Computer resources depletion is a common call driver to IT. Issues such as processes that consume several GBs of RAM would typically reach the end-user support lines and often involve manual fixing. How can 1E Tachyon help automate the monitoring and fixing of such problems?
Tachyon has a PowerShell module called PSTachyonToolkit
. This module opens up opportunities to develop PowerShell automation scripts with Tachyon. And this article aims to demonstrate how you can use the PowerShell and Tachyon integration to solve a specific use-case scenario.
Killing Processes: The PowerShell Way
As administrators, you could quickly whip up a PowerShell script to fix endpoint issues such as killing processes that breached a specific memory usage threshold. For example, the script below checks the processes on remote computers using 1.5GB or more memory and terminates them.
# Kill-MemoryHog.ps1
## Array of target endpoints
$computers = @('pc0003', 'pc0006')
## Set the RAM usage threshold
$threshold = 1.5GB
Invoke-Command `
-ComputerName $computers `
-ScriptBlock {
## Get and kill the process(es)
Get-Process | Where-Object { $_.WS -ge $Using:threshold } | Stop-Process -Force -Confirm:$false
}
If you’ve worked with PowerShell remoting, you’re probably shaking your head already because you know the pitfalls of running such scripts.
- The script alone does not check whether the target endpoint is online. Running any command against offline remote computers will significantly impact the duration and performance of the script.
- WinRM must be properly configured and running on the endpoints, or the remoting will fail.
- You’ll have to update your target endpoints list manually
- You may need to use a service account with local administrator permissions on all endpoints. Otherwise, your script will end up with an access denied error.
- Firewall exceptions.
Killing Processes: PowerShell and Tachyon, Better Together
Instead of running PowerShell commands remotely to kill rogue processes, you can use existing Tachyon instructions or combine them with new ones to perform the same task — but better. How?
First, if a user’s PC is online, you know that someone is using that PC. And ideally, your workflow should involve, at the least, notifying users that an automated task will terminate an impacting process.
Second, running Tachyon instructions eliminates the need for PowerShell remoting. So long as the endpoint has the 1E client, you no longer have to deal with the remoting requirements.
Third, you already have an inventory of all endpoints. You’ll only need to filter which endpoints your automation should target.
Lastly, you’ll have a record or run history of the task in Tachyon, which you may view in the Tachyon Explorer.
All of what’s listed above, Tachyon can provide.
With those in mind, the solution should be capable of doing the following:
- Get a list of target endpoints that are currently online.
- List each endpoint’s running processes that breached a specific amount of memory usage.
- Send a notification to each affected endpoint about the automatic termination of these processes.
- Kill all offending processes on each affected endpoint.
Building the Target Endpoint List
Unless you plan to run an instruction against every available endpoint device in your environment, which is generally a bad idea, you’ll want to build a target list. How you select the endpoints is entirely up to you.
The below code gets the list of all online Desktop
devices and saves it into a variable.
# Import the PSTachyonToolkit module
Import-Module 'C:\Program Files\1E\Tachyon\pstoolkit\PSTachyonToolkit.psd1'
# Connect to the Tachyon Server
Set-TachyonServer 1E01.corp.1EDemoLab.com
# Set the instruction prefix that matches your license.
Set-TachyonInstructionPrefix "1E-Demo"
# Get all desktop endpoints that are online.
$targetEndpoints = @((Get-TachyonDevice -Full | Where-Object { $_.DeviceType -eq 'Desktop' -and $_.Status -eq 1 }).FQDN)
As you can see below, the command pulled six Tachyon endpoint devices, which will be the list of computers we’ll target to query information from.

Retrieving the Process List and Filtering the Results
Now that the target FQDN list is available, next is to invoke a Tachyon instruction to list each endpoint’s running processes. The Tachyon instruction name, in this case, is 1E-Exchange-ProcessDetails
.
# Invoke the instruction to get the running processes details on each endpoint.
$instructionID = Invoke-TachyonInstruction -instruction '1E-Exchange-ProcessDetails' -TargetFqdns $targetEndpoints -NoWait
After invoking the instruction, retrieve the results by running the Get-TachyonInstructionResult
and specifying the instruction ID. But instead of getting all results, the command below will return only the processes that use 1.5GB memory or more.
# Set the RAM usage threshold
$memThreshold = 1.5GB
# Retrieve the result
$result = Get-TachyonInstructionResult -Id $instructionID -Resultfilter "MemoryUsageBytes>=$($memThreshold)"
By default, the result is in a Hashtable object, as you can see below.

Using some PowerShell magic, convert the Hastable result into a PSCustom object for easier reference.
