Inevitably a system administrator will need to restart a server or system. Rather than stepping through the user interface, why not use PowerShell to restart the computer (or lots of computers!)?
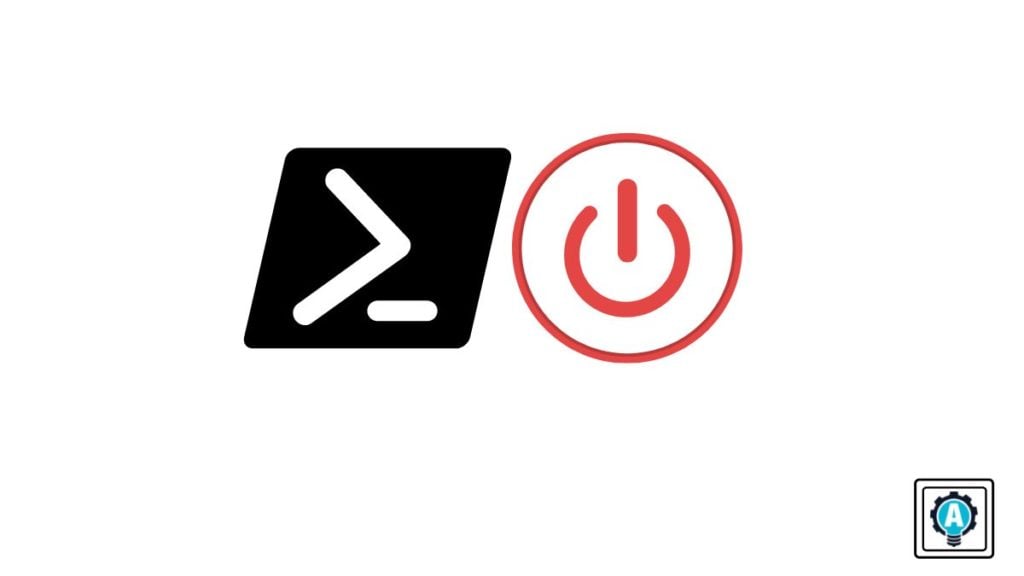
In this article, you’re going to learn all the ways to manage computer restarts with PowerShell. We’re going a lot deeper than just using the Restart-Computer
cmdlet.
Let’s go!
Prerequisites
This tutorial is going to be a guide taking you through various methods to use PowerShell to restart computers. If you intend to follow along, be sure you have the following:
- A user account on any computer (local or remote) in the local Adminstrators group
- Windows PowerShell or PowerShell Core. The tutorial will use Windows PowerShell 5.1.
- WinRM is configured and allowed through the remote computer’s Windows firewall and that WMI is allowed through the Windows firewall
Using PowerShell to Restart Computers with Restart-Computer
The first PowerShell specific method, and most common, is the PowerShell Restart-Computer
cmdlet. This cmdlet is simple to use with flexible parameters, some of which make script integration very easy.
As you can see in the example below, this is generally the most straightforward method and go-to solution for most PowerShell scripts.
The simple example below connects to a remote computer called SRV1. To skip the default confirmation, it uses the Force
parameter to restart the computer.
Restart-Computer -ComputerName SRV1 -Force
The Restart-Computer
cmdlet has a few parameters to configure how to command interacts with the computer as shown below.
ComputerName
– The system that you intend to restart. This parameter can take the following remote addresses, NetBIOS, IP Address, or the Fully Qualified Domain Name (FQDN). For the local system use.
,localhost
, or omit the parameter.Force
– Used if other users are currently on the system. This will force a shutdown.Wait
– This parameter will block the prompt and pipeline indefinitely (unless paired with the timeout parameter). This works in conjunction with theFor
parameter to poll for a specific component to become available.Timeout
– Used with theWait
parameter, this will ensure that the restart doesn’t block the prompt and pipeline indefinitely if there is a problem.For
– There are a few components that PowerShell can look for to indicate a successful restart. By default,Restart-Computer
looks to see if PowerShell itself is running to indicate a successful restart operation. Another option is to wait for WMI or WinRM to be available.Delay
– By default, the cmdlet will poll every5
seconds for the defined component to check while waiting for a remote system to become available. This parameter will override that default delay time period.
The
ComputerName
parameter does not use WinRM for the remote system call, therefore, you don’t need to worry about if the local system is configured for WinRM.
Using PowerShell to Restart Computers with Invoke-CimMethod
Not specifically intended for rebooting a system remotely, Invoke-CimMethod
works by using a WIM method to reboot the remote system. Not as flexible as the Restart-Computer
cmdlet, you can use PowerShell to restart computers remotely using a native command.
Ensure that WinRM is configured and allowed through the remote computer’s Windows firewall for this method.
Invoke-CimMethod -ComputerName SRV1 -ClassName 'Win32_OperatingSystem' -MethodName 'Reboot'
The Invoke-CimMethod
has a few parameters you should be aware of.
