“Hello IT, my website is not loading.” — Sound familiar? It sure does if you’ve worked with desktop or end-user support. And is most cases, flushing the DNS cache on the user’s computer can help. Granted that there are many possible reasons for this issue, but you have to start somewhere.
Why not automate the monitoring of URL response times and flush the DNS cache on remote machines. As a result, you can potentially reduce or prevent service desk calls. Yes, you can do so with the Tachyon and PowerShell.
This article will show you how you can use 1E’s Tachyon platform and its PSTachyonToolkit
module integration to solve this example use-case scenario.
Fixing Endpoint Issues with PowerShell Remoting
Having used PowerShell for automation for most of my IT career, my instinct would be to create a script or function for this purpose. For example, the code below is for a reusable script named PSFlushDNS.ps1
.
This script will perform a URL query on remote computers. If the total duration exceeds a specified threshold in milliseconds, the script will execute the ipconfig /flushdns
command to flush the remote computer’s DNS cache.
# PSFlushDNSCache.ps1
[CmdletBinding()]
param(
# List of target computers
[Parameter(Mandatory)]
[string[]]
$TargetComputer,
# URL for testing (ie. yahoo.com, https://www.ietf.org/, http://powershell.org)
[Parameter(Mandatory)]
[string]
$URL,
# Duration threshold in milliseconds
[Parameter(Mandatory)]
[int]
$Threshold
)
$TargetComputer | ForEach-Object {
$_ | Out-Default
Invoke-Command -ComputerName $_ -ScriptBlock {
# Set the URL string
$URL = $using:URL
# Set the response time threshold in milliseconds
$Threshold = $using:ResponseThresholdMS
# If the URl does not begin with 'http', insert the 'http://' string prfix.
if ($URL.Substring(0, 4) -notcontains 'http') {
$URL = "http://$($URL)"
}
# Download the website content and return the duration in milliseconds.
$wc = New-Object System.Net.WebClient
$result = Measure-Command { $wc.DownloadString($URL) }
# Display duration
$result.Milliseconds | Out-Default
# Check if the total duration is over the threshold.
# If so, flush the DNS cache.
if ($result.Milliseconds -gt $Threshold) {
cmd /c 'ipconfig /flushdns'
}
}
}
Running the script using the command below will target two computers namely PC0003
and PC0006
.
.\PsFlushDnsCache.ps1 `
-TargetComputer @('PC0003','PC0006') `
-URL yahoo.com `
-Threshold 400
The result below shows that the script successfully flushed the DNS cache for PC0006
, which took 635
milliseconds to query yahoo.com
.
But PC0003
failed due to a WinRM-related error. The WinRM service is probably not running, not configured, or perhaps the remote computer is offline. Whatever the reason, the bottom line is this error will require more troubleshooting. What if you need to run the same script on hundreds of PC?
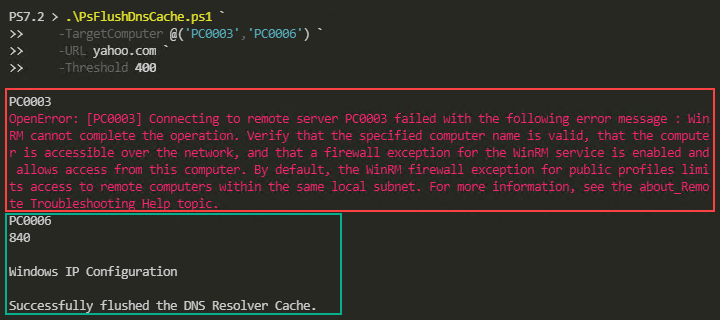
This script is basic and can be improved to include checking for device connectivity, adding error handling, and so on. But even then, the script will still be dependent on PowerShell remoting and WinRM.
Saving Time and Increasing Efficiency with 1E Tachyon and PowerShell
Doing away with PowerShell remoting, you could instead use PowerShell to integrate with Tachyon to run instructions. You can always take advantage of existing instructions or combine them with new custom instructions as you see fit.
But in this example, I’ll stick to running PowerShell scripts as dynamic instructions. This way, I do not have to publish the Tachyon instruction beforehand, and the PowerShell script will run as though I’m executing them locally on the remote machine.
The PowerShell Script
The code below is a modified version of the previous script, removing the remoting logic. Also, this script accepts two parameters only — URL
and Threshold
. You’ll also notice that the final line returns the output in JSON format, which is important for proper serialization with Tachyon.
# TachyonFlushDNSCache.ps1
[CmdletBinding()]
param(
# URL for testing (ie. yahoo.com, https://www.ietf.org/, http://powershell.org)
[Parameter(Mandatory)]
[string]
$URL,
# Duration threshold in milliseconds
[Parameter(Mandatory)]
[int]
$Threshold
)
# If the URl does not begin with 'http', insert the 'http://' string prfix to make a proper URL string.
if ($URL.Substring(0, 4) -notcontains 'http') {
$URL = "http://$($URL)"
}
# Download the website content and measure the response duration
$wc = New-Object System.Net.WebClient
$duration = Measure-Command { $wc.DownloadString($URL) }
# Create a custom object to hold the result.
$output = '' | Select-Object ResponseTime, Result
$output.ResponseTime = $duration.TotalMilliseconds
$output.Result = "No action. Duration < ($($Threshold)ms)."
if ($duration.TotalMilliseconds -gt $Threshold) {
$output.Result = [string](cmd /c 'ipconfig /flushdns')
}
# Return output in JSON format
$output | ConvertTo-Json
Running the PowerShell Dynamic Instruction
With Tachyon, you already have an inventory of target devices, and you only need to filter based on your requirements. In this example, I’ll be targeting devices based on two locations; London and New York.