# Merge Fqdn and values
$result.Keys | ForEach-Object {
$result[$_] | Add-Member -MemberType NoteProperty -Name Fqdn -Value $_ -Force
}
# Convert result from Hashtable to custom object
$result = $result.Values.ToArray() | Sort-Object Fqdn | Select-Object Fqdn, Executable, ProcessId, ParentExecutable, MemoryUsageBytes, AccountName
The result is now clearer, being an array rather than a hashtable. As you can see on the screenshot below, the Tachyon instruction reported two devices with processes consuming over 1.5GB of RAM.
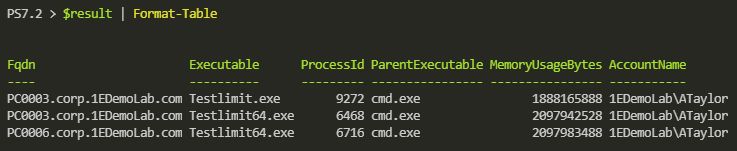
Notifying Users and Killing the Processes
The next step is to notify the users about the offending processes and automatically kill them. But first, make sure that your target FQDN list is unique. You would not want to invoke the same instruction more than once to the same endpoint.
# Get a unique list of FQDN.
$fqdns = @(($result | Select-Object -Unique Fqdn).Fqdn)
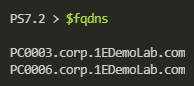
Loop through each device FQDN and invoke the 1E-Demo-SendNotification
and 1E-Demo-TerminateProcessList
instructions to notify the users and kill processes, respectively.
The code in this section uses two custom Tachyon instructions, namely
1E-Demo-SendNotification
for sending notifications to users, and1E-Demo-TerminateProcessList
kills the processes on the target machines.These XML files for these two instructions were signed using the Tachyon Instruction Management Studio (TIMS) and uploaded to Tachyon. You can download the unsigned version of these files using the links below should you want to use these instructions.
1E-Demo-SendNotification.xml, 1E-Demo-TerminateProcessList.xml
# Loop through each FQDN and issue the notify and kill instructions
for ($i = 0; $i -lt $fqdns.Count; $i++) {
# Get all the processes to terminate on the current endpoint
$process = $result | Where-Object { $_.Fqdn -eq $fqdns[$i] }
# Notify Users
$notificationSplat = @{
TargetFqdns = $fqdns[$i]
Instruction = '1E-Demo-SendNotification' # Must publish the 1E-Demo-SendNotification.xml instruction first
NoWait = $true
InstTTL = 10
Title = 'Kill Process Notification'
Message = "The follow processes are consuming over $("{0:N0}" -f $memThreshold) bytes of memory each and may be affecting your system's performance. " +
"Your IT department will now attempt to close these processes and there are no actions required from you at this time." +
"`n`nProcess list: $(($process.Executable) -Join ',')"
}
$null = Invoke-TachyonInstruction @notificationSplat
# Kill Processes
$killProcessSplat = @{
TargetFqdns = $fqdns[$i]
Instruction = '1E-Demo-TerminateProcessList' # Must publish the 1E-Demo-TerminateProcessList.xml instruction first
NoWait = $true
InstTTL = 10
ProcessIdList = $(($process.ProcessId) -Join ',')
}
$null = Invoke-TachyonInstruction @killProcessSplat
}
This PowerShell script is available to download at this link:
Kill-TachyonRougeProcess.ps1
.
Once you run the code, the end-user will receive the notification below.
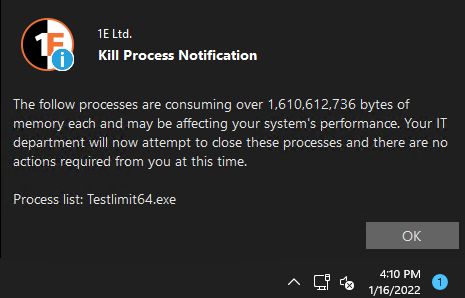
That’s it! You have created a reusable PowerShell script that uses that PSTachyonToolkit to invoke instructions, process results, and perform follow-up actions.
You can now schedule this script to run at an interval using your preferred tool. Perhaps in Jenkins, Azure Automation, or Task Scheduler?
My Conclusion
System admins and developers alike have been using PowerShell for automation since its inception, and rightfully so. But using PowerShell alone is not always the best solution.
Tachyon’s PowerShell integration can help elevate how IT deals with seemingly mundane yet ubiquitous tasks. With Tachyon, you can focus on creating solutions and improving productivity while skipping the burden that comes with PowerShell remoting.