Do you need to integrate external data from web services into your PowerShell scripts? In this practical tutorial, you’ll learn how to query REST APIs using PowerShell and parse the results without any fuss. We’ll build a reusable tool that handles errors gracefully and returns clean, structured data.
Let’s explore this by working with a free API from postcodes.io that provides UK postal code data.
The Basics: Making Your First API Call
The simplest way to query a REST API with PowerShell is using the Invoke-WebRequest
cmdlet. Think of it as a command-line web browser – it reaches out to any URL and downloads whatever content is available.
$result = Invoke-WebRequest -Uri 'http://api.postcodes.io/random/postcodes'
When you run this command, you’ll get back several properties, but the most important ones are:
- StatusCode – Should be 200 for a successful request
- Content – The actual data returned by the API
Let’s look at what’s in that Content property:
$result.Content

What you’ll see is JSON data containing postal code information. But there’s a catch – this content is just a string:
$result.Content.GetType().FullName
# Returns: System.String
To actually work with this data in PowerShell, you need to convert it from JSON to objects. Here’s how:
$objResult = $result.Content | ConvertFrom-Json
$objResult.result
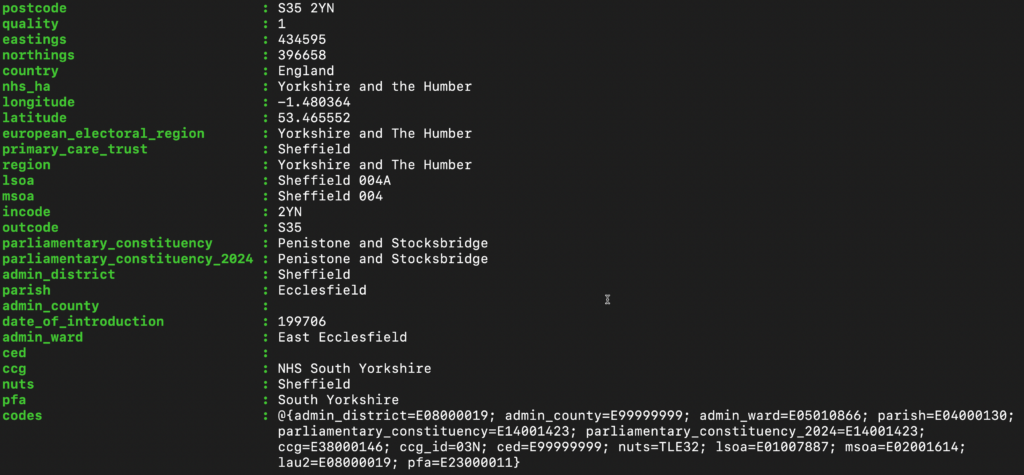
The Better Way: Using Invoke-RestMethod
While Invoke-WebRequest
works, PowerShell has a better cmdlet specifically designed for working with REST APIs: Invoke-RestMethod
. Here’s the magic – it automatically converts JSON responses into PowerShell objects:
$response = Invoke-RestMethod -Uri 'http://api.postcodes.io/random/postcodes'
$response.result
Much simpler, right? No manual JSON conversion needed!
Building a Reusable Tool
Let’s take this to the next level and build a reusable script. We’ll create a tool that can:
- Accept different API endpoints
- Handle errors gracefully
- Return nicely formatted results
Here’s our script (save it as Get-RandomPostCode.ps1):
[CmdletBinding()]
param(
[Parameter()]
[string]$Uri = 'http://api.postcodes.io/random/postcodes'
)
$response = Invoke-RestMethod -Uri $Uri -SkipHttpErrorCheck -StatusCodeVariable respStatus
if ($respStatus -ne 200) {
throw "API query failed: $_"
} else {
[PSCustomObject]@{
Postcode = $response.result.postcode
Country = $response.result.country
Region = $response.result.region
Latitude = $response.result.latitude
Longitude = $response.result.longitude
JSONFullResponse = $response
}
}
Let’s break down what this script does:
- Accepts a custom URI parameter with a default value
- Uses -SkipHttpErrorCheck and -StatusCodeVariable for better error handling
- Returns a clean custom object with just the properties we care about
- Includes the full JSON response if needed for troubleshooting
To use the script:
# Get a random postcode using the default API endpoint
.\Get-RandomPostCode.ps1
# Try a custom endpoint (this will demonstrate error handling)
.\Get-RandomPostCode.ps1 -Uri 'https://oops.postcodes.io'
A Note About Error Handling
In PowerShell 7+, we have some nice features for handling API errors. The -SkipHttpErrorCheck
parameter tells PowerShell not to throw errors into the error stream (avoiding that scary red text), while -StatusCodeVariable
lets us check the HTTP status code ourselves.
If you’re using an older version of PowerShell, you can use this alternative error handling approach:
$ErrorActionPreference = 'Stop'
try {
$response = Invoke-RestMethod -Uri $Uri
$response.result
} catch {
throw "API query failed: $_"
}
Pro Tips
- Always check the status code – just because an API responded doesn’t mean it worked
- Consider returning custom objects instead of raw API responses – it makes your scripts more professional and easier to work with
- Include the full API response in your output objects for troubleshooting
Next Steps
Now that you know how to work with REST APIs in PowerShell, you can:
- Integrate data from any web service into your scripts
- Build wrapper functions for your favorite APIs
- Create tools that combine data from multiple sources
The possibilities are endless! Just remember – always check the API’s documentation for authentication requirements and rate limits before building production tools.
What APIs are you planning to integrate with PowerShell? Let me know in the comments!