Like many other programming languages, PowerShell has various comparison operators. However, unlike other languages, those comparison operators can look a little odd. Instead of ==
, you have eq
. Instead of <>
, you’ve got ne
. It can be confusing to newcomers. Let’s check out the PowerShell like operator and other comparison operators in this article.
Testing for Equality With eq
and ceq
Operators
To check to see if one object is equal to another object in PowerShell is done using the eq
operator. The eq
operator compares simple objects of many types such as strings, boolean values, integers and so on. When used, the eq
operator will either return a boolean True
or False
value depending on the result.
The -eq
operator needs two values to compare. Let’s say you assign a string to a variable called $string
. You’d like to compare this variable’s value to the string value "PowerShell"
to ensure they are equal.
In the following example, you can see that we’re assigning the value PowerShell
to the variable $string
. Then, using the eq
operator, the example is comparing the value of $string
with the string powershell
. Easy enough, right? But wait, they aren’t really the same though.
PS> $string = "PowerShell"
PS> $string -eq "powershell"
True
Note that
=
is an assignment operator and NOT a comparison operator. You cannot use=
to compare one value against another in PowerShell.
Testing Case-Sensitivity With the ceq
Operator
In the above example, notice how eq
returned a boolean True
value above even when the string wasn’t the exact same. This behavior happens because the eq
operator is case-insensitive. To test for case-sensitive equality, use the ceq
operator. The ceq
operator is the exact same as eq
with the exception of being case-sensitive.
You can see an example of using ceq
in the following code snippet. Now notice the PowerShell sees the case-sensitive difference.
PS> $string -ceq "PowerShell"
True
PS> $string -ceq "powershell"
False
Testing for Inequality With the ne
and cne
Operators
Just as eq
and ceq
test for equality, PowerShell has a pair of operators that do the exact opposite called ne
and cne
. Like their counterparts, these two operators perform the exact same operation yet opposite.
PS> $string = "PowerShell"
PS> $string -ne "PowerShell"
False
PS> $string -cne "PowerShell"
False
PS> $string -cne "powershell"
True
Testing for Items in a Collection
Typically, the eq
and ceq
operators are used for scalar or single values like strings, integers and boolean values. But these operators can also find instances of particular values contained within a collection like an array.
Notice in the example below that we’re creating an array with 12 integers. Perhaps you’d like to find all instances in that array that equal the number 9. No problem, use the eq
operator against the array to return all instances of the compared integer.
PS> $array =@(1,2,3,4,5,6,7,8,9,9,9,9)
PS> $array -eq 9
9
9
9
9
PS> ($array -eq 9).Count
4
Testing Collections to Contain Values
Since you just learned about using the eq
operator to find instances in arrays, let’s take that a step further and introduce the “containment” operators. These operators are contains
, notcontains
, in
and notin
. These operators return a boolean True
or False
value if a collection contains an instance or not.
Check out the following example. In this example, we’re creating an array with nine integers. If you want a simple Yes/No answer to if that array contains a certain integer, you can get that using contains
or notcontains
.
PS> $array = @(2,4,5,6,8,8,9,9,9)
PS> $array -contains 9
True
PS> $array -notcontains 9
False
PS> $array -contains 5
Similarly, you have the in
and notin
operators. These operators are nearly identical to contains
and notcontains
with one exception: the value to compare is on the left instead of the right.
$array = @(2,4,5,6,8,8,9,9,9)
9 -in $array
True
9 -notin $array
False
Testing “Greater than” With gt
and ge
Operators
What happens if you need to test whether a number (an integer) is greater than another number or perhaps greater than or equal to another number? You’d use the gt
and ge
operators. These operators compare integers.
Like the other operators, these operators return boolean True
or False
values depending on if one integer is greater than another. Both of these operators test whether the left integer is greater than or greater than or equal to the right integer.
In the below example, you can see how each of these operators behave.
PS> 7 -gt 5
True
PS> 7 -gt 7
False
PS> 7 -ge 7
True
Similar to the eq
operator that allows you to find instances in collections, you can do the same with the ge
and gt
operators too. PowerShell searches through each item in a collection and compares each value to the one you’ve provided. You can see a great example of this below.
PS> $array =@(1,2,3,4,5,6,7,8,9,9,9,9)
PS> $array -gt 5
6
7
8
9
9
9
9
PS> $array -ge 5
5
6
7
8
9
9
9
9
Testing “less than” With lt
and le
Operators
Similar to the gt
and ge
operators, the lt
and le
operators perform the same function yet opposite. The lt
and le
operators compare two values testing whether the integer on the left side is less than or less than or equal to the integer on the right.
You can see in the example below, the same functionality of comparing each value in a collection applies exactly the same as gt
and ge
.
