Do you need to find a local or remote Windows computer’s name in a PowerShell script? Look no further. In this tutorial, you’re going to learn how to use PowerShell to get computer name in a few different and sometimes unexpected ways.
Not a reader? Watch this related video tutorial!
Not seeing the video? Make sure your ad blocker is disabled.
Prerequisites
This article will be a hands-on tutorial. If you’d like to apply the concepts you learn in this tutorial, please be sure you have PowerShell. That’s it! The article will be using PowerShell 7 (the latest as of this writing) but Windows PowerShell will probably work just as well.
Using the Hostname
command in PowerShell to Get Computer Name
Back before the days of PowerShell, the only Windows command interpreter we had was good ol’ cmd .exe. Back then, we didn’t need no stinkin’ PowerShell to get a computer name; we had the hostname
command!
The hostname
command couldn’t be any simpler. Open up a PowerShell (or even cmd .exe prompt) and type hostname
. Done. This command returns a single string (the computer name of the local computer).
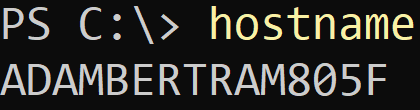
Using the System.Net.DNS
.NET Class
If you’d like to go the more PowerShell-centric approach, you can also reference a static method called GetHostByName()
located in the System.Net.DNS
.NET class or perhaps the GetHostName()
method.
The GetHostName()
Method
Using the GetHostName()
method is probably the easiest way to use PowerShell to get a computer name. Simply call this static method with no arguments as shown below. This command will return a single string just like the hostname
command does.
[System.Net.Dns]::GetHostName()
The GetHostByName()
Method
An alternative but similar System.Net.DNS
class method you can use to get a computer name is called GetHostByName()
. This method is actually a DNS resolver and can be used to look up other host names as well.
If, for example, you’d like to find the host name of the local computer, run [System.NET.DNS]::GetHostByName($null)
or [System.NET.DNS]::GetHostByName('')
.
[System.Net.DNS]::GetHostByName('')
[System.Net.DNS]::GetHostByName($Null)
You’ll see that this method isn’t strictly meant for finding computer names; you can also lookup IP addresses as well via the AddressList
property as shown below.
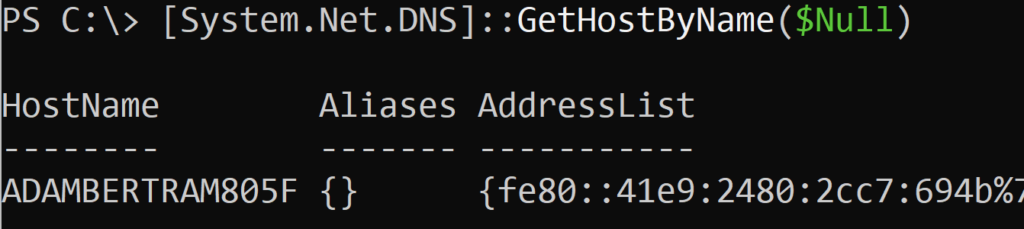
If, however, you want to only find the local computer name; reference the HostName
property, and PowerShell will only return the hostname.
[System.Net.DNS]::GetHostByName('').HostName
[System.Net.DNS]::GetHostByName($Null).HostName
Using Environment Variables
Environment variables are always a great place to find information about Windows computers; using PowerShell to get a computer name is no different.
The ComputerName Environment Variable
Every Windows computer stores an environment variable called ComputerName
. Like all other environment variables, you can access user environment variables via the $env
PowerShell construct.
To reference the COMPUTERNAME
environment variable, open up PowerShell and preface the environment variable name with $env:
. PowerShell will then return the local computer name as a single string.
$env:COMPUTERNAME
The MachineName Property
Alternatively, if, for some reason, the user-based COMPUTERNAME
environment variable doesn’t work in your situation, you can also use the MachineName
property that’s part of the .NET Environment
class.
Reference the MachineName
property in the Environment
.NET class as shown below.