But first, I’ll import the PSTachyonToolkit
module, connect to the Tachyon server, and set the instruction prefix that matches the Tachyon license.
# Import the PSTahcyonToolkit module.
Import-Module 'C:\Program Files\1E\Tachyon\pstoolkit\PSTachyonToolkit.psd1'
# Connect to the Tachyon server.
Set-TachyonServer 1E01.corp.1EDemoLab.com
# Set the licensed dynamic instruction prefix.
Set-TachyonInstructionPrefix '1E-Demo'
Next, I’ll run the command below that targets the devices in the New York and London locations using the Invoke-TachyonDynamic
cmdlet. To understand the command better, here are the parameters:
- The
-Script
parameter specifies the PowerShell script to run on the remote computers.
- The
-TargetScope
parameter accepts the filter expression. If a filter value contains spaces, make sure to enclose the value in square brackets. In this example, the filter islocation=[New York]
, which means that Tachyon will run the instruction against the devices in New York only.
- The
-Parameters
parameter accepts the parameter(s) to pass to the script. The values must follow the same order in the script as an array. For example, the script accepts theURL
andThreshold
parameters, so the sample values are@('yahoo.com',400)
.
- The
-Schema
parameter is optional. I’m using this parameter to control the instruction output and filter which properties to return.
- The
-Force
switch overrides the target machine’s script execution policy.
# Run the script against the devices on location 1 (New York)
Invoke-TachyonDynamic `
-Script .\TachyonFlushDNSCache.ps1 `
-Parameters @('yahoo.com',400) `
-TargetScope "location=[New York]" `
-Schema 'ResponseTime double,Result string' `
-Force
# Run the script against the devices on location 2 (London)
Invoke-TachyonDynamic `
-Script .\TachyonFlushDNSCache.ps1 `
-Parameters @('yahoo.com',400) `
-TargetScope "location=London" `
-Schema 'ResponseTime double,Result string' `
-Force
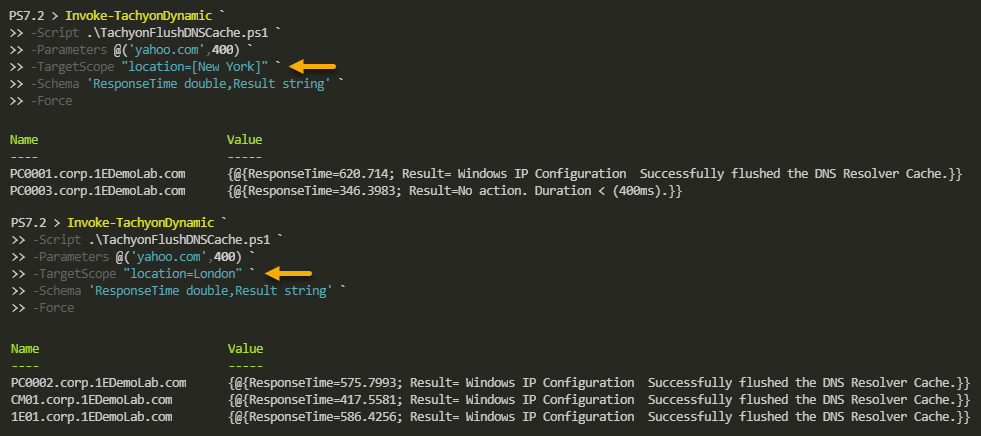
Alternatively, I can combine the TargetScope
filter expression to include both locations at once instead of running the instructions targeting different locations individually.
# Run the script against the devices on New York and London endpoint devices
Invoke-TachyonDynamic `
-Script .\TachyonFlushDNSCache.ps1 `
-Parameters @('yahoo.com',400) `
-TargetScope "location=[New York] or location=London" `
-Schema 'ResponseTime double,Result string' `
-Force
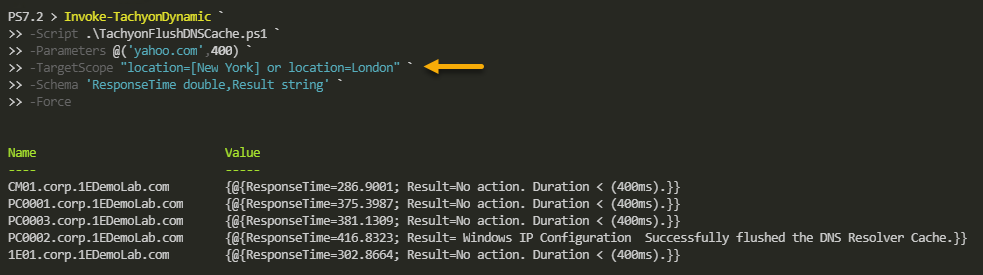
There it is! Take this script, modify it to your needs, and perhaps learn more about how you can work with Tachyon in PowerShell.
My Conclusion
With Tachyon and its PowerShell integration, you don’t need to become a Tachyon expert if you’re more comfortable with coding PowerShell. You can keep working with PowerShell and incorporate Tachyon’s features and power to make your work more efficient.
If you’re open to exploring Tachyon more, you may find that there could already be instructions that you can use for your specific use-cases. Take a look at the Tachyon Exchange and its 84 product packs, 799 instructions, and its 18 policies. Wouldn’t those be a time saver?