Ever wondered why PowerShell seems to magically know so much about your data? The secret lies in how PowerShell treats everything as an object. In this hands-on tutorial, you’ll learn how PowerShell objects work and how to harness their power for your automation needs.
Prerequisites
This tutorial assumes you have:
- Windows PowerShell 5.1 or PowerShell 7+ installed
- Basic familiarity with PowerShell commands
- A PowerShell console open and ready to follow along
Understanding PowerShell Objects
In PowerShell, everything you work with is an object – even simple text strings. This might sound complex, but it’s actually one of PowerShell’s superpowers. Let’s explore this with some practical examples.
Creating Your First Object
Fire up PowerShell and let’s start with something super simple – creating a string:
$color = 'red'
This looks basic, but there’s more than meets the eye. That simple string is actually a full-fledged object with properties and methods. Let’s peek under the hood with Select-Object.
Select-Object -InputObject $color -Property *
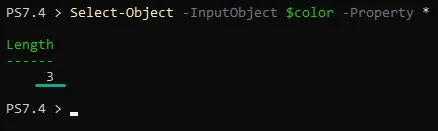
You’ll see output showing the string’s properties, including a Length
property. This is just the tip of the iceberg!
Working with Object Properties
Properties are like characteristics or attributes of an object. To access a property, you use what’s called dot notation:
$color.Length

This returns 3
– the number of characters in ‘red’. Simple, right? But wait, there’s more!
To see ALL the properties and methods available on your object, use the Get-Member
cmdlet:
Get-Member -InputObject $color
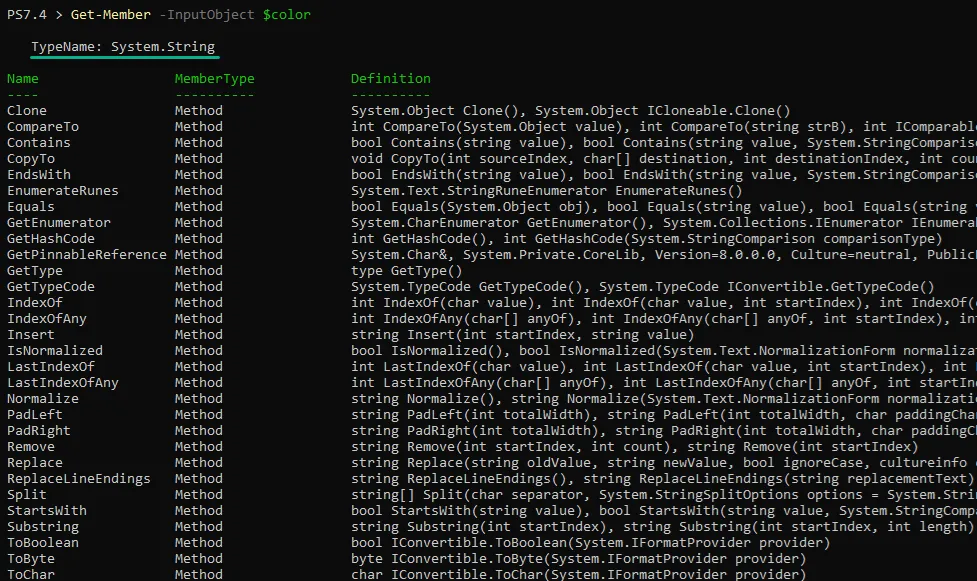
This command reveals the true nature of your string object – it’s actually a System.String
type with dozens of built-in capabilities!
Unleashing Object Methods
Methods are actions that objects can perform. Think of them as built-in functions. For example, let’s examine the Remove()
method:
Get-Member -InputObject $color -Name Remove
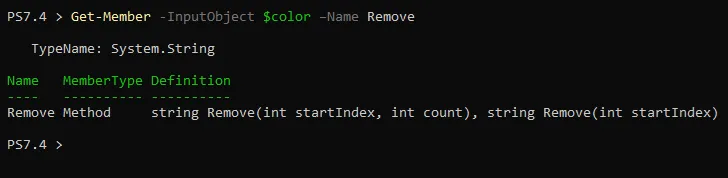
Methods often take parameters to customize their behavior. Let’s try removing the second character from our string:
$color.Remove(1,1)
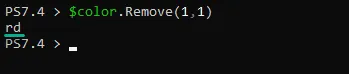
This returns rd
– PowerShell removed the ‘e’ from ‘red’. The numbers in parentheses (1,1) tell the method to start at position 1 (the second character, since PowerShell starts counting at 0) and remove 1 character.
PowerShell Objects in Action
Let’s put this knowledge to practical use with a more real-world example. When you run Get-Service
to check Windows services, you’re actually getting back a collection of objects:
Get-Service | Select-Object -First 1
Each service is an object with properties like Name, Status, and DisplayName. You can access these properties using the same dot notation:
(Get-Service | Select-Object -First 1).Status
This is powerful because it means you can easily:
- Filter services based on their properties
- Format the output exactly how you want
- Perform operations based on service attributes
Pro Tips
- Use tab completion after typing a dot to explore available properties and methods
- The `Get-Member` cmdlet is your best friend for discovering object capabilities
- Remember that almost everything in PowerShell is an object – files, processes, services, and more
- Properties describe an object, methods act on an object
Conclusion
Understanding PowerShell objects unlocks a whole new level of scripting power. Instead of just working with raw text and values, you get rich objects with built-in functionality. This makes your scripts more robust and often shorter since you can leverage the object’s built-in capabilities.
As you continue your PowerShell journey, keep exploring object properties and methods. They’re the building blocks for efficient and powerful automation.
Remember: In PowerShell, everything’s an object – and that’s a beautiful thing! 🚀