If you’re using PowerShell to get free disk space on a Windows computer, you’ve come to the right place. In this tutorial, you will learn how to use PowerShell to get free disk space and monitor disk usage.
Prerequisites
This tutorial will assume you’re on a Windows 10 or greater computer with Windows PowerShell v5.1 installed. PowerShell 6+ will most likely work fine also. It is also assumed that you’re in an Active Directory environment. If not, be sure to learn about the Credential
property on the New-CimSession
cmdlet.
Querying CIM
Every piece of Windows system information is located in CIM/WMI. The WMI datastore has information on thousands of Windows systems; disk and storage information is just one.
The CIM datastore is broken down into various classes. WMI stores information about disks in the Win32_LogicalDisk class. The Win32_LogicalDisk class contains disk information such as disk name, total size, free space, etc. To query this class with PowerShell, you must create a CIM instance with the Get-CimInstance
cmdlet.
Using Get-CimInstance
only specifying the ClassName
parameter is the easiest way to query the class.
Get-CimInstance -ClassName Win32_LogicalDisk
Get-CimInstance
will return a PowerShell object for each storage volume found as shown below.
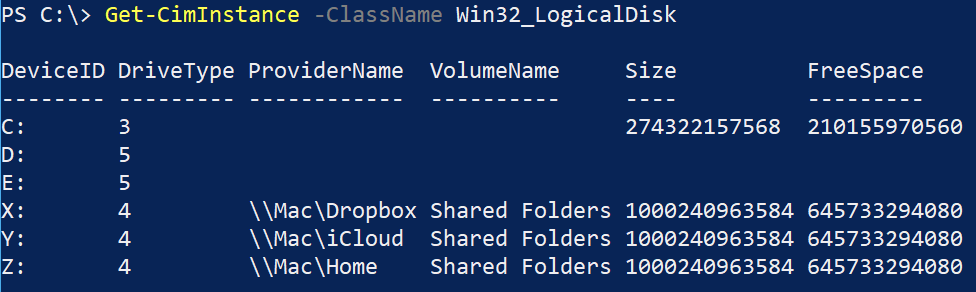
Get-CimInstance
Notice that Get-CimInstance
returns a FreeSpace
property attached to each storage volume object returned. The FreeSpace
property is what you need to work with.
Limiting CIM Output Disk Name and Free Space
Assuming you don’t need all of the information returned with Get-CimInstance
, you’ll now need to limit what to see. Suppose you’d like only to see the drive letter name (DeviceID
) and the amount of free space (FreeSpace
). To do that, you can trim down the output with Select-Object
.
Get-CimInstance -ClassName Win32_LogicalDisk | Select-Object -Property DeviceID,FreeSpace
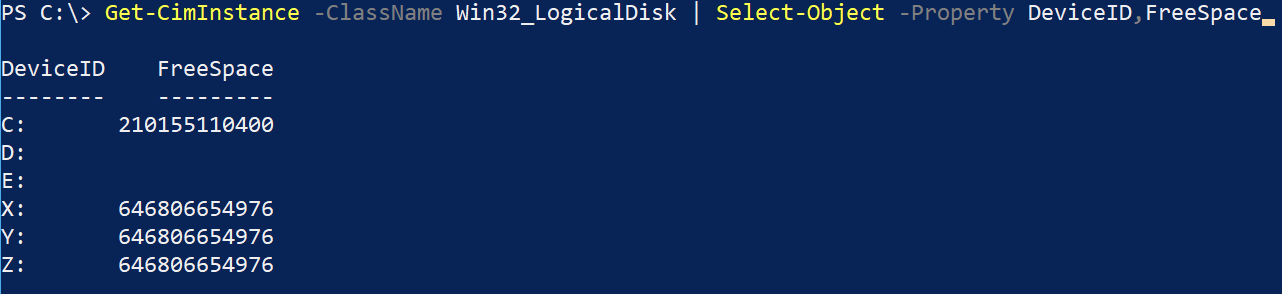
DeviceID
and FreeSpace
You now have a nice little report of free disk space.
