When working with PowerShell, you’ll often encounter complex objects with deeply nested properties and relationships. While simple objects are easy to export to CSV files, preserving the structure of complex objects requires a different approach. Enter CliXML – PowerShell’s way of serializing and storing complex objects.
In this tutorial, you’ll learn how to export complex PowerShell objects to CliXML using a workout program management system as an example. You’ll see how to maintain object relationships, handle nested data, and ensure your exports are done safely.
Prerequisites
To follow along with this tutorial, you’ll need:
- PowerShell 5.1 or later (all examples use PowerShell 7)
- Basic understanding of PowerShell objects and properties
- A text editor to view XML files
Understanding Complex Objects in PowerShell
Let’s start with a real-world example – a workout program management system. We’ll use a function called Get-WorkoutProgram that returns workout data with nested trainers, routines, and exercise details.
function Get-WorkoutProgram {
param()
@(
[pscustomobject]@{
Name = "StrengthTraining"
Trainers = @(
[pscustomobject]@{
Name = "Alex";
Specialization = "Strength Conditioning";
ExperienceYears = 5;
Certifications = @("CPT", "CSCS")
},
[pscustomobject]@{
Name = "Jordan";
Specialization = "Functional Training";
ExperienceYears = 3;
Certifications = @("CPT", "FMS Level 1")
}
)
Routines = @(
[pscustomobject]@{
Day = "Monday";
Exercises = @(
[pscustomobject]@{
Name = "Squats";
Sets = 5;
Reps = 10;
Notes = "Focus on form and depth";
Details = @{
Equipment = "Barbell";
TechniqueTips = "Keep feet shoulder-width apart and drive through the heels."
}
},
[pscustomobject]@{
Name = "Bench Press";
Sets = 4;
Reps = 8;
Notes = "Maintain a slow and controlled motion";
Details = @{
Equipment = "Bench, Barbell";
TechniqueTips = "Align wrists and elbows, lower bar to mid-chest."
}
}
)
}
[pscustomobject]@{
Day = "Wednesday";
Exercises = @(
[pscustomobject]@{
Name = "Deadlift";
Sets = 5;
Reps = 5;
Notes = "Keep your back straight";
Details = @{
Equipment = "Barbell";
TechniqueTips = "Keep the bar close to your body, lift with your legs."
}
},
[pscustomobject]@{
Name = "Pull-ups";
Sets = 3;
Reps = "Max";
Notes = "Full range of motion";
Details = @{
Equipment = "Pull-up Bar";
TechniqueTips = "Avoid swinging, pull up until chin is above the bar."
}
}
)
}
)
}
[pscustomobject]@{
Name = "CardioBlast"
Trainers = @(
[pscustomobject]@{
Name = "Mia";
Specialization = "Cardio Training";
ExperienceYears = 4;
Certifications = @("ACE", "NASM")
}
)
Routines = @(
[pscustomobject]@{
Day = "Tuesday";
Exercises = @(
[pscustomobject]@{
Name = "Treadmill Sprint";
Duration = "30min";
Notes = "High-intensity interval training";
},
[pscustomobject]@{
Name = "Cycling";
Duration = "45min";
Notes = "Steady state cycling";
}
)
}
)
}
[pscustomobject]@{
Name = "YogaFlex"
Trainers = @(
[pscustomobject]@{
Name = "Ethan";
Specialization = "Yoga and Flexibility";
ExperienceYears = 6;
Certifications = @("RYT-200", "C-IAYT")
}
)
Routines = @(
[pscustomobject]@{
Day = "Friday";
Exercises = @(
[pscustomobject]@{
Name = "Vinyasa Flow";
Duration = "60min";