ClassName
– The name of CIM class to use. In the case of a restart command, we are using theWin32_OperatingSystem
class.ComputerName
– Using the WsMan protocol, you can use any of the following remote address types, NetBIOS, IP Address, or the Fully Qualified Domain Name (FQDN). If this parameter is omitted, then local operations are performed using COM.MethodName
– The method name is the WMI method for the class that is being targeted. In the case of a restart operation, you will need to use theReboot
method.
Using PowerShell to Restart Computers Remotely with Running shutdown.exe
Moving on from PowerShell-specific cmdlets, we come to the standard built-in executable that Windows offers to restart a system. The shutdown.exe
executable has been around a long time and offers a robust series of options.
Although not technically a PowerShell cmdlet, you can still use PowerShell to restart computers with shutdown.exe by invoking as an executable.
Ensure that the remote computer has the Remote Registry service enabled and WMI allowed through the Windows firewall for this method.
shutdown.exe /m \\remotecomputer /r /t 0
Below are the parameters for the shutdown command you should know.
r
– Restarts a computer after first shutting the system down.g
– This is similar to ther
command, but will also restart any registered applications upon startup. Introduced in Windows Vista, the Windows Restart Manager allows gracefully shutting down and restarting applications registered with this system. An example would be the Outlook application which is automatically started back up, if it was open initially upon shutdown.e
– Document the reason for an unexpected restart of the system.m
– The remote system to restart, takes the parameter of\\computername
.t
– The time in seconds before initiating the restart operation. If a value greater than0
is defined, thef
(force) parameter is implied. The default value is30
seconds, but no force.c
– An optional restart message that will appear on-screen before the shutdown and also in the Windows event log comment and can be up to 512 characters.f
– Force any running applications to close, which will not prompt for a File→Save prompt in any application and may cause data loss.d
– Listing of a reason code for the restart operation. An example of this type of reason code isP:2:18
which corresponds to Operating System: Security fix (Planned).
Using PowerShell to Restart Computers with PSExec.exe
Using PowerShell to restart computers is through one of the most used utilities within the Sysinternals toolkit, psexec.exe
offers several unique abilities that make interacting with a remote system easy. Taking a different approach than both PowerShell and built-in utilities, psexec.exe
creates a service on the remote system that commands are then proxied through.
Ensure the SMB Service is running, file and printer sharing is enabled, simple file sharing is disabled and the admin$ administrative share is available for this method.
psexec.exe -d -h \\remotecomputer "shutdown.exe /r /t 0 /f"
d
– Usepsexec
non-interactively by not waiting for the process to terminate, useful in scripts.h
– If the target system is Vista or higher, run this process using the account’s elevated token, if available.n
– Specifies a timeout in seconds while connecting to a remote computer.s
– Run the process using the system account, which confers a much higher level of access than the typical administrative account. This is not always needed, or used, but a very useful ability to have.computer
– A positional parameter that takes the form of\\remotecomputer
this allows usage ofpsexec
against a remote system.cmd
– Another positional parameter that is used to specify the actual command to run against the system. Sincepsexec
does not do the rebooting of the system itself, this command will be another utility such asshutdown.exe
used to restart a system.arguments
– This positional parameter contains any arguments that are needed to be passed to the previously definedcmd
.accepteula
– Ifpsexec
has not recorded that you have accepted the EULA, the tool would typically show an on-screen prompt to accept the EULA. This will accept that EULA without the graphical prompt.nobanner
– When connecting to a remote system, there is banner information displayed from the tool, whichnobanner
will suppress.
Bonus Methods!
The methods shown below are not very commonly used but could be useful depending on the needs.
RunDLL32.exe
The rundll32.exe
offers a way to run certain methods against internal executables and Windows APIs, such as shell32.dll. There are two methods you can restart a system using this functionality.
rundll32.exe user.exe ExitWindowsExec
– Restarts the local system.rundll32.exe shell32.dll,SHExitWindowsEx 2
– Will also restart the local system.
This method cannot be actually used remotely by itself, but you can combine this with PowerShell via an Invoke-Command
on a remote system.
# Method 1
Invoke-Command -ComputerName $ComputerName -ScriptBlock { & rundll32.exe user.exe ExitWindowsExec }
# Method 2
Invoke-Command -ComputerName $ComputerName -ScriptBlock { & rundll32.exe shell32.dll,SHExitWindowsEx 2 }
Taskkill.exe
Finally, taskkill.exe
is one other Windows utility that offers some functionality to restart computers, though in a roundabout way. By ending the lsass.exe
process, you will force a Windows restart.
taskkill.exe /S \\remotecomputer /IM lsass.exe /F
Using PowerShell to Restart Computers (Multiple Systems in Parallel)
Most system administrators will need to restart multiple systems at one point or another. Let’s take a quick look at how one might be able to tie these commands together to do this.
Using PowerShell to Restart Computers in parallel is easy using PowerShell 7 and the Restart-Computer
command.
$ComputerArray | ForEach-Object -Parallel {
Restart-Computer -ComputerName $_ -Force
} -ThrottleLimit 3
As you can tell with the newer ForEach-Object -Parallel
ability, it’s trivial to add parallelization onto your commands. Since Restart-Computer
supports remote restarting natively when combined with ForEach-Object
the ease of managing and restarting large systems can quickly be done!