One feature that sets PowerShell apart from other languages is the pipeline. The pipeline is a way to “pipe” or send output from one command directly to another with no code in between.
This capability allows you to chain commands together in a single line, enabling the efficient execution of complex tasks.
Today’s sponsor is ScriptRunner, your #1 platform to accelerate your IT automation with PowerShell. They offer a FREE PDF cheat sheet, designed to be your go-to guide for the most important and frequently used Active Directory cmdlets. Download for free
Demonstrating the Pipeline
Before jumping into chaining commands together, it’s essential to understand the concept of the PowerShell pipeline. The pipeline enables seamless data flow between commands, allowing you to chain them together in a single line.
To demonstrate the pipeline, let’s start with a common command: Get-Service
.
Get-Service
Get-Service
returns all Windows services on your local system.
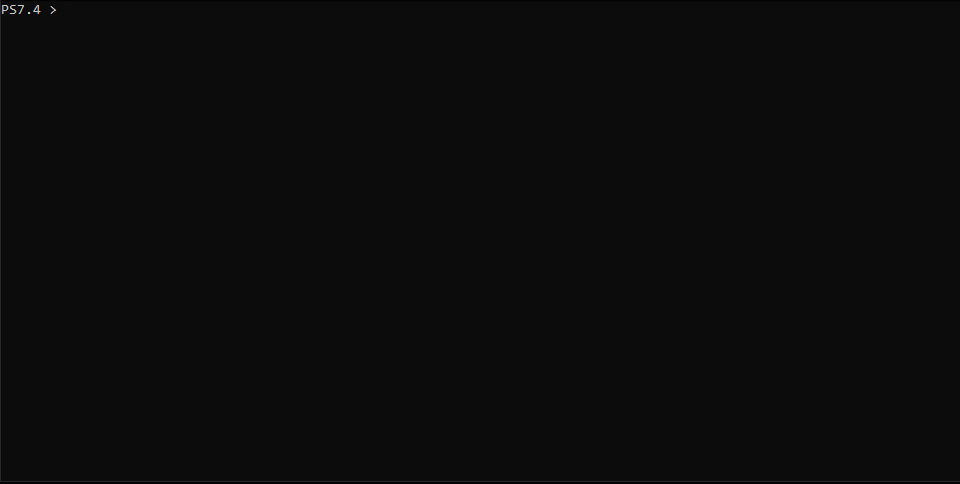
If you wish to check the status of the Windows Update service, you can use:
$serviceName = 'wuauserv'
Get-Service -Name $serviceName
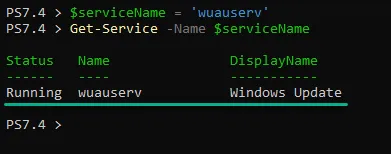
Now, to stop a service, execute the Stop-Service
command.
Stop-Service -Name $serviceName
This approach works, but there’s some duplication. Following the DRY (Don’t Repeat Yourself) principle is best practice when writing code.
Instead of defining the service name twice, you can streamline the process. In this case, Get-Service
queries the service and then pipes it to Stop-Service
.
Since Stop-Service
understands the output from Get-Service
, PowerShell handles the task seamlessly.
Get-Service -Name 'wuauserv' | Stop-Service
You can also pipe the service name directly to Get-Service
and bypass specifying the Name
parameter:
This example shows how efficient the pipeline can be.
'wuauserv' | Get-Service | Stop-Service
Imagine what would happen if you did this. PowerShell would attempt to stop all services on your machine, which is usually not the desired outcome.
Get-Service | Stop-Service
If you intend to find the status of multiple services listed in a text file, you can use Get-Content
to read the file and send the contents to the Get-Service
cmdlet:
Get-Content -Path C:\services.txt | Get-Service
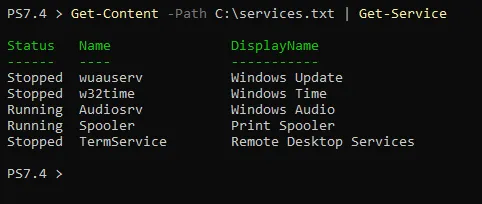
Checking Pipeline Support
Many commands in PowerShell support the pipeline, but not all of them, and knowing which ones do is crucial.
To check if a command supports pipe input, use Get-Help
with the Full
parameter:
Get-Help -Name Stop-Service -Full
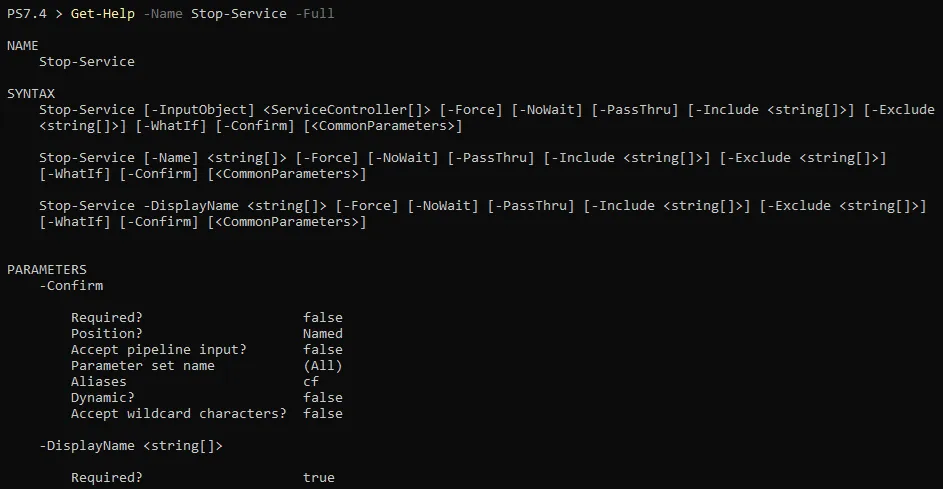
Scrolling down, you’ll see the InputObject
parameter, which accepts pipeline input by value.
This behavior signifies the parameter takes in the entire object from Get-Service
rather than just a property name.
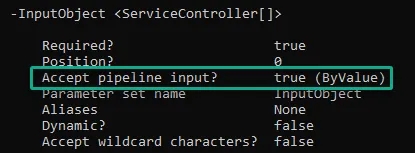
Below is an example of a command that does not support pipeline input:
'explorer' | Get-Process

While Get-Service
works as expected:
'wuauserv' | Get-Service
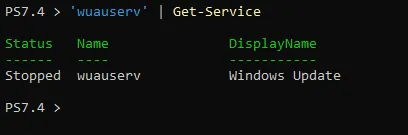
Conclusion
Through this tutorial, you’ve learned to chain commands together seamlessly, reducing redundancy and adhering to best practices like the DRY principle. You’ve seen how to combine pipelines with common cmdlets like Get-Service
, Stop-Service
, and Get-Content
to perform tasks more succinctly and effectively.
Understanding and leveraging the pipeline is fundamental for anyone looking to harness the full potential of PowerShell. By exploring the pipeline capabilities of various cmdlets using Get-Help
, you can continue to expand your PowerShell proficiency and streamline your workflows.
Embrace the PowerShell pipeline to make your scripting more efficient and powerful!