Converting Disk Space from Bytes to Gigabytes
Although you now have a command to find free disk space, something looks off. You can probably tell that FreeSpace
is not defined in GB; it’s actually defined in bytes. Who needs that level of granularity, anyway? Let’s convert bytes to a more readable gigabytes.
To perform the conversion, you must first divide bytes by 1GB
. For example, in this instance, you know that the C:
disk contains 210155110400
bytes of free disk space. To convert bytes to gigabytes, you can divide that number by 1GB
like below.
210155110400 / 1GB
You’ll see that the C:
drive as a total of 195GB and some change.

You’re getting closer. Chances are you were looking for a nice round number but didn’t get it with that conversion. No sweat! You need to do one last thing, which is round the gigabytes to the closest integer.
One of the easiest ways to “round” decimals to the nearest integer is casting them to an integer like below. You’ll see that the number is rounded up. This method is quicker than using the Round()
method.
[int](210155110400 / 1GB)
Prettying up the Disk Space Output
Now that you know how to convert the free space bytes into gigabytes incorporate this technique into your script. One of the best ways to do that is with calculated properties. Calculated properties allow you to run code against property values to change them.
Calculated properties allow you to define a property value as a scriptblock which is essentially a portable unit of code.
The code you have so far only returns the disk label (DeviceID
) and free space (FreeSpace
) displayed in bytes like below.
Get-CimInstance -ClassName Win32_LogicalDisk | Select-Object -Property DeviceID,FreeSpace
Now build your own FreeSpace
property only this time, it’s represented in gigabytes.
Get-CimInstance -ClassName Win32_LogicalDisk | Select-Object -Property DeviceID,@{'Name' = 'FreeSpace (GB)'; Expression= { [int]($_.FreeSpace / 1GB) }}
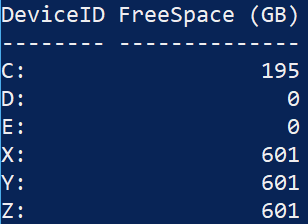
That’s much better! You now have exactly the information you were looking for.
Bonus: Grouping Free Space
In the example above, you built a command to return free space for each volume. Maybe you don’t care about each volume and would rather get a total free disk space from all volumes on the computer.
Maybe you’re building a script that will process many machines at once and you want output to look like this:
ServerName FreeSpace
---------- ---------
SRV1 195
SRV2 45
In that case, you need to group all of the rows returned in the previous example and sum up all of the FreeSpace
rows using the Measure-Object
cmdlet. This cmdlet takes an input of multiple objects, reads the property, and then performs some operation.
Get-CimInstance -ClassName Win32_LogicalDisk | Select-Object -Property DeviceID,@{'Name' = 'FreeSpace (GB)'; Expression= { [int]($_.FreeSpace / 1GB) }} | Measure-Object -Property 'FreeSpace (GB)' -Sum
You can now see that the Sum
property returned from Measure-Object
is 1998, which is the total amount of free space in gigabytes this computer has.
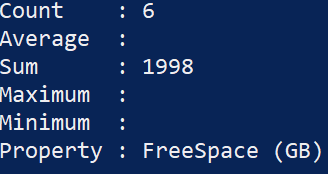
Finally, remove all of the unwanted properties and just return the total amount of free space.
(Get-CimInstance -ClassName Win32_LogicalDisk | Select-Object -Property DeviceID,@{'Name' = 'FreeSpace (GB)'; Expression= { [int]($_.FreeSpace / 1GB) }} | Measure-Object -Property 'FreeSpace (GB)' -Sum).Sum
Conclusion
Finding disk usage and disk free space with PowerShell only takes a single line, but you must first know how to create it! In this tutorial, you learned how to use PowerShell to get free disk space and format the output to your liking.
Improve upon this code. See if you can build a script to run this code over many servers at once!