handling lists of items, hashtables offer a more advanced way to store and retrieve data using key/value pairs.
This capability makes hashtables ideal for tasks where you must quickly look up values based on unique identifiers.
Now, let’s explore hashtables as follows:
$users = @{
abertram = 'Adam Bertram';
raquelcer = 'Raquel Cerillo';
zheng21 = 'Justin Zheng'
}
$users
Unlike arrays, hashtables store a label or key that describes the value.
For example, this hashtable maps usernames to their full names.
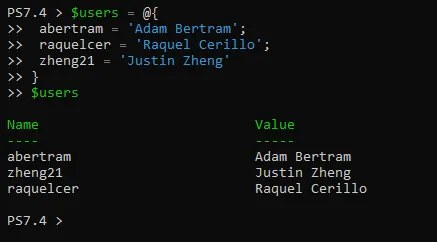
You can read elements using dot notation or brackets.
$users['abertram']
$users.abertram
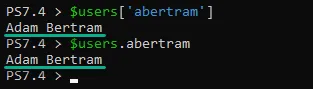
Next, to get a list of keys or values:
$users.Keys
$users.Values
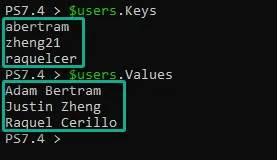
If you prefer to see items in a nicely formatted style:
Select-Object -InputObject $users -Property *
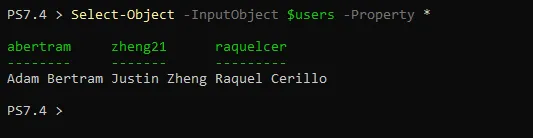
When adding elements to a hashtable, reference the key in brackets and assign a value.
$users['phrigo'] = 'Phil Rigo'
$users
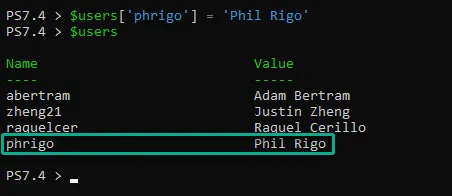
To check if a hashtable contains a particular key, use the ContainsKey()
method.
This method returns $true
if the key exists or $false
if not.
$users.ContainsKey('johnnyq')
$users.ContainsKey('phrigo')
$users
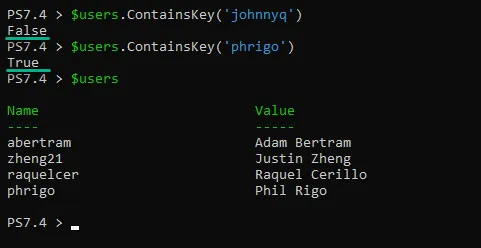
And finally, to remove an element, use the Remove()
method with the key.
$users.Remove('raquelcer')
$users
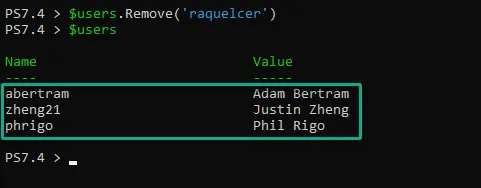
Conclusion
With an understanding of scriptblocks, arrays, and hashtables, you now have a versatile toolkit for managing data and reusable code in PowerShell. These core concepts make your scripts cleaner and more efficient and lay the foundation for tackling more advanced PowerShell tasks.
Continue to build on these skills, and you’ll find new ways to automate and streamline your workflows in PowerShell!