[Environment]::MachineName
Using WMI
Finally, you’ve always got the option of going into WMI or CIM. You probably should make this your last resort as it will require the most overhead albeit tiny. Using PowerShell to get a computer name with WMI would be best to query remote computer names.
If you’d like to use WMI to query a local computer name, use Get-CimInstance
to query the Win32_ComputerSystem class as shown below. Since Get-CimInstance
doesn’t return the computer name but an object representing a CIM instance, reference the Name
property to only return the computer name.
Get-CimInstance -ClassName Win32_ComputerSystem
(Get-CimInstance -ClassName Win32_ComputerSystem).Name
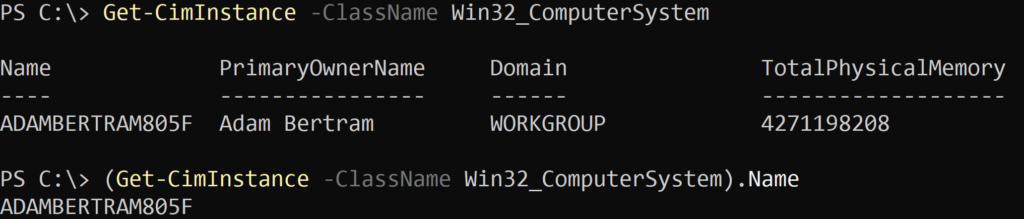
Finding Remote Computer Names
Perhaps you manage many computers and need to find the hostname across all of them. Typically, the hostname will be represented in Active Directory (AD) if you’re in that kind of environment or collected by some other asset management tool. But if it’s not, you can always fall back to PowerShell.
To use PowerShell to get remote computer names, you have two options. You can either wrap the methods you learned above in a PowerShell Remoting scriptblock or use WMI.
Using PowerShell Remoting
Rather than cover each method cover earlier again in this section, just know this. You can wrap any local command in a PowerShell Remoting scriptblock.
If, for example, you have PowerShell Remoting enabled on a remote computer, you can put any of the above methods inside of a scriptblock and execute that scriptblock with the Invoke-Command
command.
Assuming you only have an IP address and need to find the hostname of a computer with the IP address of 192.168.1.2 and you’re not in an AD environment, call Invoke-Command
like below.
## Create the pscredential object to pass to Invoke-Command
$credential = Get-Credential
## Run the command on the remote computer
Invoke-Command -ComputerName 192.168.1.2 -ScriptBlock { [System.Net.Dns]::GetHostName() } -Credential $credential
If PowerShell Remoting was able to connect to the remote computer, PowerShell will return the same output as you’d see if you were running this command locally.
Using WMI
Alternatively, you can also use WMI to use PowerShell to get a computer name without having to wrap a command inside of a scriptblock. The process to find a remote computer name is nearly the same as locally; simply use the ComputerName
parameter.
Get-CimInstance -ClassName Win32_ComputerSystem -ComputerName 192.168.1.2
(Get-CimInstance -ClassName Win32_ComputerSystem -ComputerName 192.168.1.2).Name
The Get-CimInstance
requires authentication. If you’re in an AD environment, you will not need to supply any credentials as seen above. However, if you’re not in an AD environment, you’ll need to establish a new CIM session with the New-CimSession
cmdlet, use Get-CimInstance
to use that session, and then remove the CIM session yourself. Below is a code snippet on how to do that.
## Create the PSCredential object
$credential = Get-Credential
## Connect to the remote computer passing the creds and creating a remote session
$cimSession = New-CimSession -ComputerName 192.168.1.5 -Credential $credential
## Use the session to query WMI and reference the Name property
(Get-CimInstance -Session $cimSession -ClassName Win32_ComputerSystem).Name
Next Steps
If you’ve read through this entire tutorial, you should now have nearly all of the most popular ways to use PowerShell to get a computer name. Although there are probably many more we’ve missed, these are all of the ways you’ll find this task accomplished in many scripts.
Now that you know how to accomplish this task, try to build a script that will get computer names in bulk with a loop perhaps via a CSV or text file!