Apart from being a command line tool, PowerShell is a programming language from Microsoft that enables you to control and automate your systems. PowerShell uses a different syntax for its operators than most programming languages.
But, once you understand the PowerShell operator syntax, you will find that it is not difficult to use. One of the most common operators that you will use in PowerShell is the Not Equal operator (-ne
). This operator allows you to test whether two values are not equal to each other.
In this article, you will learn about the PowerShell Not Equal operator and how to use it with examples.
Prerequisites
This tutorial will be a hands-on demonstration. If you’d like to follow along, be sure you have a system with PowerShell installed. This tutorial uses Windows 10 and PowerShell 7.
Using the PowerShell Not Equal Operator (Basics)
In a gist, the PowerShell Not Equal operator (-ne
) determines whether the value of two objects is not equal and returns a Boolean value based on the result. If the values are not equal, the result is True
; otherwise, the result is False
.
But how and when do you use the PowerShell Not Equal operator? The succeeding sections will teach you the PowerShell Not Equal basics.
Comparing Variables
One of the most common uses for the PowerShell Not Equal operator is to compare two variables. For example, suppose you have two variables, $a
and $b
, and you want to see if they are equal. The comparison syntax is as follows:
$a -ne $b
If the values of $a
and $b
are not equal, then this comparison will return True
. Otherwise, it will return False.
1. Run the following commands in your PowerShell console to create the variables you’ll use as examples. The $a, $b, $c, and $d variables represent different values. You can compare each of these values to test whether or not they are equal.
You assign $c and $d the same value to see how the PowerShell Not Equal operator works with equal values.
$a = 1
$b = 2
$c = 3
$d = 3
2. Now, perform the PowerShell Not Equal comparison to see if the values of $a and $b are not equal.
$a -ne $b
The result returns True because the value of $a is 1, and $b is 2, which are not equal.
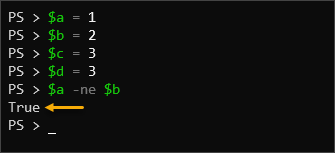
3. Now compare the $c and $d variables to see if they also are not equal.
$c -ne $d
You will see a False output return, as shown in the image below, since the values of $c and $d are equal (3=3).

Comparing Values
When working with PowerShell, not all objects you want to compare are in variables. In this case, you can directly compare values with the PowerShell Not Equal operator.
For example, the below command compares two values directly using the PowerShell Not Equal operator.
5 -ne 3
You will see a True value return, as shown below since the values aren’t equal.
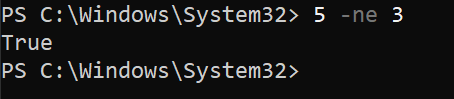
On the other hand, comparing equal values will return False
.
5 -ne 5
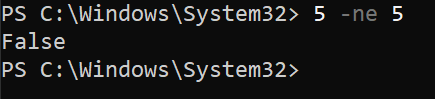
Removing a Value from the Array
The PowerShell Not Equal operator returns a Boolean result to indicate whether two values are not equal. Apart from that function, this operator can also filter out items from an array.
For example, create a variable called $numbers
containing an array of numbers 1 through 5.
# Create an array containing numbers 1 through 5.
$numbers = 1..5
# Display the array
$numbers
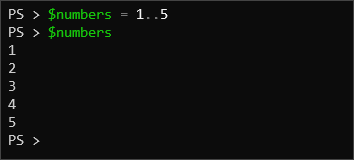
Next, run the command below to return the array except for one number (4
).
$numbers -ne 4
And now you can see that the command returned every number in the array except for the number 4.
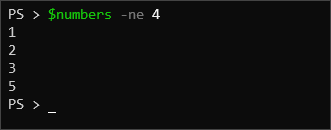
Comparing Strings
In addition to numbers, you can also apply the PowerShell Not Equal comparison on strings.
1. For example, suppose you have two strings, “Hello” and “World”. To determine if the two strings are not equal, run the below command.
"Hello" -ne "World"
The result is True since “Hello” is not equal to “World”.
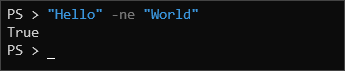
2. Now compare the strings “Hello” and “hello” to see if they are equal.
"Hello" -ne "hello"
You’ll then see the result is False because “Hello” is equal to “hello”.
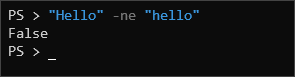
3. The PowerShell Not Equal operator is not case-sensitive. As you can see in the previous step, “Hello” is equal to “hello”. But if you require case sensitivity when comparing string values, substitute the -ne operator with -cne operator, which means Case Sensitive Not Equal.
"Hello" -cne "hello"
As a result, “Hello” and “hello” and no longer equal according to PowerShell.
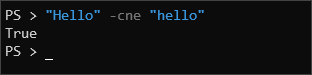
Using PowerShell Not Equal Operator in the Real World (Examples)
Now that you understand the basic concept of the PowerShell Not Equal operator, the following sections will let you experience some of its real-world uses.
Monitoring Services Status
A staple of Windows systems administration is monitoring the server service status. This task involves filtering out which services whose Status Is Not Running
.