Notes = "Fluid movement and breath synchronization";
},
[pscustomobject]@{
Name = "Hatha Yoga";
Duration = "60min";
Notes = "Focus on alignment and mindfulness";
}
)
}
)
}
)
}
# Get our workout program data
$workoutData = Get-WorkoutProgram
Take a look at what this data contains:
$workoutData
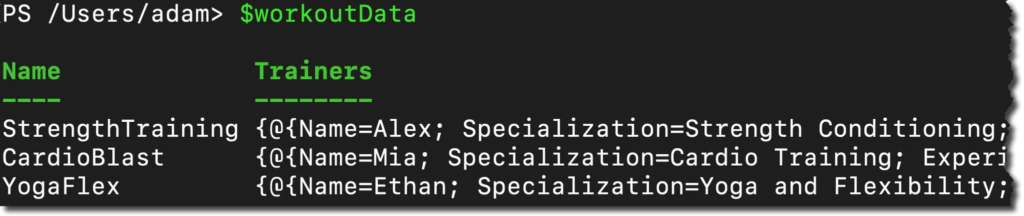
You’ll notice each program has properties like `Trainers` and `Routines` that contain nested objects (indicated by the `@` sign and curly braces). Let’s peek under the hood with `Get-Member`:
$workoutData | Get-Member
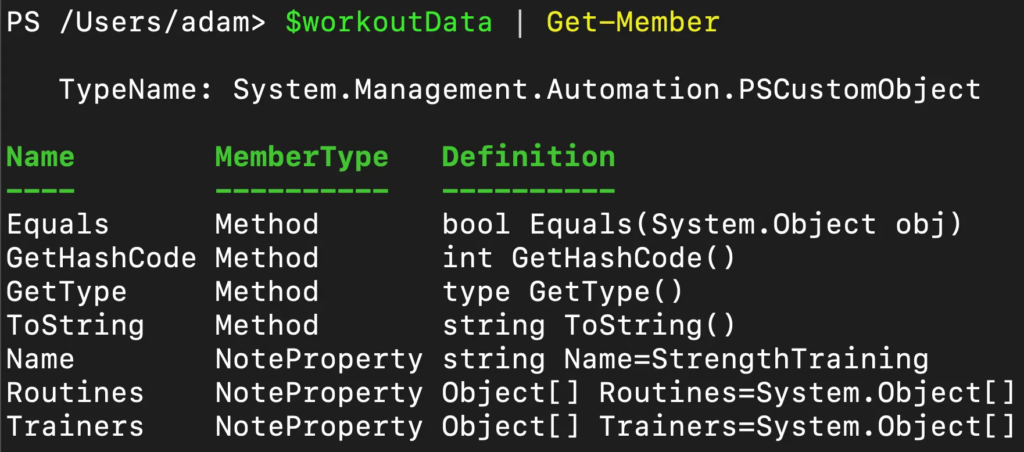
See those `Object[]` types for `Routines` and `Trainers`? That tells us these properties contain arrays of nested objects. Let’s look at the trainers for the first program:
$workoutData[0].Trainers

Now we can see each trainer has their own properties, including an array of certifications. This kind of nested structure is exactly why we need CliXML for export.
Exporting to CliXML
Exporting to CliXML is straightforward with the `Export-Clixml` cmdlet:
$workoutData | Export-Clixml -Path "WorkoutPrograms.xml"
Let’s peek at what PowerShell created:
Get-Content "WorkoutPrograms.xml"
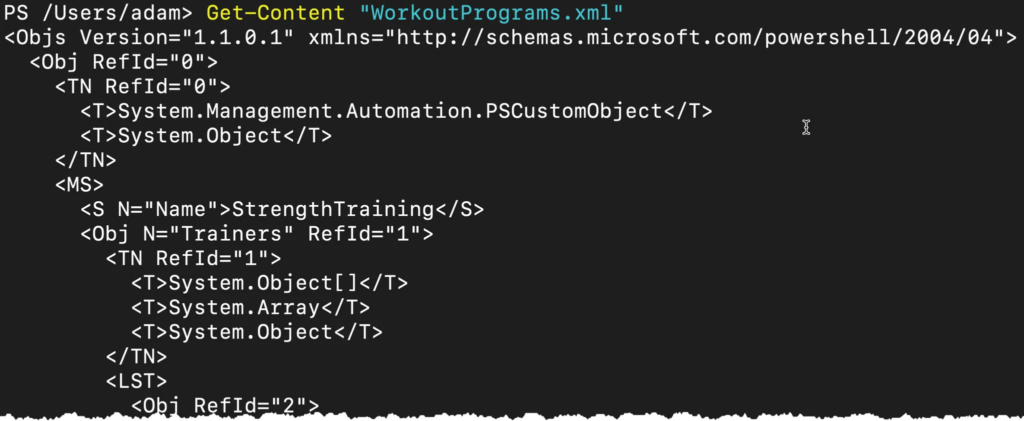
The output might look intimidating at first – it’s a structured XML format with tags like `
Protecting Your Data with NoClobber
What happens if you need to export updated data but want to protect your original export? The `-NoClobber` parameter prevents accidental overwrites:
$workoutData | Export-Clixml -Path "WorkoutPrograms.xml" -NoClobber
If the file exists, PowerShell will throw an error instead of overwriting it – much safer than the default behavior!
Best Practices for CliXML Exports
When working with CliXML exports, keep these tips in mind:
1. Use meaningful file names that indicate the data type and export date
2. Always use `NoClobber` when protecting existing exports is important
3. Remember that CliXML files are text-based and can be version controlled
4. Consider compressing large CliXML files if storage is a concern
When to Use CliXML vs Other Export Types
CliXML is perfect for:
- Complex objects with nested properties
- Data that needs to maintain type information
- PowerShell-specific data structures
- Temporary storage of live objects
But consider alternatives when:
- You need human-readable exports (use CSV)
- You’re sharing data with non-PowerShell systems
- You only have simple, flat data structures
Wrapping Up
CliXML is a powerful tool for preserving complex PowerShell objects. While the exported files might not be pretty to look at, they maintain all the rich structure and relationships of your original objects. Whether you’re working with workout programs like our example or any other complex data structure, CliXML has you covered.
Next time you need to export complex PowerShell objects, remember:
1. Check your object structure with `Get-Member`
2. Use `Export-Clixml` to preserve all object relationships
3. Protect existing files with `NoClobber`
4. Choose CliXML when object complexity matters
With these tools in your PowerShell toolbelt, you’re ready to handle even the most complex data export scenarios.