PS> $array =@(1,2,3,4,5,6,7,8,9,10)
PS> $array -lt 5
1
2
3
4
PS> $array -le 5
1
2
3
4
5
Matching Based on Wildcards with the PowerShell Like Operator
So far, you’ve learned how to perform 1:1 matches. All of the operators used so far compared one whole value against another but PowerShell has another trick up it’s sleeve. You can also compare values based on wildcards or asterisks.
Let’s say you only know a few characters of a string. You can’t use eq
because eq
requires you to know the entire string. Using the PowerShell like operator, Â you don’t have to know the entire string. To test if a value is like another, you can replace the part you don’t know with a wildcard.
As with other operators, this same functionality can be applied to collections too.
You can see an example of PowerShell like and it’s case-sensitive brother, clike
below.
PS> $string = "PowerShell"
PS> $string -like "*Shell"
True
PS> $array = @("PowerShell","Microsoft")
PS> $array -like "*Shell"
PowerShell
PS> $array -clike "*Shell"
PowerShell
The operator -notlike
returns boolean True
if no match found and False
if there is a match. In case of using against a collection, it will return all other values that don’t match the pattern given on the right side of the -notlike
operator. Adding case sensitivity to the pattern, use -cnotlike
operator.
$string = "PowerShell"
$string -notlike "*Shell"
False
$array = @("PowerShell","Microsoft")
$array -notlike "*Shell"
Microsoft
$array -cnotlike "*shell"
PowerShell
Microsoft
Matching Based on Regular Expression
The PowerShell like operator and its relative are handy but as soon as you begin to require more complex matching. At that point, you can dive into the deep, dark world of regular expressions (regex). Using the match
and notmatch
operators, you can perform extremely complex string matching with regex.
Similar to the PowerShell like operator, match
and it’s opposite counterpart, notmatch
, compares two strings return a boolean True
or False
value. Also, like the other operators, the same behavior can be applied for a collection as the example below demonstrates.
PS> $string = "PowerShell"
PS> $string -match "ow"
True
PS> $array = @("PowerShell","Microsoft")
PS> $array -match "ow"
PowerShell
PS> $string = "PowerShell"
PS> $string -cmatch "po"
False
PS> $string -cmatch "Po"
True
PS> $array = @("PowerShell","Microsoft")
PS> $array -cmatch "Po"
PowerShell
Summary
In this article, you learned about the PowerShell like operator and many others and how to use each operator for single values and collections. You’ve seen how the output differs based on the operator and whether scalar or collection.
Additionally, you’ve also seen operators with case sensitivity, matching operators how to match based on regular expression or wildcard, and containment operators to test if the object contains a specific value or if a value is existing in a specific object.
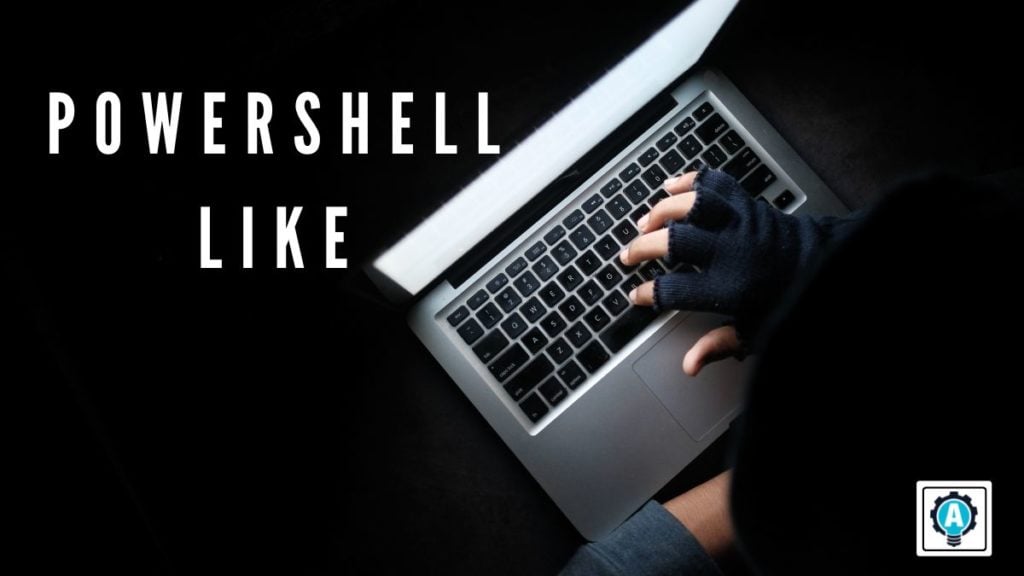