For example, run the Get-Service
cmdlet to display all services on the local machine.
Get-Service
You can see all stopped and running services in the output below.
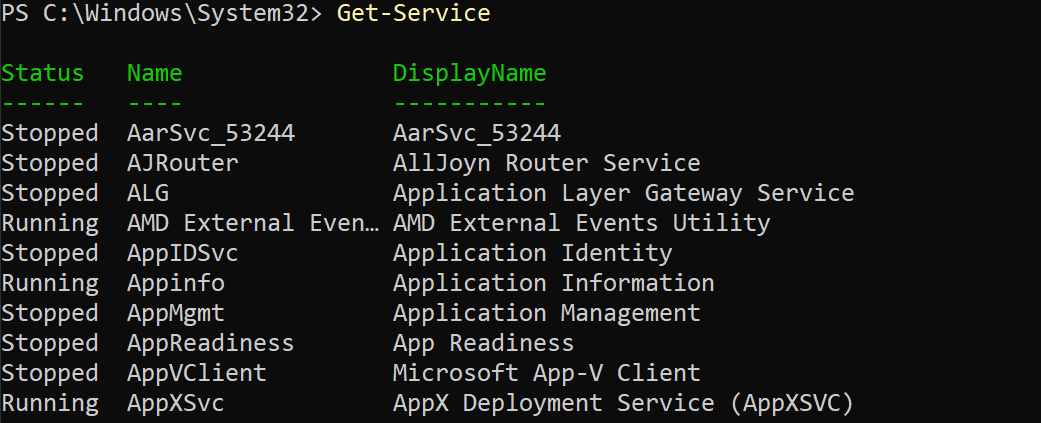
Now, to filter only those services that are not Running
, run the below command, and apply the -ne
operator as follows.
Get-Service | Where-Object {$_.Status -ne "Running"}
As you can see below, the Status column shows only the Stopped services after applying the PowerShell Not Equal comparison.
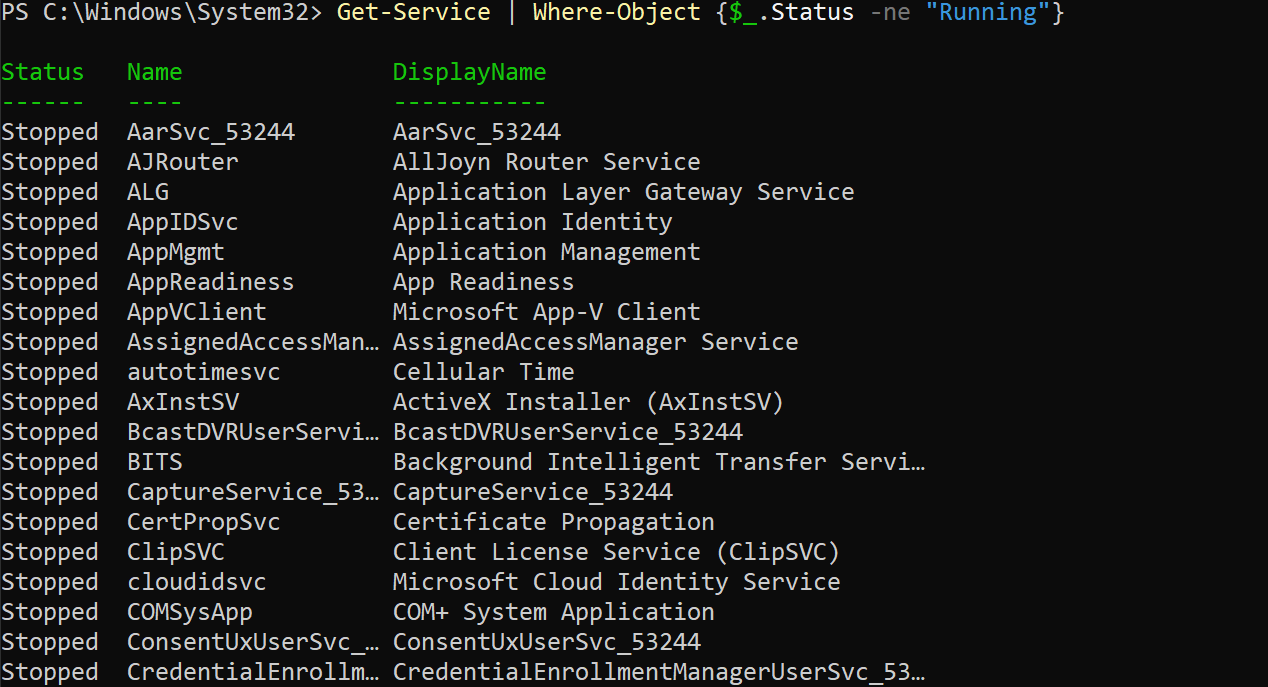
Likewise, run the below command to return the services whose Status Is Not Stopped
.
Get-Service | Where-Object {$_.Status -ne "Stopped"}
This time, you only see services that are running.
