As a Windows systems administrator, working with JSON (JavaScript Object Notation) data is increasingly becoming a crucial skill. Whether you’re parsing configuration files, interacting with REST APIs, or managing application data, knowing how to handle JSON efficiently in PowerShell can save you hours of work. In this hands-on tutorial, I’ll show you the practical side of working with JSON in PowerShell.
Prerequisites
Before diving in, make sure you have:
- PowerShell 5.1 or higher installed (all examples use PowerShell 7)
- Basic familiarity with PowerShell cmdlets and pipelines
- A text editor of your choice (I’ll be using VS Code)
Reading JSON Files in PowerShell
Let’s start with a basic example. Say you have a JSON file called People.json
containing information about several people:
[
{
"Name": "John Smith",
"Age": 30,
"City": "New York"
},
{
"Name": "Jane Doe",
"Age": 25,
"City": "Los Angeles"
}
]
To read this JSON file into PowerShell, we use a combination of Get-Content
and ConvertFrom-Json
:
$people = Get-Content -Path ~\People.json | ConvertFrom-Json
$people
The magic happens in the ConvertFrom-Json
cmdlet – it transforms the raw JSON text into PowerShell objects that you can easily work with. When you run this command, PowerShell displays the data in a structured format:
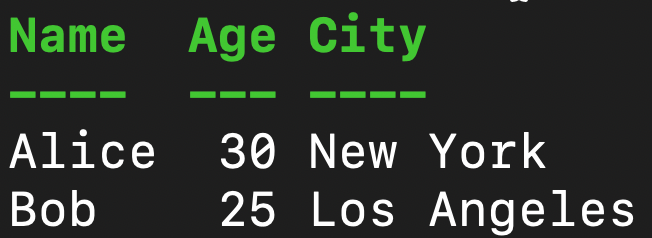
Manipulating JSON Data
One of the best parts about converting JSON to PowerShell objects is that you can manipulate the data just like any other PowerShell object. Here’s how to modify values:
# Change the city of the first person
$people[0].City = 'Chicago'
# View the changed object
$people[0]
The real power comes when you need to filter or transform the data. For example, to find everyone over 25 years old:
$people | Where-Object { $_.Age -gt 25 }
This is MUCH simpler than trying to parse the JSON directly with regular expressions, which would look something like this monstrosity:
$regexPattern = '({[^}]"Age"\s:\s(?:[2-9][6-9]|[3-9][0-9]|[1-9][0-9]{2,})[^}]})'
$matches = [regex]::Matches($jsonFromFile, $regexPattern)
Trust me, you don’t want to go down that path unless absolutely necessary!
Saving Modified JSON Data
After making changes to your data, you’ll probably want to save it back to a file. Here’s how to convert PowerShell objects back to JSON:
$people | ConvertTo-Json | Set-Content -Path ~\UpdatedPeople.json
This pipeline:
- Takes your PowerShell objects ($people)
- Converts them back to JSON format (ConvertTo-Json)
- Saves the JSON to a new file (Set-Content)
To verify the changes, you can read the new file:
Get-Content -Path ~\UpdatedPeople.json
Common Pitfalls to Avoid
- Encoding Issues: If you see strange characters in your JSON, make sure you’re using the correct encoding when reading/writing files
- Depth Limitations: By default, `ConvertTo-Json` only converts 2 levels deep. Use the `-Depth` parameter for deeper objects
- Special Characters: Watch out for special characters in JSON strings – they need proper escaping
Conclusion
Working with JSON in PowerShell doesn’t have to be complicated. By leveraging built-in cmdlets like ConvertFrom-Json
and ConvertTo-Json
, you can easily read, manipulate, and write JSON data without wrestling with complex regular expressions or string manipulation.
The next time you need to work with JSON data in your PowerShell scripts, remember this simple workflow:
- Read the JSON file with Get-Content
- Convert it to PowerShell objects with ConvertFrom-Json
- Manipulate the data using standard PowerShell commands
- Save the changes using ConvertTo-Json and Set-Content
Have you found other useful techniques for working with JSON in PowerShell? Share your experiences in the comments below!