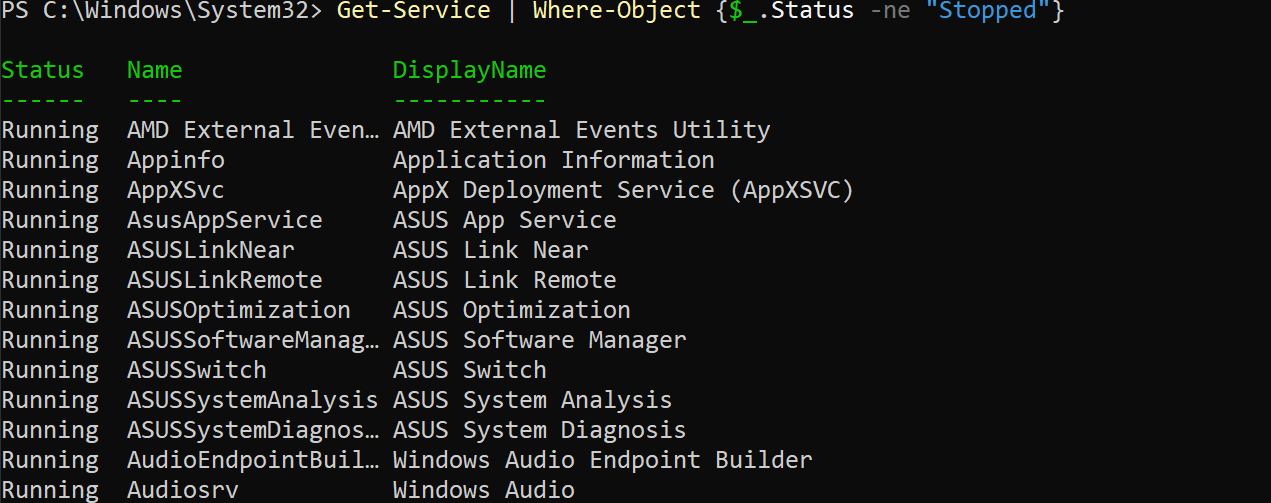
Combining PowerShell Not Equal with the AND
Operator
Apart from making a 1:1 comparison, you can combine the PowerShell Not Equal condition with another comparison operator, such as -eq
(equal), using the -and
operator. This method is useful when the object you are comparing must meet multiple conditions.
For example, monitoring server services typically involves ensuring that all Automatic services must be running. Run the command below to list all services whose Status Is Not Running
and whose StartType Is Automatic
. In this example, the object value must match all conditions.
Get-Service |
Where-Object {$_.Status -ne "Running" -and $_.StartType -eq "Automatic"} |
Select-Object Status,StartType,Name
Now you can see the automatic services that are not running, which can help you decide what actions you must take.
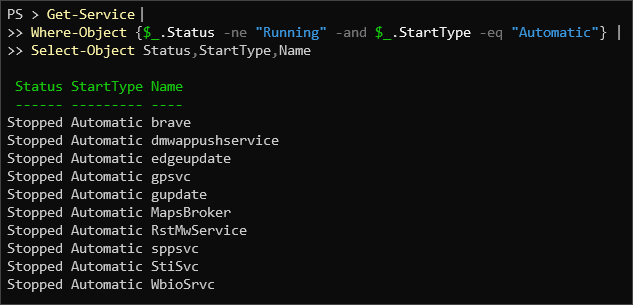
Adding If/Else Conditional Logic
You can also use the PowerShell Not Equal operator inside the conditional statements, such as the if/else statement.
For example, a service must be running in most cases if its StartType value is Automatic. The script below gets all the services, checks which automatic services are not running, and executes the corrective action(s)—to display a message and start the service.
Note: The Start-Service $_.Name line is commented out and will not run. Uncomment this line if you want to execute the command.
Copy the below code and run it in PowerShell.
Get-Service -ErrorAction SilentlyContinue | ForEach-Object {
if ($_.Status -ne 'Running' -and $_.StartType -eq 'Automatic') {
"The service [$($_.Name)] StartType is [$($_.StartType)] but its current Status is [$($_.Status)]"
# Start-Service $_.Name
}
}
The result shows you which automatic services are not running.
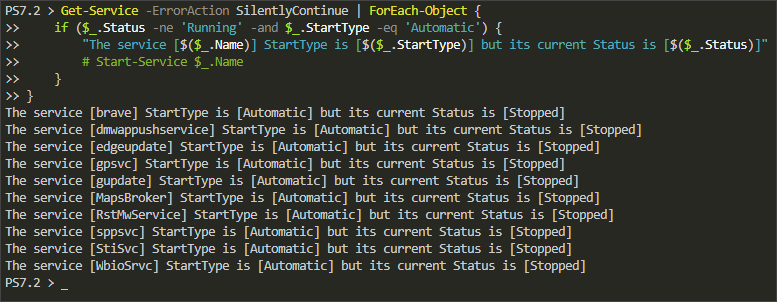
Conclusion
In this article, you learned about the PowerShell Not Equal operator. You also learned how to use it with examples. At this point, you should have a good understanding of how it works.
With this newfound knowledge, you can now use the Not Equal operator in your PowerShell scripts to test conditions. However, keep in mind that other comparison operators are also available. You might want to combine multiple operators to test more complex conditions.